Analyze microhh LES data
In this notebook, we will analyze data from the microhh model, simulating a case near the Southern Great Plains.
Imports
#Load the usual libraries
import matplotlib.pyplot as plt
import xarray as xr
import scipy as sp
import numpy as np
import netCDF4 as nc
Find the Data
# locate the folder where the data is stored
basedir = '/data/project/ARM_Summer_School_2024_Data/summerschool_microhh/'
basename = 'SGP'
NC_arr = [10, 50, 80, 100, 200, 400, 800]
Configure some helper functions
# Load a specific data set, and cycling through all groups to load all data
def load_data(fname):
groups = [''] + list(nc.Dataset(fname).groups.keys())
prof_dict = {}
for group in groups:
try:
prof_dict[group] = xr.open_dataset(fname, group=group, decode_times=True)
except KeyError:
print(f'Group {group} not found in {fname}')
except IOError:
print(f'File {fname} not found')
except:
print('Unexpected error')
prof = xr.merge(prof_dict.values())
prof = prof.where(prof.apply(np.fabs)<1e10)
return prof
# Cycle through all conditional samples and load the data; the conditional sample is added as a new dimension
def load_cond_data(dir, basename, cond_samps):
prof_dict = {}
for cond_samp in cond_samps:
fname = dir + '/' + basename +'.' + cond_samp + '.0000000.nc'
prof_dict[cond_samp] = load_data(fname).expand_dims(dim={'sample': [cond_samp]})
prof = xr.merge(prof_dict.values())
return prof
# Cycle through all different Number concentrations and load the data; the number concentration is added as a new dimension
def load_all_data(basedir, fname, NCs, cond_samps = ['default']):
prof_array = {}
for NC in NCs:
NCstr = f'NC{NC:03}'
print('Loading data for ' + NCstr)
dir = basedir + '/' + NCstr + '/'
prof_array[NCstr] = load_cond_data(dir, basename, cond_samps).expand_dims(dim={'NC': [NC]})
return xr.merge(prof_array.values())
Run through the Analysis
# Load the data for all number concentrations and conditional samples
cond_samps = ['default', 'ql', 'qlcore']
prof = load_all_data(basedir, basename, NC_arr, cond_samps)
#Slice the data for our area of interest, and resample to 30 min intervals
prof = prof.sel(time=slice("2016-06-11T12", "2016-06-12T02"),z=slice(0,4000), zh=slice(0,4000)).resample(time='0.5H').mean(dim='time')
Loading data for NC010
Loading data for NC050
Loading data for NC080
Loading data for NC100
Loading data for NC200
Loading data for NC400
Loading data for NC800
#Explore the data set.
prof
<xarray.Dataset> Dimensions: (z: 107, zh: 108, zs: 4, zsh: 5, sample: 3, NC: 7, time: 30, lev: 141) Coordinates: * z (z) float32 10.0 21.0 33.0 ... 3.94e+03 3.98e+03 * zh (zh) float32 0.0 15.5 27.0 ... 3.92e+03 3.96e+03 4e+03 * zs (zs) float32 -1.89 -0.72 -0.21 -0.07 * zsh (zsh) float32 -2.62 -1.16 -0.28 -0.14 0.0 * sample (sample) object 'default' 'ql' 'qlcore' * NC (NC) int64 10 50 80 100 200 400 800 * time (time) datetime64[ns] 2016-06-11T12:00:00 ... 2016-... Dimensions without coordinates: lev Data variables: (12/141) p_rad (time, NC, sample, lev) float32 9.645e+04 ... 3.53 iter (time, NC, sample) float64 1.738e+05 ... 2.484e+05 area (time, NC, sample, z) float32 1.0 1.0 1.0 ... 0.0 0.0 areah (time, NC, sample, zh) float32 1.0 1.0 1.0 ... 0.0 0.0 u (time, NC, sample, z) float32 -0.09499 ... nan u_3 (time, NC, sample, z) float32 -0.001409 ... nan ... ... sw_flux_dn_ref (time, NC, sample, lev) float32 132.0 135.4 ... 0.6207 sw_flux_dn_dir_ref (time, NC, sample, lev) float32 105.2 109.1 ... 0.6207 lw_flux_up_ref (time, NC, sample, lev) float32 476.5 465.4 ... 282.1 lw_flux_dn_ref (time, NC, sample, lev) float32 386.1 359.6 ... 0.0 sza (time, NC, sample) float32 1.39 1.39 ... 1.743 1.743 sw_flux_dn_toa (time, NC, sample) float32 236.5 236.5 ... 0.6207
xarray.Dataset
- z: 107
- zh: 108
- zs: 4
- zsh: 5
- sample: 3
- NC: 7
- time: 30
- lev: 141
- z(z)float3210.0 21.0 ... 3.94e+03 3.98e+03
- units :
- m
- long_name :
- Full level height
array([ 10., 21., 33., 46., 60., 75., 92., 111., 132., 155., 180., 207., 237., 267., 300., 340., 380., 420., 460., 500., 540., 580., 620., 660., 700., 740., 780., 820., 860., 900., 940., 980., 1020., 1060., 1100., 1140., 1180., 1220., 1260., 1300., 1340., 1380., 1420., 1460., 1500., 1540., 1580., 1620., 1660., 1700., 1740., 1780., 1820., 1860., 1900., 1940., 1980., 2020., 2060., 2100., 2140., 2180., 2220., 2260., 2300., 2340., 2380., 2420., 2460., 2500., 2540., 2580., 2620., 2660., 2700., 2740., 2780., 2820., 2860., 2900., 2940., 2980., 3020., 3060., 3100., 3140., 3180., 3220., 3260., 3300., 3340., 3380., 3420., 3460., 3500., 3540., 3580., 3620., 3660., 3700., 3740., 3780., 3820., 3860., 3900., 3940., 3980.], dtype=float32)
- zh(zh)float320.0 15.5 27.0 ... 3.96e+03 4e+03
- units :
- m
- long_name :
- Half level height
array([ 0. , 15.5, 27. , 39.5, 53. , 67.5, 83.5, 101.5, 121.5, 143.5, 167.5, 193.5, 222. , 252. , 283.5, 320. , 360. , 400. , 440. , 480. , 520. , 560. , 600. , 640. , 680. , 720. , 760. , 800. , 840. , 880. , 920. , 960. , 1000. , 1040. , 1080. , 1120. , 1160. , 1200. , 1240. , 1280. , 1320. , 1360. , 1400. , 1440. , 1480. , 1520. , 1560. , 1600. , 1640. , 1680. , 1720. , 1760. , 1800. , 1840. , 1880. , 1920. , 1960. , 2000. , 2040. , 2080. , 2120. , 2160. , 2200. , 2240. , 2280. , 2320. , 2360. , 2400. , 2440. , 2480. , 2520. , 2560. , 2600. , 2640. , 2680. , 2720. , 2760. , 2800. , 2840. , 2880. , 2920. , 2960. , 3000. , 3040. , 3080. , 3120. , 3160. , 3200. , 3240. , 3280. , 3320. , 3360. , 3400. , 3440. , 3480. , 3520. , 3560. , 3600. , 3640. , 3680. , 3720. , 3760. , 3800. , 3840. , 3880. , 3920. , 3960. , 4000. ], dtype=float32)
- zs(zs)float32-1.89 -0.72 -0.21 -0.07
- units :
- m
- long_name :
- Full level height soil
array([-1.89, -0.72, -0.21, -0.07], dtype=float32)
- zsh(zsh)float32-2.62 -1.16 -0.28 -0.14 0.0
- units :
- m
- long_name :
- Half level height soil
array([-2.62, -1.16, -0.28, -0.14, 0. ], dtype=float32)
- sample(sample)object'default' 'ql' 'qlcore'
array(['default', 'ql', 'qlcore'], dtype=object)
- NC(NC)int6410 50 80 100 200 400 800
array([ 10, 50, 80, 100, 200, 400, 800])
- time(time)datetime64[ns]2016-06-11T12:00:00 ... 2016-06-...
array(['2016-06-11T12:00:00.000000000', '2016-06-11T12:30:00.000000000', '2016-06-11T13:00:00.000000000', '2016-06-11T13:30:00.000000000', '2016-06-11T14:00:00.000000000', '2016-06-11T14:30:00.000000000', '2016-06-11T15:00:00.000000000', '2016-06-11T15:30:00.000000000', '2016-06-11T16:00:00.000000000', '2016-06-11T16:30:00.000000000', '2016-06-11T17:00:00.000000000', '2016-06-11T17:30:00.000000000', '2016-06-11T18:00:00.000000000', '2016-06-11T18:30:00.000000000', '2016-06-11T19:00:00.000000000', '2016-06-11T19:30:00.000000000', '2016-06-11T20:00:00.000000000', '2016-06-11T20:30:00.000000000', '2016-06-11T21:00:00.000000000', '2016-06-11T21:30:00.000000000', '2016-06-11T22:00:00.000000000', '2016-06-11T22:30:00.000000000', '2016-06-11T23:00:00.000000000', '2016-06-11T23:30:00.000000000', '2016-06-12T00:00:00.000000000', '2016-06-12T00:30:00.000000000', '2016-06-12T01:00:00.000000000', '2016-06-12T01:30:00.000000000', '2016-06-12T02:00:00.000000000', '2016-06-12T02:30:00.000000000'], dtype='datetime64[ns]')
- p_rad(time, NC, sample, lev)float329.645e+04 9.188e+04 ... 3.871 3.53
- units :
- Pa
- long_name :
- Pressure of radiation reference column
array([[[[9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00]], [[9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00]], [[9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00]], ... [[9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00]], [[9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00]], [[9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00]]]], dtype=float32)
- iter(time, NC, sample)float641.738e+05 1.738e+05 ... 2.484e+05
- units :
- -
- long_name :
- Iteration number
array([[[173824.83333333, 173824.83333333, 173824.83333333], [173710. , 173710. , 173710. ], [171538. , 171538. , 171538. ], [171538. , 171538. , 171538. ], [173173.5 , 173173.5 , 173173.5 ], [173498.16666667, 173498.16666667, 173498.16666667], [171815.16666667, 171815.16666667, 171815.16666667]], [[176302.16666667, 176302.16666667, 176302.16666667], [176024.66666667, 176024.66666667, 176024.66666667], [173800.5 , 173800.5 , 173800.5 ], [173800.5 , 173800.5 , 173800.5 ], [175536. , 175536. , 175536. ], [175847.66666667, 175847.66666667, 175847.66666667], [174150. , 174150. , 174150. ]], [[178840.33333333, 178840.33333333, 178840.33333333], [178431.16666667, 178431.16666667, 178431.16666667], [176048.66666667, 176048.66666667, 176048.66666667], [176048.66666667, 176048.66666667, 176048.66666667], ... [244279.66666667, 244279.66666667, 244279.66666667], [247222.5 , 247222.5 , 247222.5 ], [247072.5 , 247072.5 , 247072.5 ], [245470.83333333, 245470.83333333, 245470.83333333]], [[249770.5 , 249770.5 , 249770.5 ], [249189.16666667, 249189.16666667, 249189.16666667], [245713.83333333, 245713.83333333, 245713.83333333], [245713.83333333, 245713.83333333, 245713.83333333], [248669.5 , 248669.5 , 248669.5 ], [248487.16666667, 248487.16666667, 248487.16666667], [246900.5 , 246900.5 , 246900.5 ]], [[251346.16666667, 251346.16666667, 251346.16666667], [250717.33333333, 250717.33333333, 250717.33333333], [247236.16666667, 247236.16666667, 247236.16666667], [247236.16666667, 247236.16666667, 247236.16666667], [250206.66666667, 250206.66666667, 250206.66666667], [250015.33333333, 250015.33333333, 250015.33333333], [248411.16666667, 248411.16666667, 248411.16666667]]])
- area(time, NC, sample, z)float321.0 1.0 1.0 1.0 ... 0.0 0.0 0.0 0.0
- units :
- -
- long_name :
- Fractional area contained in mask
array([[[[1., 1., 1., ..., 1., 1., 1.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], [[1., 1., 1., ..., 1., 1., 1.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], [[1., 1., 1., ..., 1., 1., 1.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], ..., [[1., 1., 1., ..., 1., 1., 1.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], [[1., 1., 1., ..., 1., 1., 1.], [0., 0., 0., ..., 0., 0., 0.], ... [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], [[1., 1., 1., ..., 1., 1., 1.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], ..., [[1., 1., 1., ..., 1., 1., 1.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], [[1., 1., 1., ..., 1., 1., 1.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], [[1., 1., 1., ..., 1., 1., 1.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]]]], dtype=float32)
- areah(time, NC, sample, zh)float321.0 1.0 1.0 1.0 ... 0.0 0.0 0.0 0.0
- units :
- -
- long_name :
- Fractional area contained in mask
array([[[[1., 1., 1., ..., 1., 1., 1.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], [[1., 1., 1., ..., 1., 1., 1.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], [[1., 1., 1., ..., 1., 1., 1.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], ..., [[1., 1., 1., ..., 1., 1., 1.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], [[1., 1., 1., ..., 1., 1., 1.], [0., 0., 0., ..., 0., 0., 0.], ... [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], [[1., 1., 1., ..., 1., 1., 1.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], ..., [[1., 1., 1., ..., 1., 1., 1.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], [[1., 1., 1., ..., 1., 1., 1.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], [[1., 1., 1., ..., 1., 1., 1.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]]]], dtype=float32)
- u(time, NC, sample, z)float32-0.09499 -0.09687 ... nan nan
- units :
- m s-1
- long_name :
- U velocity
array([[[[-9.49917212e-02, -9.68650654e-02, -8.95264447e-02, ..., -1.30459642e+01, -1.31258478e+01, -1.31902342e+01], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-1.67487394e-02, 5.63607842e-04, 2.34647561e-02, ..., -1.30383606e+01, -1.31147003e+01, -1.31708059e+01], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 5.64690344e-02, 9.15815011e-02, 1.28741562e-01, ..., -1.30466280e+01, -1.31305094e+01, -1.31981239e+01], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -1.59149802e+00, -2.06409812e+00, -2.54042363e+00], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-2.09977627e+00, -2.49809241e+00, -2.82182002e+00, ..., -1.46103466e+00, -1.93254316e+00, -2.41721559e+00], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-2.08752060e+00, -2.48598742e+00, -2.80993962e+00, ..., -1.50068474e+00, -1.97253859e+00, -2.45660615e+00], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- u_3(time, NC, sample, z)float32-0.001409 -0.0009854 ... nan nan
- units :
- m3 s-3
- long_name :
- Moment 3 of the U velocity
array([[[[-1.40902773e-03, -9.85385966e-04, -1.59479052e-04, ..., 2.62038526e-03, 1.91816140e-03, 9.36055323e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 1.43977255e-03, 2.87981145e-03, 4.21256153e-03, ..., 2.38896420e-04, 7.26173923e-04, 1.20713946e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 1.00412208e-03, 1.59067556e-03, 2.04086746e-03, ..., -1.82128523e-03, -5.86811570e-04, -1.91333165e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -1.40808960e-02, -3.07288263e-02, -3.62359621e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 4.48108232e-03, 6.49248622e-03, 8.41626711e-03, ..., -3.49943265e-02, -3.38279046e-02, -2.12429855e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 5.94858965e-03, 7.49488873e-03, 7.74336653e-03, ..., -3.00069642e-03, -2.56332080e-03, -9.12722957e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- u_4(time, NC, sample, z)float320.01919 0.03403 0.04558 ... nan nan
- units :
- m4 s-4
- long_name :
- Moment 4 of the U velocity
array([[[[0.01918945, 0.03403257, 0.04558229, ..., 0.01016053, 0.00863376, 0.0082782 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00906352, 0.01653056, 0.02278082, ..., 0.00661484, 0.00576363, 0.0057016 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00407337, 0.00726029, 0.01000305, ..., 0.01024383, 0.00929487, 0.00795281], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[0.01967291, 0.03420463, 0.04213536, ..., 0.25425413, 0.30869257, 0.37556842], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.01229064, 0.021283 , 0.02697428, ..., 0.2518671 , 0.28112355, 0.30377305], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.01996377, 0.03464783, 0.0410837 , ..., 0.28225797, 0.3465459 , 0.4004334 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- u_diff(time, NC, sample, zh)float320.004834 -0.0002237 ... nan nan
- units :
- m2 s-2
- long_name :
- Diffusive flux of the U velocity
array([[[[ 4.8337313e-03, -2.2370182e-04, -3.0375787e-03, ..., 5.0083078e-03, 4.4756564e-03, 3.7104320e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 2.6823976e-04, -5.1314686e-03, -8.2919905e-03, ..., 5.1225591e-03, 4.2030192e-03, 3.1180584e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-3.5626304e-03, -9.3061673e-03, -1.2770951e-02, ..., 5.8964840e-03, 5.0147320e-03, 4.0007792e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 4.5493256e-02, 4.5931518e-02, 4.6126273e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 8.8402569e-02, 8.1081741e-02, 7.5889923e-02, ..., 4.7641065e-02, 4.8390687e-02, 4.8661698e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 8.9991041e-02, 8.2294174e-02, 7.6785348e-02, ..., 4.4840824e-02, 4.5126006e-02, 4.6265598e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- u_w(time, NC, sample, zh)float320.0 -0.000416 ... nan nan
- units :
- m2 s-2
- long_name :
- Turbulent flux of the U velocity
array([[[[ 0.00000000e+00, -4.16043913e-04, -9.52353410e-04, ..., 3.13887000e-03, 2.08051922e-03, 1.08090532e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, -2.60635716e-04, -6.17534562e-04, ..., 3.31096421e-03, 3.08820326e-03, 2.80047231e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, -2.76613369e-04, -6.60845137e-04, ..., 3.51774110e-03, 3.21261119e-03, 2.75658048e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 1.58949830e-02, 1.73573103e-02, 1.84237305e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 1.20568695e-03, 2.54518702e-03, ..., 1.66938379e-02, 1.77665502e-02, 1.87248122e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 1.42514578e-03, 2.94300099e-03, ..., 1.76848453e-02, 1.90284271e-02, 1.90232154e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- u_grad(time, NC, sample, zh)float32-0.009499 -0.0001703 ... nan nan
- units :
- s-1
- long_name :
- Gradient of the U velocity
array([[[[-9.49917268e-03, -1.70304163e-04, 6.11551455e-04, ..., -1.99707877e-03, -1.60965521e-03, -1.18984294e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-1.67487410e-03, 1.57385028e-03, 1.90842885e-03, ..., -1.90849928e-03, -1.40263047e-03, -9.35917778e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 5.64690307e-03, 3.19204223e-03, 3.09667177e-03, ..., -2.09703553e-03, -1.69031892e-03, -1.24180259e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -1.18149975e-02, -1.19081447e-02, -1.23671191e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-2.09977672e-01, -3.62105221e-02, -2.69773006e-02, ..., -1.17877126e-02, -1.21168131e-02, -1.26073137e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-2.08752051e-01, -3.62242796e-02, -2.69960091e-02, ..., -1.17963487e-02, -1.21016940e-02, -1.23827755e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- u_2(time, NC, sample, z)float320.07758 0.1033 0.1193 ... nan nan
- units :
- m2 s-2
- long_name :
- Moment 2 of the U velocity
array([[[[0.07757594, 0.10334253, 0.11932987, ..., 0.05822359, 0.05638469, 0.05637448], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.05485221, 0.07437593, 0.08756799, ..., 0.04853062, 0.04446862, 0.04261628], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.03722739, 0.04992924, 0.05872838, ..., 0.05875093, 0.05609078, 0.05301555], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[0.07946972, 0.10871731, 0.12318816, ..., 0.2886051 , 0.32145718, 0.35842928], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.06079409, 0.08316822, 0.09457625, ..., 0.30183765, 0.3181478 , 0.32992613], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.07831373, 0.10593799, 0.11652273, ..., 0.31453082, 0.34970632, 0.3716754 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- u_path(time, NC, sample)float32-4.716e+03 nan nan ... nan nan
- units :
- m-1 s-1 kg
- long_name :
- U velocity path
array([[[-4715.812 , nan, nan], [-4754.7944 , nan, nan], [-4701.4146 , nan, nan], [-4701.4146 , nan, nan], [-4761.2563 , nan, nan], [-4768.281 , nan, nan], [-4731.2886 , nan, nan]], [[-4502.8438 , nan, nan], [-4536.906 , nan, nan], [-4475.775 , nan, nan], [-4475.775 , nan, nan], [-4532.352 , nan, nan], [-4542.9644 , nan, nan], [-4498.607 , nan, nan]], [[-3741.0918 , nan, nan], [-3767.9307 , nan, nan], [-3703.6038 , nan, nan], [-3703.6038 , nan, nan], ... [44743.484 , nan, nan], [44775. , nan, nan], [44678.77 , nan, nan], [44734.082 , nan, nan]], [[41304.848 , nan, nan], [41262.37 , nan, nan], [41446.03 , nan, nan], [41446.03 , nan, nan], [41474.35 , nan, nan], [41373.203 , nan, nan], [41437.78 , nan, nan]], [[37084.523 , nan, nan], [37066.5 , nan, nan], [37194.44 , nan, nan], [37194.44 , nan, nan], [37222.44 , nan, nan], [37134.44 , nan, nan], [37184.668 , nan, nan]]], dtype=float32)
- u_flux(time, NC, sample, zh)float320.004834 -0.0006397 ... nan nan
- units :
- m2 s-2
- long_name :
- Total flux of the U velocity
array([[[[ 0.00483373, -0.00063975, -0.00398993, ..., 0.00814718, 0.00655618, 0.00479134], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00026824, -0.0053921 , -0.00890952, ..., 0.00843352, 0.00729122, 0.00591853], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.00356263, -0.00958278, -0.0134318 , ..., 0.00941422, 0.00822734, 0.00675736], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 0.09183112, 0.08559972, 0.08162395, ..., 0.06138824, 0.06328883, 0.06455 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.08840257, 0.08228742, 0.07843512, ..., 0.06433491, 0.06615724, 0.06738651], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.08999104, 0.08371932, 0.07972834, ..., 0.06252567, 0.06415442, 0.06528881], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- v(time, NC, sample, z)float324.354 5.359 6.127 ... nan nan nan
- units :
- m s-1
- long_name :
- V velocity
array([[[[ 4.354159 , 5.3590884, 6.127005 , ..., 11.668595 , 12.139609 , 12.615883 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 4.307282 , 5.3010173, 6.0629115, ..., 11.610049 , 12.0808 , 12.559289 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 4.3404784, 5.3431535, 6.112883 , ..., 11.667278 , 12.140002 , 12.618789 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 3.076462 , 3.7007847, 4.227028 , ..., -19.906942 , -20.513496 , -21.102257 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 3.0726795, 3.6933982, 4.2173495, ..., -19.530668 , -20.165392 , -20.789743 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 3.1292334, 3.761398 , 4.292082 , ..., -19.47424 , -20.089907 , -20.687008 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- v_3(time, NC, sample, z)float320.09091 0.1656 0.2339 ... nan nan
- units :
- m3 s-3
- long_name :
- Moment 3 of the V velocity
array([[[[ 9.09094736e-02, 1.65625244e-01, 2.33898476e-01, ..., -4.20214562e-03, -6.94942242e-03, -9.19930264e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 7.45725036e-02, 1.44534886e-01, 2.17775390e-01, ..., 9.46714543e-03, 5.61530283e-03, 2.56581022e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 3.98850851e-02, 7.11039528e-02, 9.92931947e-02, ..., 1.14700375e-02, 8.35625082e-03, 1.29812525e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 1.16332114e-01, 1.16775252e-01, 7.91464373e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-1.12319551e-02, -1.96370166e-02, -3.16545852e-02, ..., -5.84175773e-02, -2.25789603e-02, -6.37704181e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-2.57698540e-03, -4.94174147e-03, -8.35200679e-03, ..., -3.55744660e-02, -1.76107455e-02, 1.75447911e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- v_4(time, NC, sample, z)float320.8115 1.85 3.014 ... nan nan nan
- units :
- m4 s-4
- long_name :
- Moment 4 of the V velocity
array([[[[8.11496735e-01, 1.84975803e+00, 3.01371121e+00, ..., 7.19377995e-02, 7.78881088e-02, 8.58658180e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[3.89646530e-01, 9.07469809e-01, 1.51135457e+00, ..., 7.03007132e-02, 6.54432550e-02, 6.27623722e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[1.18167073e-01, 2.59517938e-01, 4.14338678e-01, ..., 1.13024659e-01, 1.20911896e-01, 1.22352071e-01], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 1.99051440e+00, 1.78279400e+00, 1.42681754e+00], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[5.46404012e-02, 1.25033692e-01, 2.03147843e-01, ..., 1.37845516e+00, 1.19354188e+00, 1.13856626e+00], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[4.41932194e-02, 1.18256591e-01, 2.03074574e-01, ..., 1.31161869e+00, 1.16126144e+00, 9.71093714e-01], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- v_diff(time, NC, sample, zh)float32-0.2723 -0.2668 -0.2613 ... nan nan
- units :
- m2 s-2
- long_name :
- Diffusive flux of the V velocity
array([[[[-0.2723187 , -0.26684368, -0.26133433, ..., -0.02283219, -0.02311927, -0.0225108 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.26330158, -0.25924253, -0.25516912, ..., -0.02359941, -0.02435344, -0.02418181], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.26414326, -0.2608383 , -0.25767645, ..., -0.0237529 , -0.02484685, -0.02519906], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[-0.13200468, -0.12964274, -0.12678503, ..., 0.06429256, 0.06075839, 0.05720695], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.12980865, -0.12709188, -0.12384892, ..., 0.0711123 , 0.06792122, 0.06305306], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.13495374, -0.13168573, -0.12774041, ..., 0.06274878, 0.05928886, 0.05707845], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- v_w(time, NC, sample, zh)float320.0 -0.003076 -0.006489 ... nan nan
- units :
- m2 s-2
- long_name :
- Turbulent flux of the V velocity
array([[[[ 0. , -0.00307555, -0.00648921, ..., -0.00540954, -0.00548873, -0.00607088], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , -0.00212908, -0.00454533, ..., -0.0042045 , -0.00400367, -0.00405313], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , -0.00118444, -0.00250309, ..., -0.00531726, -0.00499956, -0.00502317], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 0. , -0.00185205, -0.00410024, ..., 0.03458073, 0.03337919, 0.03106754], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , -0.00234464, -0.00511139, ..., 0.03162116, 0.02963656, 0.02944005], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , -0.00291575, -0.00637811, ..., 0.02793687, 0.02730381, 0.02530385], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- v_grad(time, NC, sample, zh)float320.4354 0.09136 0.06399 ... nan nan
- units :
- s-1
- long_name :
- Gradient of the V velocity
array([[[[ 0.43541586, 0.09135725, 0.06399304, ..., 0.01177533, 0.01190685, 0.01195674], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.4307282 , 0.09033957, 0.06349116, ..., 0.01176882, 0.0119622 , 0.01214385], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.43404785, 0.09115225, 0.06414418, ..., 0.0118181 , 0.01196966, 0.01203723], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 0.3076462 , 0.0567566 , 0.04385361, ..., -0.0151638 , -0.01471905, -0.01425529], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.30726793, 0.05642899, 0.04366258, ..., -0.0158681 , -0.01560878, -0.01507068], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.31292334, 0.05746952, 0.04422364, ..., -0.0153917 , -0.01492754, -0.0145243 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- v_2(time, NC, sample, z)float320.5385 0.8205 1.053 ... nan nan nan
- units :
- m2 s-2
- long_name :
- Moment 2 of the V velocity
array([[[[0.5384955 , 0.8205159 , 1.0525634 , ..., 0.15860043, 0.16706336, 0.17569675], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.39135894, 0.59841627, 0.77161473, ..., 0.14564537, 0.14447454, 0.14592505], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.19480985, 0.29185885, 0.37168288, ..., 0.1869903 , 0.19423968, 0.20012741], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[0.13781263, 0.21603782, 0.27521345, ..., 0.8389434 , 0.7847247 , 0.70331484], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.13425352, 0.2085383 , 0.26403055, ..., 0.7255812 , 0.6681343 , 0.6372035 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.14048027, 0.23067643, 0.30136383, ..., 0.67165256, 0.63084364, 0.5739154 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- v_path(time, NC, sample)float321.625e+04 nan nan ... nan nan
- units :
- m-1 s-1 kg
- long_name :
- V velocity path
array([[[ 1.6245251e+04, nan, nan], [ 1.6327103e+04, nan, nan], [ 1.6450805e+04, nan, nan], [ 1.6450805e+04, nan, nan], [ 1.6378855e+04, nan, nan], [ 1.6380281e+04, nan, nan], [ 1.6439955e+04, nan, nan]], [[ 2.0423604e+04, nan, nan], [ 2.0521787e+04, nan, nan], [ 2.0640174e+04, nan, nan], [ 2.0640174e+04, nan, nan], [ 2.0575502e+04, nan, nan], [ 2.0576330e+04, nan, nan], [ 2.0628928e+04, nan, nan]], [[ 2.4335977e+04, nan, nan], [ 2.4438525e+04, nan, nan], [ 2.4554469e+04, nan, nan], [ 2.4554469e+04, nan, nan], ... [ 6.1798676e+02, nan, nan], [ 5.4692499e+02, nan, nan], [ 6.2734949e+02, nan, nan], [ 6.0980713e+02, nan, nan]], [[-3.3100574e+03, nan, nan], [-3.1599929e+03, nan, nan], [-3.4127236e+03, nan, nan], [-3.4127236e+03, nan, nan], [-3.4946768e+03, nan, nan], [-3.3898298e+03, nan, nan], [-3.4215486e+03, nan, nan]], [[-7.0398613e+03, nan, nan], [-6.8704263e+03, nan, nan], [-7.1494868e+03, nan, nan], [-7.1494868e+03, nan, nan], [-7.2409648e+03, nan, nan], [-7.1154976e+03, nan, nan], [-7.1654790e+03, nan, nan]]], dtype=float32)
- v_flux(time, NC, sample, zh)float32-0.2723 -0.2699 -0.2678 ... nan nan
- units :
- m2 s-2
- long_name :
- Total flux of the V velocity
array([[[[-0.2723187 , -0.26991922, -0.26782352, ..., -0.02824172, -0.02860799, -0.02858169], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.26330158, -0.26137164, -0.25971445, ..., -0.02780391, -0.02835711, -0.02823495], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.26414326, -0.26202273, -0.26017955, ..., -0.02907016, -0.02984641, -0.03022222], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[-0.13200468, -0.13149479, -0.13088526, ..., 0.09887329, 0.09413758, 0.08827449], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.12980865, -0.12943651, -0.12896031, ..., 0.10273346, 0.09755778, 0.09249311], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.13495374, -0.1346015 , -0.13411851, ..., 0.09068566, 0.08659267, 0.0823823 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- w(time, NC, sample, zh)float320.0 1.16e-10 1.775e-10 ... nan nan
- units :
- m s-1
- long_name :
- Vertical velocity
array([[[[ 0.00000000e+00, 1.15976194e-10, 1.77543605e-10, ..., -6.43586684e-10, -6.70604905e-10, -6.13613771e-10], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 1.20320087e-10, 1.70441050e-10, ..., -4.28033692e-10, -4.29768859e-10, -4.59531885e-10], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 1.30374656e-10, 4.05349893e-10, ..., -5.25977761e-10, -4.81743478e-10, -5.50631041e-10], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -1.10604814e-09, -1.14130805e-09, -1.12637000e-09], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 4.87720475e-10, 8.62101446e-10, ..., -1.37780243e-09, -1.54771840e-09, -1.47588708e-09], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 2.68065098e-10, 5.87139670e-10, ..., -9.50123091e-10, -1.01147857e-09, -9.78468084e-10], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- w_3(time, NC, sample, zh)float320.0 -9.067e-07 ... nan nan
- units :
- m3 s-3
- long_name :
- Moment 3 of the Vertical velocity
array([[[[ 0.00000000e+00, -9.06703065e-07, -4.93891685e-06, ..., 2.80323817e-04, 2.41136935e-04, 1.95793502e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, -3.21992076e-07, -1.77402251e-06, ..., -2.30133810e-05, 1.30888320e-05, 1.89649963e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, -7.35410097e-08, -4.65083644e-07, ..., 3.61101207e-04, 3.98091885e-04, 4.33752866e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -7.71546678e-04, -8.07258941e-04, -7.66528246e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, -3.57939740e-07, -1.38005464e-06, ..., 1.29378506e-03, 1.47577142e-03, 1.69295853e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, -2.97157612e-07, -1.46258469e-06, ..., -5.94276353e-04, -5.99161664e-04, -5.01382805e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- w_4(time, NC, sample, zh)float320.0 6.668e-08 6.486e-07 ... nan nan
- units :
- m4 s-4
- long_name :
- Moment 4 of the Vertical velocity
array([[[[0.00000000e+00, 6.66771030e-08, 6.48565674e-07, ..., 9.40940401e-04, 9.42035345e-04, 9.61839047e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00000000e+00, 2.27871340e-08, 2.25901502e-07, ..., 5.23299619e-04, 5.03925898e-04, 4.90895996e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00000000e+00, 1.25350823e-08, 1.34344319e-07, ..., 1.23084930e-03, 1.23891840e-03, 1.26626110e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 4.59349295e-03, 5.04566729e-03, 5.46755129e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00000000e+00, 7.75968303e-08, 6.97235521e-07, ..., 4.87371767e-03, 5.12770889e-03, 5.47287846e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00000000e+00, 7.93894586e-08, 6.87945487e-07, ..., 2.03512283e-03, 2.13561649e-03, 2.21739407e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- w_2(time, NC, sample, zh)float320.0 9.161e-05 0.000298 ... nan nan
- units :
- m2 s-2
- long_name :
- Moment 2 of the Vertical velocity
array([[[[0.00000000e+00, 9.16085264e-05, 2.97962717e-04, ..., 1.74643099e-02, 1.75024848e-02, 1.77055653e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00000000e+00, 5.97967301e-05, 1.95054061e-04, ..., 1.37713207e-02, 1.34718912e-02, 1.32834027e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00000000e+00, 5.90166273e-05, 1.95237444e-04, ..., 2.14855373e-02, 2.14047786e-02, 2.13972572e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 3.97772528e-02, 4.17786352e-02, 4.34389412e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00000000e+00, 1.27760795e-04, 3.87271954e-04, ..., 3.96590754e-02, 4.07278873e-02, 4.19532470e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00000000e+00, 1.41293727e-04, 4.27006744e-04, ..., 2.54677460e-02, 2.61678938e-02, 2.67648324e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- p(time, NC, sample, z)float32-0.108 -0.1084 -0.1087 ... nan nan
- units :
- Pa
- long_name :
- Pressure
array([[[[-0.10800928, -0.10835731, -0.1086956 , ..., -0.11452613, -0.11444441, -0.1144923 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.10794923, -0.10819447, -0.10844407, ..., -0.11419653, -0.11386812, -0.11358403], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.13556617, -0.1357502 , -0.13596681, ..., -0.14468706, -0.14449061, -0.14439411], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[-0.23705041, -0.23741335, -0.2377463 , ..., -0.26914257, -0.27090684, -0.27265644], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.23685719, -0.23728843, -0.23767434, ..., -0.27016094, -0.27144003, -0.27255103], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.23667555, -0.23717429, -0.23758115, ..., -0.25887594, -0.25940368, -0.25994244], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- p_grad(time, NC, sample, zh)float320.0 -3.164e-05 ... nan nan
- units :
- Pa m-1
- long_name :
- Gradient of the Pressure
array([[[[ 0.00000000e+00, -3.16387777e-05, -2.81903576e-05, ..., 2.04312460e-06, -1.19728975e-06, -2.85893657e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, -2.22944054e-05, -2.08010752e-05, ..., 8.21014601e-06, 7.10233735e-06, 5.41984264e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, -1.67290782e-05, -1.80501866e-05, ..., 4.91127457e-06, 2.41244084e-06, 4.58316372e-08], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -4.41065349e-05, -4.37399030e-05, -3.44555519e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, -3.92033071e-05, -3.21595689e-05, ..., -3.19765386e-05, -2.77755607e-05, -2.66761272e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, -4.53412904e-05, -3.39050421e-05, ..., -1.31937495e-05, -1.34686043e-05, -1.23689542e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- p_2(time, NC, sample, z)float322.858 2.824 2.792 ... nan nan nan
- units :
- Pa2
- long_name :
- Moment 2 of the Pressure
array([[[[ 2.8579495 , 2.8240483 , 2.7924984 , ..., 2.207691 , 2.1574059 , 2.1123304 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 3.171086 , 3.1687863 , 3.1685035 , ..., 1.1986715 , 1.1707826 , 1.1495337 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 2.9313374 , 2.9286213 , 2.927327 , ..., 2.0324907 , 2.0163167 , 2.0076082 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 3.2375793 , 3.2190793 , 3.2026198 , ..., 4.414969 , 4.4367547 , 4.439202 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 2.6819513 , 2.6608226 , 2.6425488 , ..., 4.36505 , 4.3610935 , 4.358871 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.47701955, 0.46205878, 0.44835815, ..., 1.9038005 , 1.9109792 , 1.9085034 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- p_w(time, NC, sample, zh)float320.0 -0.002575 -0.004335 ... nan nan
- units :
- Pa m s-1
- long_name :
- Turbulent flux of the Pressure
array([[[[ 0. , -0.00257495, -0.00433491, ..., 0.0253437 , 0.02622891, 0.02714764], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , -0.00148246, -0.00244596, ..., 0.0190824 , 0.01892645, 0.01881476], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , -0.00158928, -0.00264439, ..., 0.01583462, 0.01583607, 0.01576538], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 0. , -0.00250581, -0.00422122, ..., 0.10670681, 0.11139262, 0.11579252], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , -0.00164386, -0.00259885, ..., 0.07761899, 0.08227109, 0.08783305], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , -0.00115905, -0.00175072, ..., 0.00810947, 0.00820806, 0.0077717 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- ke(time, NC, sample, z)float329.795 14.83 19.37 ... nan nan nan
- units :
- m2 s-2
- long_name :
- Kinetic energy
array([[[[ 9.795363 , 14.830974 , 19.365995 , ..., 153.5522 , 160.21724 , 166.9752 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 9.502869 , 14.390937 , 18.814737 , ..., 152.76085 , 159.34296 , 165.98346 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 9.542724 , 14.456715 , 18.916265 , ..., 153.56331 , 160.30305 , 167.13416 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 7.1398025, 10.271957 , 13.302558 , ..., 200.46599 , 213.60085 , 226.94548 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 7.021042 , 10.084592 , 13.051422 , ..., 192.77563 , 206.17482 , 220.02295 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 7.1824226, 10.330031 , 13.365296 , ..., 191.69432 , 204.71935 , 217.97585 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- tke(time, NC, sample, z)float320.3072 0.4609 0.5849 ... nan nan
- units :
- m2 s-2
- long_name :
- Turbulent kinetic energy
array([[[[0.30719957, 0.46090594, 0.5848716 , ..., 0.11605256, 0.1193805 , 0.1237849 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.2225483 , 0.3356966 , 0.42883086, ..., 0.10304159, 0.10031692, 0.10005572], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.11558611, 0.17035629, 0.21464165, ..., 0.13234799, 0.13468122, 0.13618617], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[0.10674816, 0.15982823, 0.19634579, ..., 0.5765986 , 0.56680125, 0.54538995], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.0953754 , 0.1430042 , 0.17612737, ..., 0.5271252 , 0.5071241 , 0.49798715], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.10700534, 0.1651404 , 0.20549679, ..., 0.49899924, 0.49636483, 0.47923926], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- lat(time, NC, sample)float3236.5 36.5 36.5 ... 36.5 36.5 36.5
- units :
- degrees
- long_name :
- Latitude
array([[[36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5]], [[36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5]], [[36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], ... [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5]], [[36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5]], [[36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5]]], dtype=float32)
- lon(time, NC, sample)float32-97.5 -97.5 -97.5 ... -97.5 -97.5
- units :
- degrees
- long_name :
- Longitude
array([[[-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5]], [[-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5]], [[-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], ... [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5]], [[-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5]], [[-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5]]], dtype=float32)
- evisc(time, NC, sample, z)float322.098 3.572 4.354 ... nan nan nan
- units :
- m2 s-1
- long_name :
- Eddy viscosity
array([[[[2.0977395, 3.5720208, 4.3541985, ..., 1.6772243, 1.7104617, 1.6868349], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.0781243, 3.5316608, 4.319552 , ..., 1.7484323, 1.7766293, 1.7778598], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.0941918, 3.567627 , 4.3743362, ..., 1.6959138, 1.7910227, 1.8332195], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[1.9579177, 2.5096712, 3.1434746, ..., 3.4353645, 3.3282907, 3.2266405], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[1.9394323, 2.46546 , 3.0798695, ..., 3.6931698, 3.6100519, 3.5069816], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[1.9597093, 2.5042717, 3.120716 , ..., 3.447755 , 3.299697 , 3.2448044], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- evisc_2(time, NC, sample, z)float320.1074 0.4291 0.6318 ... nan nan
- units :
- m4 s-2
- long_name :
- Moment 2 of the Eddy viscosity
array([[[[0.10735131, 0.4290662 , 0.63181734, ..., 1.9052709 , 1.9713782 , 1.959446 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.0788307 , 0.32360795, 0.49348748, ..., 1.7801892 , 1.8902756 , 1.9255518 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.0399599 , 0.15465891, 0.25249264, ..., 2.0641997 , 2.038348 , 2.0201557 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[0.03858019, 0.20011969, 0.29435793, ..., 6.476036 , 6.4885592 , 6.426048 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.04886763, 0.20157452, 0.31760672, ..., 6.110246 , 6.153991 , 6.1157136 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.04385162, 0.23412722, 0.3406634 , ..., 5.7889557 , 5.812475 , 5.8039165 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- nr(time, NC, sample, z)float320.003148 0.003262 ... nan nan
- units :
- m-3
- long_name :
- Number density rain
array([[[[3.1479627e-03, 3.2623836e-03, 3.3518311e-03, ..., 5.4207473e-04, 4.9476390e-04, 4.5082203e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[7.9370715e-05, 8.2239239e-05, 8.4471299e-05, ..., 1.9437164e-06, 1.7863771e-06, 1.6419261e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[8.2359758e-05, 8.5319982e-05, 8.7609260e-05, ..., 2.0887869e-06, 1.8857172e-06, 1.7119846e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[7.6262302e-05, 7.9164187e-05, 8.1756261e-05, ..., 2.1335927e-03, 1.8611659e-03, 1.6149493e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.8147741e-05, 2.9195335e-05, 3.0110217e-05, ..., 1.1235340e-03, 9.9690992e-04, 8.7939418e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.5819822e-05, 2.6785527e-05, 2.7636333e-05, ..., 2.6740483e-04, 2.3645627e-04, 2.0816055e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- nr_3(time, NC, sample, z)float324.025e-12 4.235e-12 ... nan nan
- units :
- m-9
- long_name :
- Moment 3 of the Number density rain
array([[[[ 4.02455673e-12, 4.23546051e-12, 4.23310475e-12, ..., 9.15468466e-15, 1.13323903e-14, -9.41237834e-16], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 3.81188550e-17, 4.20001519e-17, 4.53645402e-17, ..., 1.18068077e-21, 4.99992512e-22, 1.57913684e-22], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-7.63993785e-19, -8.18762034e-19, -8.24912638e-19, ..., 2.09834981e-21, 7.20141755e-22, 5.26168709e-22], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 2.11824200e-12, 5.99198588e-13, -5.42659755e-14], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-1.21418930e-16, -1.34564299e-16, -1.48469378e-16, ..., 3.87546982e-13, 6.99197305e-14, -3.50754311e-14], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-1.13686680e-18, -1.18958777e-18, -1.10628707e-18, ..., 2.87408973e-15, 1.63446686e-15, -5.34798127e-16], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- nr_4(time, NC, sample, z)float328.206e-15 9.307e-15 ... nan nan
- units :
- m-12
- long_name :
- Moment 4 of the Number density rain
array([[[[8.20607467e-15, 9.30712772e-15, 1.02686626e-14, ..., 1.07605039e-17, 7.81798424e-18, 6.64601200e-18], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[7.33173182e-22, 8.21558723e-22, 8.92098539e-22, ..., 1.65672230e-27, 1.03001728e-27, 6.45728536e-28], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[5.94375832e-23, 6.24402150e-23, 6.19400645e-23, ..., 5.78941513e-27, 2.87919690e-27, 1.41365656e-27], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 2.36454956e-14, 1.48162715e-14, 8.53896709e-15], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[1.44627570e-20, 1.62000625e-20, 1.77286793e-20, ..., 9.87319073e-16, 6.99550059e-16, 5.18060650e-16], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[1.27811029e-22, 1.42831837e-22, 1.58222539e-22, ..., 2.25692280e-18, 1.66019605e-18, 1.33599761e-18], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- nr_diff(time, NC, sample, zh)float32-8.264e-05 -8.754e-05 ... nan nan
- units :
- m-2 s-1
- long_name :
- Diffusive flux of the Number density rain
array([[[[-8.26411342e-05, -8.75351907e-05, -8.73357276e-05, ..., 4.57057695e-06, 4.19627349e-06, 3.84657460e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-2.06252389e-06, -2.17984439e-06, -2.16897593e-06, ..., 1.63124039e-08, 1.51442077e-08, 1.41378758e-08], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-2.16566855e-06, -2.28179920e-06, -2.26528732e-06, ..., 2.01636166e-08, 1.82692173e-08, 1.64759886e-08], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 5.30302095e-05, 4.64568329e-05, 4.06848667e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-5.41000645e-07, -6.26841484e-07, -6.26105930e-07, ..., 2.88765150e-05, 2.59080116e-05, 2.31031572e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-5.00880844e-07, -5.82132259e-07, -5.83734334e-07, ..., 6.11107635e-06, 5.40975316e-06, 4.81929555e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- nr_w(time, NC, sample, zh)float320.0 5.269e-07 1.01e-06 ... nan nan
- units :
- m-2 s-1
- long_name :
- Turbulent flux of the Number density rain
array([[[[ 0.00000000e+00, 5.26867780e-07, 1.01025432e-06, ..., -4.83350107e-07, -4.66095003e-07, -4.69549263e-07], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 6.60265620e-09, 1.26158506e-08, ..., -1.31457123e-09, -1.10652276e-09, -9.84318071e-10], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 1.35220990e-09, 2.44131604e-09, ..., -2.53168975e-09, -2.11530549e-09, -1.53443536e-09], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -3.06533980e-06, -2.94294546e-06, -2.23952134e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 9.25427290e-09, 1.63356848e-08, ..., -4.83853910e-07, -6.24774771e-07, -9.15997987e-07], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 1.07969322e-08, 1.93824761e-08, ..., -1.61054814e-07, -9.23061805e-08, -9.81890764e-08], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- nr_grad(time, NC, sample, zh)float320.0003148 1.04e-05 ... nan nan
- units :
- m-4
- long_name :
- Gradient of the Number density rain
array([[[[ 3.14796314e-04, 1.04018955e-05, 7.45398620e-06, ..., -1.18277251e-06, -1.09854648e-06, -1.06648451e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 7.93707204e-06, 2.60775408e-07, 1.86004300e-07, ..., -3.93348509e-09, -3.61126506e-09, -3.43339246e-09], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 8.23597657e-06, 2.69111496e-07, 1.90773747e-07, ..., -5.07674214e-09, -4.34331637e-09, -3.82635346e-09], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -6.81066876e-06, -6.15541239e-06, -5.70003431e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 2.81477423e-06, 9.52356913e-08, 7.62399708e-08, ..., -3.16560181e-06, -2.93789412e-06, -2.79219580e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 2.58198247e-06, 8.77913848e-08, 7.09003842e-08, ..., -7.73714646e-07, -7.07392701e-07, -6.31861269e-07], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- nr_2(time, NC, sample, z)float327.015e-08 7.486e-08 ... nan nan
- units :
- m-6
- long_name :
- Moment 2 of the Number density rain
array([[[[7.01540444e-08, 7.48610915e-08, 7.88278172e-08, ..., 1.87821958e-09, 1.59875269e-09, 1.48401968e-09], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[1.92220077e-11, 2.04218864e-11, 2.14027546e-11, ..., 2.24398493e-14, 1.74261376e-14, 1.41901498e-14], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[4.60553514e-12, 4.73854853e-12, 4.73959977e-12, ..., 4.28393181e-14, 3.08440034e-14, 2.22561820e-14], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 8.63080416e-08, 6.92617661e-08, 5.46043601e-08], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[9.66313696e-11, 1.02290766e-10, 1.06982777e-10, ..., 1.71576939e-08, 1.46726755e-08, 1.31231639e-08], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[6.32279689e-12, 6.67718094e-12, 7.01210617e-12, ..., 8.25486179e-10, 6.98727021e-10, 6.33837427e-10], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- nr_path(time, NC, sample)float3236.91 nan nan ... 3.88 nan nan
- units :
- m-5 kg
- long_name :
- Number density rain path
array([[[3.69077530e+01, nan, nan], [6.73391819e-01, nan, nan], [6.18919075e-01, nan, nan], [6.18919075e-01, nan, nan], [2.29406312e-01, nan, nan], [1.67284310e-01, nan, nan], [1.07336678e-01, nan, nan]], [[3.67975502e+01, nan, nan], [6.71401322e-01, nan, nan], [6.16400123e-01, nan, nan], [6.16400123e-01, nan, nan], [2.28601739e-01, nan, nan], [1.66589156e-01, nan, nan], [1.06941499e-01, nan, nan]], [[3.66250458e+01, nan, nan], [6.67640865e-01, nan, nan], [6.12384379e-01, nan, nan], [6.12384379e-01, nan, nan], ... [7.84683838e+01, nan, nan], [2.30108547e+01, nan, nan], [1.35396729e+01, nan, nan], [4.12121153e+00, nan, nan]], [[1.55574424e+04, nan, nan], [9.53789673e+01, nan, nan], [7.58012161e+01, nan, nan], [7.58012161e+01, nan, nan], [2.24761887e+01, nan, nan], [1.31636772e+01, nan, nan], [3.98081136e+00, nan, nan]], [[1.52495342e+04, nan, nan], [9.27691345e+01, nan, nan], [7.38655090e+01, nan, nan], [7.38655090e+01, nan, nan], [2.21070080e+01, nan, nan], [1.29008636e+01, nan, nan], [3.87951922e+00, nan, nan]]], dtype=float32)
- nr_flux(time, NC, sample, zh)float32-8.264e-05 -8.701e-05 ... nan nan
- units :
- m-2 s-1
- long_name :
- Total flux of the Number density rain
array([[[[-8.2641134e-05, -8.7008324e-05, -8.6325475e-05, ..., 4.0872269e-06, 3.7301782e-06, 3.3770255e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-2.0625239e-06, -2.1732415e-06, -2.1563601e-06, ..., 1.4997832e-08, 1.4037685e-08, 1.3153557e-08], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-2.1656685e-06, -2.2804472e-06, -2.2628458e-06, ..., 1.7631924e-08, 1.6153910e-08, 1.4941554e-08], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 4.9964870e-05, 4.3513890e-05, 3.8445345e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-5.4100065e-07, -6.1758720e-07, -6.0977021e-07, ..., 2.8392658e-05, 2.5283238e-05, 2.2187158e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-5.0088084e-07, -5.7133525e-07, -5.6435186e-07, ..., 5.9500217e-06, 5.3174476e-06, 4.7211070e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qr(time, NC, sample, z)float322.22e-16 2.236e-16 ... nan nan
- units :
- kg kg-1
- long_name :
- Rain water specific humidity
array([[[[2.2204460e-16, 2.2362739e-16, 2.2486890e-16, ..., 2.2372507e-16, 2.2360579e-16, 2.2349343e-16], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.2204460e-16, 2.2397325e-16, 2.2548194e-16, ..., 2.2266781e-16, 2.2262173e-16, 2.2257943e-16], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.2204460e-16, 2.2435806e-16, 2.2615915e-16, ..., 2.2273168e-16, 2.2267482e-16, 2.2262575e-16], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[3.9879031e-16, 4.1335126e-16, 4.2587578e-16, ..., 2.4388368e-16, 2.4249158e-16, 2.4108630e-16], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[3.7554593e-16, 3.8938062e-16, 4.0137673e-16, ..., 2.2911876e-16, 2.2878299e-16, 2.2843370e-16], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[3.3423052e-16, 3.4655646e-16, 3.5728064e-16, ..., 2.7610122e-16, 2.7304941e-16, 2.6992166e-16], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qr_3(time, NC, sample, z)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- kg3 kg-3
- long_name :
- Moment 3 of the Rain water specific humidity
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qr_4(time, NC, sample, z)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- kg4 kg-4
- long_name :
- Moment 4 of the Rain water specific humidity
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qr_diff(time, NC, sample, zh)float32-5.882e-18 -1.192e-18 ... nan nan
- units :
- kg kg-1 m s-1
- long_name :
- Diffusive flux of the Rain water specific humidity
array([[[[-5.88247011e-18, -1.19247670e-18, -1.19681837e-18, ..., 1.15305690e-20, 1.07268039e-20, 1.00071592e-20], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-5.79815683e-18, -1.45152630e-18, -1.45543670e-18, ..., 4.77716958e-21, 4.43206401e-21, 4.15000350e-21], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-5.83872880e-18, -1.78690141e-18, -1.79049820e-18, ..., 5.64627710e-21, 5.16263130e-21, 4.71428205e-21], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 2.72837253e-19, 2.66806124e-19, 2.60538189e-19], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-7.17167576e-18, -8.24653907e-18, -8.18151523e-18, ..., 7.68798011e-20, 7.71060092e-20, 7.70576837e-20], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-6.49633118e-18, -7.45220497e-18, -7.39610393e-18, ..., 6.05926291e-19, 6.00330724e-19, 5.95907272e-19], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qr_w(time, NC, sample, zh)float320.0 4.027e-22 2.304e-21 ... nan nan
- units :
- kg kg-1 m s-1
- long_name :
- Turbulent flux of the Rain water specific humidity
array([[[[ 0.00000000e+00, 4.02657199e-22, 2.30372244e-21, ..., -1.16953413e-21, -1.14102366e-21, -1.17543658e-21], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 2.60299638e-22, 1.58521006e-21, ..., -3.88918145e-22, -3.24997339e-22, -2.87731222e-22], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 2.32686499e-23, 4.16562179e-22, ..., -7.11142935e-22, -5.97616233e-22, -4.40953455e-22], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -1.27565863e-20, -1.47042566e-20, -1.26346507e-20], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 1.11207751e-19, 1.97612841e-19, ..., -6.49325320e-22, -9.53422086e-22, -1.85437636e-21], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 1.02804566e-19, 1.84055196e-19, ..., -1.19520369e-20, -8.93570742e-21, -1.28143161e-20], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qr_grad(time, NC, sample, zh)float322.22e-17 1.439e-19 ... nan nan
- units :
- kg kg-1 m-1
- long_name :
- Gradient of the Rain water specific humidity
array([[[[ 2.22044608e-17, 1.43890885e-19, 1.03456339e-19, ..., -2.98230660e-21, -2.80853607e-21, -2.77556254e-21], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 2.22044608e-17, 1.75330094e-19, 1.25726374e-19, ..., -1.15224149e-21, -1.05684864e-21, -1.00836487e-21], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 2.22044608e-17, 2.10314845e-19, 1.50088613e-19, ..., -1.42096462e-21, -1.22710578e-21, -1.09481030e-21], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -3.48034950e-20, -3.51311458e-20, -3.63822565e-20], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 3.75545954e-17, 1.25769803e-18, 9.99675481e-19, ..., -8.39438638e-21, -8.73202632e-21, -9.31879141e-21], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 3.34230499e-17, 1.12054322e-18, 8.93679937e-19, ..., -7.62959925e-20, -7.81935449e-20, -7.78816073e-20], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qr_2(time, NC, sample, z)float320.0 2.166e-37 6.78e-37 ... nan nan
- units :
- kg2 kg-2
- long_name :
- Moment 2 of the Rain water specific humidity
array([[[[0.0000000e+00, 2.1656054e-37, 6.7797053e-37, ..., 1.1542481e-38, 1.0030577e-38, 9.6560366e-39], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.0000000e+00, 2.0457695e-37, 6.2866168e-37, ..., 1.9484691e-39, 1.4900455e-39, 1.2097494e-39], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.0000000e+00, 1.6911261e-37, 4.8701700e-37, ..., 3.2856708e-39, 2.3879331e-39, 1.7555047e-39], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[4.6747638e-34, 4.9101804e-34, 5.1327855e-34, ..., 1.9473859e-36, 1.9793629e-36, 1.9680729e-36], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[5.4105401e-34, 5.6271185e-34, 5.8222368e-34, ..., 1.0040613e-37, 1.1197119e-37, 1.2679795e-37], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.8337466e-34, 2.9799792e-34, 3.1175986e-34, ..., 6.7424524e-36, 7.3239870e-36, 8.3071692e-36], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qr_path(time, NC, sample)float322.477e-12 nan nan ... nan nan
- units :
- kg m-2
- long_name :
- Rain water specific humidity path
array([[[2.47702184e-12, nan, nan], [2.48390132e-12, nan, nan], [2.50920487e-12, nan, nan], [2.50920487e-12, nan, nan], [2.58992936e-12, nan, nan], [2.60257701e-12, nan, nan], [2.61850589e-12, nan, nan]], [[2.47666818e-12, nan, nan], [2.48302138e-12, nan, nan], [2.50766573e-12, nan, nan], [2.50766573e-12, nan, nan], [2.58592995e-12, nan, nan], [2.59927171e-12, nan, nan], [2.61752447e-12, nan, nan]], [[2.47584006e-12, nan, nan], [2.48183678e-12, nan, nan], [2.50563394e-12, nan, nan], [2.50563394e-12, nan, nan], ... [3.38543256e-12, nan, nan], [3.48320353e-12, nan, nan], [3.38130370e-12, nan, nan], [3.32723541e-12, nan, nan]], [[3.39538640e-12, nan, nan], [3.16361715e-12, nan, nan], [3.34187279e-12, nan, nan], [3.34187279e-12, nan, nan], [3.44044433e-12, nan, nan], [3.33945480e-12, nan, nan], [3.29048247e-12, nan, nan]], [[3.36109504e-12, nan, nan], [3.13615171e-12, nan, nan], [3.30498584e-12, nan, nan], [3.30498584e-12, nan, nan], [3.40443927e-12, nan, nan], [3.30392137e-12, nan, nan], [3.25813009e-12, nan, nan]]], dtype=float32)
- qr_flux(time, NC, sample, zh)float32-5.882e-18 -1.192e-18 ... nan nan
- units :
- kg kg-1 m s-1
- long_name :
- Total flux of the Rain water specific humidity
array([[[[-5.88247011e-18, -1.19207397e-18, -1.19451467e-18, ..., 1.03610357e-20, 9.58578011e-21, 8.83172258e-21], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-5.79815683e-18, -1.45126605e-18, -1.45385140e-18, ..., 4.38825131e-21, 4.10706685e-21, 3.86227188e-21], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-5.83872880e-18, -1.78687825e-18, -1.79008171e-18, ..., 4.93513402e-21, 4.56501553e-21, 4.27332854e-21], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 2.60080655e-19, 2.52101877e-19, 2.47903522e-19], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-7.17167576e-18, -8.13533208e-18, -7.98390260e-18, ..., 7.62304837e-20, 7.61525930e-20, 7.52033063e-20], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-6.49633118e-18, -7.34940131e-18, -7.21204797e-18, ..., 5.93974255e-19, 5.91395054e-19, 5.83093004e-19], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qt(time, NC, sample, z)float320.01287 0.01284 0.01281 ... nan nan
- units :
- kg kg-1
- long_name :
- Total water mixing ratio
array([[[[0.01287029, 0.01283774, 0.01281243, ..., 0.0034652 , 0.00343089, 0.00339768], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.01280969, 0.01277671, 0.01275115, ..., 0.00346343, 0.00343073, 0.00339915], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.01276983, 0.01273711, 0.0127118 , ..., 0.00346159, 0.00342722, 0.00339585], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[0.01324308, 0.01323486, 0.01322745, ..., 0.00259943, 0.00253973, 0.00248183], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.01324369, 0.01323528, 0.01322762, ..., 0.00264176, 0.00258578, 0.00253013], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.01325383, 0.01324546, 0.01323776, ..., 0.00266066, 0.00259785, 0.00253677], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qt_3(time, NC, sample, z)float32-6.863e-14 -6.587e-14 ... nan nan
- units :
- kg3 kg-3
- long_name :
- Moment 3 of the Total water mixing ratio
array([[[[-6.86283644e-14, -6.58671590e-14, -5.68206879e-14, ..., -7.04244103e-16, 1.56713885e-15, -1.99117011e-15], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-1.20937697e-13, -1.35849760e-13, -1.47553668e-13, ..., 2.98773910e-15, 1.43305015e-15, 9.20618935e-17], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-1.41049017e-15, -1.16092081e-15, -9.25048387e-16, ..., 1.55012959e-15, -9.68085814e-16, 3.01423742e-16], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -1.70527926e-14, -2.71312039e-14, -2.87716391e-14], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 1.53458314e-14, 1.57980234e-14, 1.69095169e-14, ..., 4.89087359e-16, -1.85081239e-14, -2.10561159e-14], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 3.32524298e-14, 3.34542608e-14, 3.34335695e-14, ..., 1.15985661e-15, -5.79176735e-15, -2.28340652e-14], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qt_4(time, NC, sample, z)float321.377e-16 1.562e-16 ... nan nan
- units :
- kg4 kg-4
- long_name :
- Moment 4 of the Total water mixing ratio
array([[[[1.37723759e-16, 1.56152258e-16, 1.73939539e-16, ..., 2.10297270e-18, 1.88720781e-18, 1.99201552e-18], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.87338259e-17, 3.32124861e-17, 3.75803141e-17, ..., 1.93598872e-18, 1.73045729e-18, 1.35549094e-18], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[1.40590171e-18, 1.28933748e-18, 1.25269928e-18, ..., 2.94173964e-18, 2.02081154e-18, 1.27786780e-18], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 4.42998331e-17, 3.69816438e-17, 2.91466783e-17], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[5.74116905e-18, 5.78255372e-18, 5.90687566e-18, ..., 2.65940205e-17, 2.69076377e-17, 2.71175729e-17], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[5.55513448e-18, 5.67886291e-18, 5.80068470e-18, ..., 3.08245447e-17, 2.82880119e-17, 2.94486721e-17], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qt_diff(time, NC, sample, zh)float322.366e-05 2.446e-05 ... nan nan
- units :
- kg kg-1 m s-1
- long_name :
- Diffusive flux of the Total water mixing ratio
array([[[[2.36589749e-05, 2.44553794e-05, 2.43059276e-05, ..., 3.34273159e-06, 3.19907463e-06, 3.02014246e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.39176807e-05, 2.46977634e-05, 2.44985131e-05, ..., 3.42919043e-06, 3.33454477e-06, 3.19206470e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.41104226e-05, 2.49883960e-05, 2.48563501e-05, ..., 3.44928799e-06, 3.33063940e-06, 3.14382191e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 1.16189331e-05, 1.09115817e-05, 1.03148859e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[4.02791056e-06, 4.92575646e-06, 5.10493919e-06, ..., 1.27992416e-05, 1.22783786e-05, 1.17636655e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[4.02239175e-06, 4.96608163e-06, 5.19141986e-06, ..., 1.24142507e-05, 1.16805832e-05, 1.10967221e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qt_w(time, NC, sample, zh)float320.0 -1.786e-07 ... nan nan
- units :
- kg kg-1 m s-1
- long_name :
- Turbulent flux of the Total water mixing ratio
array([[[[ 0.0000000e+00, -1.7859433e-07, -3.6079166e-07, ..., -2.5410040e-07, -2.6241665e-07, -2.8879438e-07], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.0000000e+00, -9.1905285e-08, -1.9050391e-07, ..., -2.3490503e-07, -2.0891366e-07, -1.9314109e-07], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.0000000e+00, -2.1584393e-08, -4.8769127e-08, ..., -3.6157221e-07, -3.2599658e-07, -2.5277868e-07], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -6.2624730e-07, -6.6849412e-07, -5.5643682e-07], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.0000000e+00, -1.3266053e-07, -2.4228478e-07, ..., -1.7362777e-07, -2.4139334e-07, -3.8677965e-07], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.0000000e+00, -1.8173621e-07, -3.2947253e-07, ..., -3.0179135e-07, -2.0541707e-07, -2.5850099e-07], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qt_grad(time, NC, sample, zh)float32-9.193e-05 -2.959e-06 ... nan nan
- units :
- kg kg-1 m-1
- long_name :
- Gradient of the Total water mixing ratio
array([[[[-9.1927293e-05, -2.9594521e-06, -2.1085491e-06, ..., -8.5772155e-07, -8.3040692e-07, -8.2973361e-07], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-9.3494098e-05, -2.9988857e-06, -2.1292781e-06, ..., -8.1757361e-07, -7.8938689e-07, -7.7220784e-07], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-9.2585622e-05, -2.9747891e-06, -2.1093197e-06, ..., -8.5918646e-07, -7.8420959e-07, -7.2455032e-07], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -1.4925305e-06, -1.4474172e-06, -1.4499307e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-2.1521437e-05, -7.6519427e-07, -6.3771967e-07, ..., -1.3995486e-06, -1.3912368e-06, -1.4229577e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-2.1160449e-05, -7.6053402e-07, -6.4207347e-07, ..., -1.5704244e-06, -1.5269998e-06, -1.4548618e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qt_2(time, NC, sample, z)float329.334e-09 1.002e-08 ... nan nan
- units :
- kg2 kg-2
- long_name :
- Moment 2 of the Total water mixing ratio
array([[[[9.3335766e-09, 1.0018840e-08, 1.0625603e-08, ..., 8.3812363e-10, 7.9615309e-10, 8.1174178e-10], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[3.8155821e-09, 4.1742236e-09, 4.5006936e-09, ..., 8.1970358e-10, 7.3845979e-10, 6.5589961e-10], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[7.0571343e-10, 6.8789358e-10, 6.8623168e-10, ..., 9.8909736e-10, 8.3449442e-10, 6.8084488e-10], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[1.6209363e-09, 1.6294991e-09, 1.6388580e-09, ..., 3.7198344e-09, 3.4511771e-09, 3.1794165e-09], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[1.7287322e-09, 1.7309620e-09, 1.7380382e-09, ..., 2.8781697e-09, 2.8805580e-09, 2.9996368e-09], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[1.1571061e-09, 1.1714539e-09, 1.1858753e-09, ..., 3.0509042e-09, 2.9214273e-09, 3.0023859e-09], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qt_path(time, NC, sample)float3235.09 nan nan ... 35.23 nan nan
- units :
- kg m-2
- long_name :
- Total water mixing ratio path
array([[[35.09441 , nan, nan], [35.075603 , nan, nan], [35.08698 , nan, nan], [35.08698 , nan, nan], [35.0945 , nan, nan], [35.093426 , nan, nan], [35.07809 , nan, nan]], [[34.916935 , nan, nan], [34.89986 , nan, nan], [34.911453 , nan, nan], [34.911453 , nan, nan], [34.9231 , nan, nan], [34.924908 , nan, nan], [34.90865 , nan, nan]], [[34.736526 , nan, nan], [34.72115 , nan, nan], [34.73328 , nan, nan], [34.73328 , nan, nan], ... [36.110443 , nan, nan], [36.109676 , nan, nan], [36.12029 , nan, nan], [36.099503 , nan, nan]], [[35.64555 , nan, nan], [35.613373 , nan, nan], [35.591663 , nan, nan], [35.591663 , nan, nan], [35.586906 , nan, nan], [35.60393 , nan, nan], [35.586308 , nan, nan]], [[35.284374 , nan, nan], [35.255238 , nan, nan], [35.230633 , nan, nan], [35.230633 , nan, nan], [35.22034 , nan, nan], [35.242916 , nan, nan], [35.229443 , nan, nan]]], dtype=float32)
- qt_flux(time, NC, sample, zh)float322.366e-05 2.428e-05 ... nan nan
- units :
- kg kg-1 m s-1
- long_name :
- Total flux of the Total water mixing ratio
array([[[[2.36589749e-05, 2.42767856e-05, 2.39451347e-05, ..., 3.08863127e-06, 2.93665812e-06, 2.73134810e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.39176807e-05, 2.46058571e-05, 2.43080085e-05, ..., 3.19428545e-06, 3.12563066e-06, 2.99892349e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.41104226e-05, 2.49668101e-05, 2.48075794e-05, ..., 3.08771564e-06, 3.00464239e-06, 2.89104287e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 1.09926868e-05, 1.02430868e-05, 9.75844978e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[4.02791056e-06, 4.79309665e-06, 4.86265481e-06, ..., 1.26256127e-05, 1.20369850e-05, 1.13768847e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[4.02239175e-06, 4.78434504e-06, 4.86194767e-06, ..., 1.21124604e-05, 1.14751665e-05, 1.08382219e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thl(time, NC, sample, z)float32301.9 301.9 302.0 ... nan nan nan
- units :
- K
- long_name :
- Liquid water potential temperature
array([[[[301.8982 , 301.92798, 301.95386, ..., 315.44577, 315.50064, 315.55667], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[301.96854, 301.9997 , 302.02646, ..., 315.45566, 315.50854, 315.56235], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[302.00003, 302.03192, 302.05902, ..., 315.4506 , 315.5055 , 315.55826], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[305.51022, 305.59842, 305.67416, ..., 316.77048, 316.8988 , 317.02823], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[305.4972 , 305.58676, 305.66403, ..., 316.71423, 316.8317 , 316.95273], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[305.46396, 305.5525 , 305.62918, ..., 316.69632, 316.82654, 316.95792], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thl_3(time, NC, sample, z)float320.0001191 0.0001081 ... nan nan
- units :
- K3
- long_name :
- Moment 3 of the Liquid water potential temperature
array([[[[ 1.19082608e-04, 1.08137996e-04, 7.05633138e-05, ..., 1.02648119e-05, 6.94127721e-07, 1.85313511e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 1.43887548e-04, 1.68289713e-04, 1.91936459e-04, ..., -7.71501436e-06, -1.76041738e-06, 6.52918015e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 1.24668650e-05, 1.17692034e-05, 1.09536277e-05, ..., 1.86890259e-06, 1.10436013e-05, 5.85230237e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 3.54481715e-04, 4.74084984e-04, 5.08841767e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-1.95707171e-03, -1.69025920e-03, -1.57942355e-03, ..., 9.09579467e-05, 2.67619384e-04, 2.93127930e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-1.16207253e-03, -1.12733431e-03, -1.08166819e-03, ..., 8.50136639e-05, 1.56937647e-04, 3.27268732e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thl_4(time, NC, sample, z)float320.0007332 0.0008012 ... nan nan
- units :
- K4
- long_name :
- Moment 4 of the Liquid water potential temperature
array([[[[7.33237190e-04, 8.01223796e-04, 8.65319103e-04, ..., 1.25900433e-05, 1.34630964e-05, 1.80966836e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[1.00472163e-04, 1.09841116e-04, 1.18703116e-04, ..., 1.27009125e-05, 1.38067408e-05, 1.31977240e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[7.21509650e-06, 7.25783457e-06, 7.25815653e-06, ..., 1.68208189e-05, 1.39780577e-05, 1.10715519e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 8.75383615e-04, 8.69894866e-04, 8.19602807e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[9.69555171e-04, 7.56952039e-04, 6.74618350e-04, ..., 4.61318094e-04, 5.60232962e-04, 6.52994961e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[6.04892382e-04, 5.74022008e-04, 5.32370759e-04, ..., 5.15479071e-04, 5.44183247e-04, 6.62755396e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thl_diff(time, NC, sample, zh)float32-0.01687 -0.02254 ... nan nan
- units :
- K m s-1
- long_name :
- Diffusive flux of the Liquid water potential temperature
array([[[[-1.6871180e-02, -2.2537628e-02, -2.4876773e-02, ..., -5.3352290e-03, -5.3698174e-03, -5.3868666e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-1.7886596e-02, -2.3501547e-02, -2.5716608e-02, ..., -5.5510304e-03, -5.6670480e-03, -5.7420996e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-1.8883569e-02, -2.4556058e-02, -2.6759094e-02, ..., -5.4966123e-03, -5.5814814e-03, -5.5973320e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -2.4973677e-02, -2.4360815e-02, -2.3956386e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-4.4890106e-02, -5.2938614e-02, -5.2339327e-02, ..., -2.6878783e-02, -2.6723184e-02, -2.6560239e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-4.5115355e-02, -5.3048674e-02, -5.2460879e-02, ..., -2.5752222e-02, -2.5138177e-02, -2.4810081e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thl_w(time, NC, sample, zh)float320.0 0.0002958 0.0005635 ... nan nan
- units :
- K m s-1
- long_name :
- Turbulent flux of the Liquid water potential temperature
array([[[[ 0.00000000e+00, 2.95782025e-04, 5.63540787e-04, ..., 4.08346794e-04, 4.40372387e-04, 5.08862955e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 1.39292955e-04, 2.68803560e-04, ..., 4.30678105e-04, 3.89658991e-04, 3.65182961e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 4.30848741e-05, 8.13320294e-05, ..., 6.11364783e-04, 5.90903859e-04, 5.19957393e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 1.28881005e-03, 1.46214233e-03, 1.27503776e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 3.44798871e-04, 6.36458280e-04, ..., 3.31949908e-04, 4.74436121e-04, 7.91185594e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 3.59592028e-04, 6.83759805e-04, ..., 5.99523366e-04, 4.53586021e-04, 6.19524217e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thl_grad(time, NC, sample, zh)float320.07533 0.002707 ... nan nan
- units :
- K m-1
- long_name :
- Gradient of the Liquid water potential temperature
array([[[[ 7.53307045e-02, 2.70666461e-03, 2.15476588e-03, ..., 1.37130928e-03, 1.40075746e-03, 1.49094232e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 7.97946900e-02, 2.83365906e-03, 2.23094551e-03, ..., 1.32258143e-03, 1.34466635e-03, 1.39815826e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 8.24372470e-02, 2.89949193e-03, 2.25984608e-03, ..., 1.37308950e-03, 1.31893356e-03, 1.29700371e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 3.20914481e-03, 3.23564466e-03, 3.37523222e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 2.50014007e-01, 8.14160611e-03, 6.44054124e-03, ..., 2.93585868e-03, 3.02611780e-03, 3.21307569e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 2.47533903e-01, 8.04916769e-03, 6.39063865e-03, ..., 3.25494842e-03, 3.28469952e-03, 3.25188995e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thl_2(time, NC, sample, z)float320.02109 0.02211 0.02302 ... nan nan
- units :
- K2
- long_name :
- Moment 2 of the Liquid water potential temperature
array([[[[0.02109147, 0.02210594, 0.0230155 , ..., 0.00205197, 0.00212711, 0.00243432], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00717329, 0.00751971, 0.00783541, ..., 0.00210765, 0.00206895, 0.00201906], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00162212, 0.00163538, 0.00164291, ..., 0.00237253, 0.0021978 , 0.00200051], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[0.00829388, 0.00811297, 0.00791981, ..., 0.01647351, 0.01665903, 0.01674169], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.0121158 , 0.01144692, 0.01085408, ..., 0.01206279, 0.01312798, 0.01472034], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.01039871, 0.00998918, 0.0094676 , ..., 0.01247361, 0.01291636, 0.01432262], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thl_path(time, NC, sample)float323.368e+06 nan nan ... nan nan
- units :
- K kg m-2
- long_name :
- Liquid water potential temperature path
array([[[3368451.2 , nan, nan], [3368494.2 , nan, nan], [3368427.8 , nan, nan], [3368427.8 , nan, nan], [3368492. , nan, nan], [3368512.8 , nan, nan], [3368517.8 , nan, nan]], [[3368989.8 , nan, nan], [3369042. , nan, nan], [3368985.2 , nan, nan], [3368985.2 , nan, nan], [3369041.2 , nan, nan], [3369012. , nan, nan], [3368941.2 , nan, nan]], [[3369425. , nan, nan], [3369521.2 , nan, nan], [3369583.2 , nan, nan], [3369583.2 , nan, nan], ... [3384003.8 , nan, nan], [3383938. , nan, nan], [3384021.8 , nan, nan], [3384074.2 , nan, nan]], [[3383881.2 , nan, nan], [3384029.2 , nan, nan], [3383773.2 , nan, nan], [3383773.2 , nan, nan], [3383835. , nan, nan], [3383924. , nan, nan], [3383843.8 , nan, nan]], [[3383808. , nan, nan], [3383709.2 , nan, nan], [3383818.8 , nan, nan], [3383818.8 , nan, nan], [3383766.2 , nan, nan], [3383831. , nan, nan], [3383922.2 , nan, nan]]], dtype=float32)
- thl_flux(time, NC, sample, zh)float32-0.01687 -0.02224 ... nan nan
- units :
- K m s-1
- long_name :
- Total flux of the Liquid water potential temperature
array([[[[-0.01687118, -0.02224185, -0.02431323, ..., -0.00492688, -0.00492944, -0.004878 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.0178866 , -0.02336225, -0.0254478 , ..., -0.00512035, -0.00527739, -0.00537692], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.01888357, -0.02451297, -0.02667776, ..., -0.00488525, -0.00499058, -0.00507737], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[-0.04513543, -0.05275468, -0.05185753, ..., -0.02368487, -0.02289867, -0.02268135], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.04489011, -0.05259382, -0.05170287, ..., -0.02654684, -0.02624875, -0.02576905], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.04511536, -0.05268908, -0.05177712, ..., -0.0251527 , -0.02468459, -0.02419056], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- nr_bot(time, NC, sample)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- units :
- m-3
- long_name :
- Surface Number density rain
array([[[0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.]], [[0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.]], [[0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], ... [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.]], [[0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.]], [[0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.]]], dtype=float32)
- qr_bot(time, NC, sample)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- units :
- kg kg-1
- long_name :
- Surface Rain water specific humidity
array([[[0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.]], [[0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.]], [[0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], ... [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.]], [[0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.]], [[0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.]]], dtype=float32)
- qt_bot(time, NC, sample)float320.01379 0.01379 ... 0.01347 0.01347
- units :
- kg kg-1
- long_name :
- Surface Total water mixing ratio
array([[[0.01378956, 0.01378956, 0.01378956], [0.01374463, 0.01374463, 0.01374463], [0.01369569, 0.01369569, 0.01369569], [0.01369569, 0.01369569, 0.01369569], [0.01372141, 0.01372141, 0.01372141], [0.01373716, 0.01373716, 0.01373716], [0.01372085, 0.01372085, 0.01372085]], [[0.01435342, 0.01435342, 0.01435342], [0.01431251, 0.01431251, 0.01431251], [0.01424903, 0.01424903, 0.01424903], [0.01424903, 0.01424903, 0.01424903], [0.01428383, 0.01428383, 0.01428383], [0.01429186, 0.01429186, 0.01429186], [0.01426818, 0.01426818, 0.01426818]], [[0.01495252, 0.01495252, 0.01495252], [0.01490177, 0.01490177, 0.01490177], [0.01484153, 0.01484153, 0.01484153], [0.01484153, 0.01484153, 0.01484153], ... [0.01363706, 0.01363706, 0.01363706], [0.01362747, 0.01362747, 0.01362747], [0.01362967, 0.01362967, 0.01362967], [0.01363285, 0.01363285, 0.01363285]], [[0.01343158, 0.01343158, 0.01343158], [0.01349973, 0.01349973, 0.01349973], [0.01348393, 0.01348393, 0.01348393], [0.01348393, 0.01348393, 0.01348393], [0.01346918, 0.01346918, 0.01346918], [0.01347318, 0.01347318, 0.01347318], [0.01347779, 0.01347779, 0.01347779]], [[0.0134158 , 0.0134158 , 0.0134158 ], [0.01348866, 0.01348866, 0.01348866], [0.01347347, 0.01347347, 0.01347347], [0.01347347, 0.01347347, 0.01347347], [0.0134562 , 0.0134562 , 0.0134562 ], [0.01345891, 0.01345891, 0.01345891], [0.01346543, 0.01346543, 0.01346543]]], dtype=float32)
- thl_bot(time, NC, sample)float32301.1 301.1 301.1 ... 303.0 303.0
- units :
- K
- long_name :
- Surface Liquid water potential temperature
array([[[301.1449 , 301.1449 , 301.1449 ], [301.1706 , 301.1706 , 301.1706 ], [301.17566, 301.17566, 301.17566], [301.17566, 301.17566, 301.17566], [301.17178, 301.17178, 301.17178], [301.1533 , 301.1533 , 301.1533 ], [301.15213, 301.15213, 301.15213]], [[302.35922, 302.35922, 302.35922], [302.3748 , 302.3748 , 302.3748 ], [302.37445, 302.37445, 302.37445], [302.37445, 302.37445, 302.37445], [302.3777 , 302.3777 , 302.3777 ], [302.3667 , 302.3667 , 302.3667 ], [302.36658, 302.36658, 302.36658]], [[303.74527, 303.74527, 303.74527], [303.72552, 303.72552, 303.72552], [303.73523, 303.73523, 303.73523], [303.73523, 303.73523, 303.73523], ... [303.7111 , 303.7111 , 303.7111 ], [303.75247, 303.75247, 303.75247], [303.7174 , 303.7174 , 303.7174 ], [303.70557, 303.70557, 303.70557]], [[303.35815, 303.35815, 303.35815], [303.3218 , 303.3218 , 303.3218 ], [303.28793, 303.28793, 303.28793], [303.28793, 303.28793, 303.28793], [303.34378, 303.34378, 303.34378], [303.30133, 303.30133, 303.30133], [303.29636, 303.29636, 303.29636]], [[303.04904, 303.04904, 303.04904], [303.00464, 303.00464, 303.00464], [302.97113, 302.97113, 302.97113], [302.97113, 302.97113, 302.97113], [303.02817, 303.02817, 303.02817], [302.99704, 302.99704, 302.99704], [302.98862, 302.98862, 302.98862]]], dtype=float32)
- rhoref(time, NC, sample, z)float321.099 1.098 1.097 ... 0.7671 0.7642
- units :
- kg m-3
- long_name :
- Full level basic state density
array([[[[1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521]], [[1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521]], [[1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521]], ... [[1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521]], [[1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521]], [[1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521]]]], dtype=float32)
- rhorefh(time, NC, sample, zh)float321.1 1.099 1.098 ... 0.7656 0.7627
- units :
- kg m-3
- long_name :
- Half level basic state density
array([[[[1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ]], [[1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ]], [[1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ]], ... [[1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ]], [[1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ]], [[1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ]]]], dtype=float32)
- thvref(time, NC, sample, z)float32308.7 308.7 308.7 ... 317.2 317.3
- units :
- K
- long_name :
- Full level basic state virtual potential temperature
array([[[[308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ]], [[308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ]], [[308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ]], ... [[308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ]], [[308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ]], [[308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ]]]], dtype=float32)
- thvrefh(time, NC, sample, zh)float32308.7 308.7 308.7 ... 317.2 317.3
- units :
- K
- long_name :
- Half level basic state virtual potential temperature
array([[[[308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ]], [[308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ]], [[308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ]], ... [[308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ]], [[308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ]], [[308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ]]]], dtype=float32)
- phydro(time, NC, sample, z)float329.657e+04 9.645e+04 ... 6.045e+04
- units :
- Pa
- long_name :
- Full level hydrostatic pressure
array([[[[96566.07 , 96445.625, 96314.35 , ..., 60917.598, 60614.652, 60312.83 ], [96566.07 , 96445.625, 96314.35 , ..., 60917.598, 60614.652, 60312.83 ], [96566.07 , 96445.625, 96314.35 , ..., 60917.598, 60614.652, 60312.83 ]], [[96566.09 , 96445.66 , 96314.414, ..., 60917.777, 60614.832, 60313.02 ], [96566.09 , 96445.66 , 96314.414, ..., 60917.777, 60614.832, 60313.02 ], [96566.09 , 96445.66 , 96314.414, ..., 60917.777, 60614.832, 60313.02 ]], [[96566.09 , 96445.664, 96314.44 , ..., 60916.824, 60613.887, 60312.066], [96566.09 , 96445.664, 96314.44 , ..., 60916.824, 60613.887, 60312.066], [96566.09 , 96445.664, 96314.44 , ..., 60916.824, 60613.887, 60312.066]], ... [[96546.23 , 96427.26 , 96297.625, ..., 61050.668, 60748.39 , 60447.297], [96546.23 , 96427.26 , 96297.625, ..., 61050.668, 60748.39 , 60447.297], [96546.23 , 96427.26 , 96297.625, ..., 61050.668, 60748.39 , 60447.297]], [[96546.22 , 96427.24 , 96297.6 , ..., 61050.344, 60748.02 , 60446.863], [96546.22 , 96427.24 , 96297.6 , ..., 61050.344, 60748.02 , 60446.863], [96546.22 , 96427.24 , 96297.6 , ..., 61050.344, 60748.02 , 60446.863]], [[96546.21 , 96427.22 , 96297.56 , ..., 61049.152, 60746.816, 60445.676], [96546.21 , 96427.22 , 96297.56 , ..., 61049.152, 60746.816, 60445.676], [96546.21 , 96427.22 , 96297.56 , ..., 61049.152, 60746.816, 60445.676]]]], dtype=float32)
- phydroh(time, NC, sample, zh)float329.668e+04 9.651e+04 ... 6.03e+04
- units :
- Pa
- long_name :
- Half level hydrostatic pressure
array([[[[96675.9 , 96506.03 , 96380.164, ..., 60766.13 , 60463.75 , 60162.484], [96675.9 , 96506.03 , 96380.164, ..., 60766.13 , 60463.75 , 60162.484], [96675.9 , 96506.03 , 96380.164, ..., 60766.13 , 60463.75 , 60162.484]], [[96675.9 , 96506.06 , 96380.23 , ..., 60766.3 , 60463.934, 60162.676], [96675.9 , 96506.06 , 96380.23 , ..., 60766.3 , 60463.934, 60162.676], [96675.9 , 96506.06 , 96380.23 , ..., 60766.3 , 60463.934, 60162.676]], [[96675.9 , 96506.086, 96380.25 , ..., 60765.375, 60462.996, 60161.746], [96675.9 , 96506.086, 96380.25 , ..., 60765.375, 60462.996, 60161.746], [96675.9 , 96506.086, 96380.25 , ..., 60765.375, 60462.996, 60161.746]], ... [[96655.35 , 96487.56 , 96363.25 , ..., 60899.87 , 60598.184, 60297.676], [96655.35 , 96487.56 , 96363.25 , ..., 60899.87 , 60598.184, 60297.676], [96655.35 , 96487.56 , 96363.25 , ..., 60899.87 , 60598.184, 60297.676]], [[96655.35 , 96487.555, 96363.24 , ..., 60899.52 , 60597.777, 60297.207], [96655.35 , 96487.555, 96363.24 , ..., 60899.52 , 60597.777, 60297.207], [96655.35 , 96487.555, 96363.24 , ..., 60899.52 , 60597.777, 60297.207]], [[96655.35 , 96487.54 , 96363.21 , ..., 60898.332, 60596.594, 60296.03 ], [96655.35 , 96487.54 , 96363.21 , ..., 60898.332, 60596.594, 60296.03 ], [96655.35 , 96487.54 , 96363.21 , ..., 60898.332, 60596.594, 60296.03 ]]]], dtype=float32)
- rho(time, NC, sample, z)float321.117 1.116 1.115 ... 0.769 0.7659
- units :
- kg m-3
- long_name :
- Full level density
array([[[[1.1167909 , 1.1157075 , 1.114544 , ..., 0.7734673 , 0.77059954, 0.7677355 ], [1.1167909 , 1.1157075 , 1.114544 , ..., 0.7734673 , 0.77059954, 0.7677355 ], [1.1167909 , 1.1157075 , 1.114544 , ..., 0.7734673 , 0.77059954, 0.7677355 ]], [[1.1165718 , 1.1154839 , 1.1143175 , ..., 0.7734456 , 0.7705819 , 0.7677228 ], [1.1165718 , 1.1154839 , 1.1143175 , ..., 0.7734456 , 0.7705819 , 0.7677228 ], [1.1165718 , 1.1154839 , 1.1143175 , ..., 0.7734456 , 0.7705819 , 0.7677228 ]], [[1.1164823 , 1.1153917 , 1.1142241 , ..., 0.7734502 , 0.7705824 , 0.76772565], [1.1164823 , 1.1153917 , 1.1142241 , ..., 0.7734502 , 0.7705824 , 0.76772565], [1.1164823 , 1.1153917 , 1.1142241 , ..., 0.7734502 , 0.7705824 , 0.76772565]], ... [[1.1031774 , 1.1018934 , 1.1005671 , ..., 0.7718397 , 0.7688239 , 0.765814 ], [1.1031774 , 1.1018934 , 1.1005671 , ..., 0.7718397 , 0.7688239 , 0.765814 ], [1.1031774 , 1.1018934 , 1.1005671 , ..., 0.7718397 , 0.7688239 , 0.765814 ]], [[1.1032239 , 1.101935 , 1.1006032 , ..., 0.7719539 , 0.76896197, 0.76597005], [1.1032239 , 1.101935 , 1.1006032 , ..., 0.7719539 , 0.76896197, 0.76597005], [1.1032239 , 1.101935 , 1.1006032 , ..., 0.7719539 , 0.76896197, 0.76597005]], [[1.103337 , 1.1020517 , 1.1007217 , ..., 0.7719779 , 0.76895803, 0.7659435 ], [1.103337 , 1.1020517 , 1.1007217 , ..., 0.7719779 , 0.76895803, 0.7659435 ], [1.103337 , 1.1020517 , 1.1007217 , ..., 0.7719779 , 0.76895803, 0.7659435 ]]]], dtype=float32)
- rhoh(time, NC, sample, zh)float321.12 1.116 1.115 ... 0.7675 0.7644
- units :
- kg m-3
- long_name :
- Half level density
array([[[[1.1200031 , 1.1162508 , 1.1151272 , ..., 0.77203375, 0.7691679 , 0.76630306], [1.1200031 , 1.1162508 , 1.1151272 , ..., 0.77203375, 0.7691679 , 0.76630306], [1.1200031 , 1.1162508 , 1.1151272 , ..., 0.77203375, 0.7691679 , 0.76630306]], [[1.1199381 , 1.1160295 , 1.1149024 , ..., 0.77201414, 0.7691527 , 0.7662943 ], [1.1199381 , 1.1160295 , 1.1149024 , ..., 0.77201414, 0.7691527 , 0.7662943 ], [1.1199381 , 1.1160295 , 1.1149024 , ..., 0.77201414, 0.7691527 , 0.7662943 ]], [[1.1199505 , 1.1159387 , 1.1148096 , ..., 0.7720168 , 0.76915455, 0.7663018 ], [1.1199505 , 1.1159387 , 1.1148096 , ..., 0.7720168 , 0.76915455, 0.7663018 ], [1.1199505 , 1.1159387 , 1.1148096 , ..., 0.7720168 , 0.76915455, 0.7663018 ]], ... [[1.1129618 , 1.1025419 , 1.1012369 , ..., 0.77033514, 0.76732224, 0.76430994], [1.1129618 , 1.1025419 , 1.1012369 , ..., 0.77033514, 0.76732224, 0.76430994], [1.1129618 , 1.1025419 , 1.1012369 , ..., 0.77033514, 0.76732224, 0.76430994]], [[1.1130743 , 1.1025862 , 1.1012758 , ..., 0.77046126, 0.76746935, 0.76447296], [1.1130743 , 1.1025862 , 1.1012758 , ..., 0.77046126, 0.76746935, 0.76447296], [1.1130743 , 1.1025862 , 1.1012758 , ..., 0.77046126, 0.76746935, 0.76447296]], [[1.113101 , 1.102701 , 1.1013933 , ..., 0.7704713 , 0.76745415, 0.7644451 ], [1.113101 , 1.102701 , 1.1013933 , ..., 0.7704713 , 0.76745415, 0.7644451 ], [1.113101 , 1.102701 , 1.1013933 , ..., 0.7704713 , 0.76745415, 0.7644451 ]]]], dtype=float32)
- thv(time, NC, sample, z)float32304.3 304.3 304.3 ... nan nan nan
- units :
- K
- long_name :
- Virtual potential temperature
array([[[[304.2598 , 304.28384, 304.3052 , ..., 316.11017, 316.15854, 316.20834], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[304.31955, 304.3449 , 304.36722, ..., 316.1197 , 316.16644, 316.21426], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[304.34396, 304.3701 , 304.39276, ..., 316.11426, 316.16272, 316.20956], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[307.96927, 308.05667, 308.13162, ..., 317.2709 , 317.38797, 317.50644], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[307.95627, 308.04495, 308.12143, ..., 317.22275, 317.32962, 317.4401 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[307.92462, 308.0123 , 308.08817, ..., 317.20847, 317.32678, 317.44662], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thv_diff(time, NC, sample, zh)float32-0.01266 -0.01823 ... nan nan
- units :
- K m s-1
- long_name :
- Diffusive flux of the Virtual potential temperature
array([[[[-0.01266166, -0.01822587, -0.02060974, ..., -0.00470547, -0.00476744, -0.00481864], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.01363593, -0.0191509 , -0.02141886, ..., -0.00490513, -0.00503932, -0.00514163], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.01460437, -0.02015915, -0.02240271, ..., -0.00484676, -0.00495431, -0.00500586], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[-0.04474763, -0.05261072, -0.05196116, ..., -0.02277536, -0.02229609, -0.02200433], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.04450354, -0.05244974, -0.0518119 , ..., -0.02445727, -0.02439992, -0.02433401], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.04473199, -0.05255364, -0.05191881, ..., -0.02340343, -0.0229278 , -0.02270985], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thv_w(time, NC, sample, zh)float320.0 0.0002653 0.0005017 ... nan nan
- units :
- K m s-1
- long_name :
- Turbulent flux of the Virtual potential temperature
array([[[[ 0.0000000e+00, 2.6532100e-04, 5.0172850e-04, ..., 3.6051343e-04, 3.9098645e-04, 4.5450762e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.0000000e+00, 1.2350435e-04, 2.3591476e-04, ..., 3.8650425e-04, 3.5039542e-04, 3.2887291e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.0000000e+00, 3.9456529e-05, 7.3007592e-05, ..., 5.4332259e-04, 5.2961096e-04, 4.7254600e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 1.1702279e-03, 1.3355534e-03, 1.1696478e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.0000000e+00, 3.2294600e-04, 5.9658481e-04, ..., 2.9908062e-04, 4.2867320e-04, 7.1788952e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.0000000e+00, 3.2875236e-04, 6.2808598e-04, ..., 5.4240174e-04, 4.1473648e-04, 5.7072745e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thv_grad(time, NC, sample, zh)float320.05913 0.002185 ... nan nan
- units :
- K m-1
- long_name :
- Gradient of the Virtual potential temperature
array([[[[ 5.9131678e-02, 2.1846897e-03, 1.7845514e-03, ..., 1.2097259e-03, 1.2444091e-03, 1.3348531e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 6.3342802e-02, 2.3052201e-03, 1.8573670e-03, ..., 1.1685865e-03, 1.1960714e-03, 1.2529133e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 6.6170953e-02, 2.3758756e-03, 1.8900806e-03, ..., 1.2112254e-03, 1.1712750e-03, 1.1606993e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 2.9267417e-03, 2.9617480e-03, 3.1008210e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 2.4809653e-01, 8.0650141e-03, 6.3738767e-03, ..., 2.6710581e-03, 2.7628576e-03, 2.9437726e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 2.4566364e-01, 7.9727611e-03, 6.3228137e-03, ..., 2.9578058e-03, 2.9957118e-03, 2.9765144e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thv_2(time, NC, sample, z)float320.01709 0.01786 0.01854 ... nan nan
- units :
- K2
- long_name :
- Moment 2 of the Virtual potential temperature
array([[[[0.01709154, 0.01785871, 0.01854122, ..., 0.00159176, 0.00167007, 0.00193939], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00581397, 0.00605531, 0.00627067, ..., 0.00164499, 0.00163403, 0.00161434], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00130853, 0.00132475, 0.00133275, ..., 0.00183297, 0.00172056, 0.00158957], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[0.0076741 , 0.00746953, 0.0072485 , ..., 0.01365561, 0.01392509, 0.01410683], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.01127811, 0.01060609, 0.01000253, ..., 0.00995218, 0.01091951, 0.0123275 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00978331, 0.00934559, 0.00879309, ..., 0.01025839, 0.01070793, 0.01196146], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thv_flux(time, NC, sample, zh)float32-0.01266 -0.01796 ... nan nan
- units :
- K m s-1
- long_name :
- Total flux of the Virtual potential temperature
array([[[[-0.01266166, -0.01796055, -0.02010801, ..., -0.00434496, -0.00437645, -0.00436413], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.01363593, -0.0190274 , -0.02118294, ..., -0.00451862, -0.00468892, -0.00481276], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.01460437, -0.02011969, -0.0223297 , ..., -0.00430344, -0.00442469, -0.00453331], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[-0.04474763, -0.05229161, -0.05137682, ..., -0.02160514, -0.02096053, -0.02083468], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.04450354, -0.05212678, -0.05121532, ..., -0.02415819, -0.02397125, -0.02361612], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.04473199, -0.05222489, -0.05129072, ..., -0.02286103, -0.02251306, -0.02213912], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- T(time, NC, sample, z)float32298.9 298.8 298.7 ... nan nan nan
- units :
- K
- long_name :
- Absolute temperature
array([[[[298.90024, 298.8232 , 298.73257, ..., 273.807 , 273.46497, 273.12387], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[298.96994, 298.8942 , 298.80447, ..., 273.81577, 273.47202, 273.129 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[299.00107, 298.92612, 298.8367 , ..., 273.81018, 273.46817, 273.12424], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[302.45862, 302.4394 , 302.39807, ..., 275.12784, 274.84943, 274.57175], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[302.44568, 302.42783, 302.38806, ..., 275.0787 , 274.7908 , 274.50586], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[302.4128 , 302.3939 , 302.3536 , ..., 275.0618 , 274.78497, 274.509 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- T_2(time, NC, sample, z)float320.02067 0.02165 0.02253 ... nan nan
- units :
- K2
- long_name :
- Moment 2 of the Absolute temperature
array([[[[0.0206748 , 0.02165378, 0.0225272 , ..., 0.001546 , 0.00159805, 0.00182364], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00703154, 0.00736588, 0.00766915, ..., 0.00158796, 0.00155439, 0.00151258], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00159006, 0.00160192, 0.00160804, ..., 0.00178752, 0.00165116, 0.00149866], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[0.00812905, 0.00794615, 0.00775098, ..., 0.01242699, 0.01253137, 0.01255786], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.01187499, 0.01121152, 0.0106227 , ..., 0.00909968, 0.00987519, 0.01104161], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.01019207, 0.00978379, 0.0092658 , ..., 0.00940949, 0.00971589, 0.01074316], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- ql(time, NC, sample, z)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- kg kg-1
- long_name :
- Liquid water
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- ql_diff(time, NC, sample, zh)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- kg kg-1 m s-1
- long_name :
- Diffusive flux of the Liquid water
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- ql_w(time, NC, sample, zh)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- kg kg-1 m s-1
- long_name :
- Turbulent flux of the Liquid water
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- ql_frac(time, NC, sample, z)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- -
- long_name :
- Liquid water fraction
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- ql_grad(time, NC, sample, zh)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- kg kg-1 m-1
- long_name :
- Gradient of the Liquid water
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- ql_cover(time, NC, sample)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- units :
- -
- long_name :
- Liquid water cover
array([[[0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00]], [[0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00]], [[0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], ... [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00]], [[0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00]], [[0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00]]], dtype=float32)
- ql_path(time, NC, sample)float320.0 nan nan 0.0 ... nan 0.0 nan nan
- units :
- kg m-2
- long_name :
- Liquid water path
array([[[0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan]], [[0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan]], [[0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], ... [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan]], [[0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan]], [[0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan]]], dtype=float32)
- ql_flux(time, NC, sample, zh)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- kg kg-1 m s-1
- long_name :
- Total flux of the Liquid water
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qi(time, NC, sample, z)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- kg kg-1
- long_name :
- Ice
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qi_frac(time, NC, sample, z)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- -
- long_name :
- Ice fraction
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qi_cover(time, NC, sample)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- units :
- -
- long_name :
- Ice cover
array([[[0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.]], [[0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.]], [[0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], ... [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.]], [[0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.]], [[0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.]]], dtype=float32)
- qi_path(time, NC, sample)float320.0 nan nan 0.0 ... nan 0.0 nan nan
- units :
- kg m-2
- long_name :
- Ice path
array([[[ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan]], [[ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan]], [[ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], ... [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan]], [[ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan]], [[ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan]]], dtype=float32)
- qlqi(time, NC, sample, z)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- kg kg-1
- long_name :
- Liquid water and ice
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qlqi_frac(time, NC, sample, z)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- -
- long_name :
- Liquid water and ice fraction
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qlqi_cover(time, NC, sample)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- units :
- -
- long_name :
- Liquid water and ice cover
array([[[0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00]], [[0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00]], [[0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], ... [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00]], [[0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00]], [[0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00]]], dtype=float32)
- qlqi_path(time, NC, sample)float320.0 nan nan 0.0 ... nan 0.0 nan nan
- units :
- kg m-2
- long_name :
- Liquid water and ice path
array([[[0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan]], [[0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan]], [[0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], ... [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan]], [[0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan]], [[0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan]]], dtype=float32)
- qsat(time, NC, sample, z)float320.02161 0.02154 0.02145 ... nan nan
- units :
- kg kg-1
- long_name :
- Saturated water vapor
array([[[[0.02161005, 0.02153743, 0.02144999, ..., 0.00657082, 0.00644176, 0.00631528], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.02170012, 0.02162902, 0.02154239, ..., 0.00657497, 0.00644505, 0.00631761], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.02174058, 0.02167029, 0.02158395, ..., 0.00657241, 0.00644335, 0.00631553], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[0.02669767, 0.02670103, 0.02667287, ..., 0.00721213, 0.00710463, 0.00699882], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.02667759, 0.02668304, 0.02665732, ..., 0.00718671, 0.00707472, 0.00696567], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.02662607, 0.02662994, 0.02660333, ..., 0.00717813, 0.00707188, 0.00696737], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qsat_path(time, NC, sample)float3262.67 nan nan ... 70.2 nan nan
- units :
- kg m-2
- long_name :
- Saturated water vapor path
array([[[62.673542 , nan, nan], [62.68858 , nan, nan], [62.602005 , nan, nan], [62.601994 , nan, nan], [62.60074 , nan, nan], [62.61755 , nan, nan], [62.593243 , nan, nan]], [[62.90163 , nan, nan], [62.912807 , nan, nan], [62.845795 , nan, nan], [62.845768 , nan, nan], [62.83283 , nan, nan], [62.85103 , nan, nan], [62.837982 , nan, nan]], [[63.261566 , nan, nan], [63.273342 , nan, nan], [63.17818 , nan, nan], [63.178196 , nan, nan], ... [70.8692 , nan, nan], [70.84326 , nan, nan], [70.82967 , nan, nan], [70.79688 , nan, nan]], [[70.70423 , nan, nan], [70.737206 , nan, nan], [70.6531 , nan, nan], [70.65298 , nan, nan], [70.62322 , nan, nan], [70.61326 , nan, nan], [70.519394 , nan, nan]], [[70.382965 , nan, nan], [70.43129 , nan, nan], [70.346855 , nan, nan], [70.346794 , nan, nan], [70.32101 , nan, nan], [70.28391 , nan, nan], [70.20156 , nan, nan]]], dtype=float32)
- rh(time, NC, sample, z)float320.5957 0.5962 0.5974 ... nan nan
- units :
- -
- long_name :
- Relative humidity
array([[[[0.5956568 , 0.5961567 , 0.5974106 , ..., 0.52737933, 0.53261906, 0.5380284 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.5903401 , 0.5907562 , 0.5919464 , ..., 0.5267768 , 0.5323208 , 0.5380596 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.5873893 , 0.58778363, 0.5889613 , ..., 0.5267046 , 0.5319185 , 0.5377149 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[0.4960793 , 0.49570993, 0.4959568 , ..., 0.36051726, 0.35756764, 0.35469756], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.49648312, 0.4960666 , 0.4962586 , ..., 0.3676584 , 0.3655691 , 0.36331007], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.49782053, 0.4974346 , 0.49764284, ..., 0.37073568, 0.36742303, 0.36417365], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- zi(time, NC, sample)float322.818e+04 2.818e+04 ... 3.054e+04
- units :
- m
- long_name :
- Boundary Layer Depth
array([[[28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ]], [[28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ]], [[28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], ... [29752.334, 29752.334, 29752.334], [29752.334, 29752.334, 29752.334], [29752.334, 29752.334, 29752.334], [29752.334, 29752.334, 29752.334]], [[30538. , 30538. , 30538. ], [30538. , 30538. , 30538. ], [30538. , 30538. , 30538. ], [30538. , 30538. , 30538. ], [30538. , 30538. , 30538. ], [30538. , 30538. , 30538. ], [30538. , 30538. , 30538. ]], [[30538. , 30538. , 30538. ], [30538. , 30538. , 30538. ], [30538. , 30538. , 30538. ], [30538. , 30538. , 30538. ], [30538. , 30538. , 30538. ], [30538. , 30538. , 30538. ], [30538. , 30538. , 30538. ]]], dtype=float32)
- rr(time, NC, sample)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- units :
- kg m-2 s-1
- long_name :
- Mean surface rain rate
array([[[0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00]], [[0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00]], [[0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], ... [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00]], [[0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00]], [[0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00]]], dtype=float32)
- qr_cover(time, NC, sample)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- units :
- -
- long_name :
- Rain water specific humidity cover
array([[[0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00]], [[0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00]], [[0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], ... [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00]], [[0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00]], [[0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00]]], dtype=float32)
- qr_frac(time, NC, sample, z)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- -
- long_name :
- Rain water specific humidity fraction
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- ustar(time, NC, sample)float320.5149 0.5149 ... 0.3984 0.3984
- units :
- m s-1
- long_name :
- Surface friction velocity
array([[[0.51489836, 0.51489836, 0.51489836], [0.50795513, 0.50795513, 0.50795513], [0.5115656 , 0.5115656 , 0.5115656 ], [0.5115656 , 0.5115656 , 0.5115656 ], [0.5100952 , 0.5100952 , 0.5100952 ], [0.51190597, 0.51190597, 0.51190597], [0.510747 , 0.510747 , 0.510747 ]], [[0.50229007, 0.50229007, 0.50229007], [0.49997345, 0.49997345, 0.49997345], [0.5008376 , 0.5008376 , 0.5008376 ], [0.5008376 , 0.5008376 , 0.5008376 ], [0.4982412 , 0.4982412 , 0.4982412 ], [0.49858966, 0.49858966, 0.49858966], [0.49980858, 0.49980858, 0.49980858]], [[0.49452648, 0.49452648, 0.49452648], [0.5044152 , 0.5044152 , 0.5044152 ], [0.49873543, 0.49873543, 0.49873543], [0.49873543, 0.49873543, 0.49873543], ... [0.38412952, 0.38412952, 0.38412952], [0.38917065, 0.38917065, 0.38917065], [0.38200548, 0.38200548, 0.38200548], [0.38511586, 0.38511586, 0.38511586]], [[0.388016 , 0.388016 , 0.388016 ], [0.37931672, 0.37931672, 0.37931672], [0.38153347, 0.38153347, 0.38153347], [0.38153347, 0.38153347, 0.38153347], [0.3879046 , 0.3879046 , 0.3879046 ], [0.37961766, 0.37961766, 0.37961766], [0.3847156 , 0.3847156 , 0.3847156 ]], [[0.39959124, 0.39959124, 0.39959124], [0.3908273 , 0.3908273 , 0.3908273 ], [0.393356 , 0.393356 , 0.393356 ], [0.393356 , 0.393356 , 0.393356 ], [0.39712453, 0.39712453, 0.39712453], [0.39250827, 0.39250827, 0.39250827], [0.39838925, 0.39838925, 0.39838925]]], dtype=float32)
- obuk(time, NC, sample)float32885.5 885.5 885.5 ... 109.3 109.3
- units :
- m
- long_name :
- Obukhov length
array([[[ 8.85527405e+02, 8.85527405e+02, 8.85527405e+02], [ 7.39732483e+02, 7.39732483e+02, 7.39732483e+02], [ 6.76298645e+02, 6.76298645e+02, 6.76298645e+02], [ 6.76298645e+02, 6.76298645e+02, 6.76298645e+02], [ 7.26304199e+02, 7.26304199e+02, 7.26304199e+02], [ 7.37503906e+02, 7.37503906e+02, 7.37503906e+02], [ 7.26206543e+02, 7.26206543e+02, 7.26206543e+02]], [[ 5.24071924e+03, 5.24071924e+03, 5.24071924e+03], [ 6.93332336e+02, 6.93332336e+02, 6.93332336e+02], [-1.95544275e+03, -1.95544275e+03, -1.95544275e+03], [-1.95544275e+03, -1.95544275e+03, -1.95544275e+03], [ 2.78458569e+03, 2.78458569e+03, 2.78458569e+03], [ 6.03595264e+03, 6.03595264e+03, 6.03595264e+03], [ 2.23545850e+03, 2.23545850e+03, 2.23545850e+03]], [[-3.09008179e+02, -3.09008179e+02, -3.09008179e+02], [-3.31303986e+02, -3.31303986e+02, -3.31303986e+02], [-3.28497925e+02, -3.28497925e+02, -3.28497925e+02], [-3.28497925e+02, -3.28497925e+02, -3.28497925e+02], ... [ 9.47774734e+01, 9.47774734e+01, 9.47774734e+01], [ 8.96412354e+01, 8.96412354e+01, 8.96412354e+01], [ 9.13753281e+01, 9.13753281e+01, 9.13753281e+01]], [[ 9.85804214e+01, 9.85804214e+01, 9.85804214e+01], [ 9.36997604e+01, 9.36997604e+01, 9.36997604e+01], [ 9.42589417e+01, 9.42589417e+01, 9.42589417e+01], [ 9.42589417e+01, 9.42589417e+01, 9.42589417e+01], [ 9.80484390e+01, 9.80484390e+01, 9.80484390e+01], [ 9.25349503e+01, 9.25349503e+01, 9.25349503e+01], [ 9.55584946e+01, 9.55584946e+01, 9.55584946e+01]], [[ 1.09874290e+02, 1.09874290e+02, 1.09874290e+02], [ 1.05215157e+02, 1.05215157e+02, 1.05215157e+02], [ 1.06735481e+02, 1.06735481e+02, 1.06735481e+02], [ 1.06735481e+02, 1.06735481e+02, 1.06735481e+02], [ 1.08037148e+02, 1.08037148e+02, 1.08037148e+02], [ 1.04659515e+02, 1.04659515e+02, 1.04659515e+02], [ 1.09322395e+02, 1.09322395e+02, 1.09322395e+02]]], dtype=float32)
- wl(time, NC, sample)float327.758e-15 7.758e-15 ... 1.273e-13
- units :
- m
- long_name :
- Liquid water reservoir
array([[[7.75759445e-15, 7.75759445e-15, 7.75759445e-15], [3.43826767e-16, 3.43826767e-16, 3.43826767e-16], [1.47517326e-16, 1.47517326e-16, 1.47517326e-16], [1.47517326e-16, 1.47517326e-16, 1.47517326e-16], [3.40821143e-17, 3.40821143e-17, 3.40821143e-17], [7.41979438e-18, 7.41979438e-18, 7.41979438e-18], [1.72483877e-18, 1.72483877e-18, 1.72483877e-18]], [[5.16716483e-15, 5.16716483e-15, 5.16716483e-15], [2.28339621e-16, 2.28339621e-16, 2.28339621e-16], [9.67383425e-17, 9.67383425e-17, 9.67383425e-17], [9.67383425e-17, 9.67383425e-17, 9.67383425e-17], [2.25519528e-17, 2.25519528e-17, 2.25519528e-17], [4.90102238e-18, 4.90102238e-18, 4.90102238e-18], [1.14763741e-18, 1.14763741e-18, 1.14763741e-18]], [[3.25202905e-15, 3.25202905e-15, 3.25202905e-15], [1.42786872e-16, 1.42786872e-16, 1.42786872e-16], [5.99428806e-17, 5.99428806e-17, 5.99428806e-17], [5.99428806e-17, 5.99428806e-17, 5.99428806e-17], ... [5.20163079e-12, 5.20163079e-12, 5.20163079e-12], [1.65279194e-12, 1.65279194e-12, 1.65279194e-12], [5.58753402e-13, 5.58753402e-13, 5.58753402e-13], [1.94691797e-13, 1.94691797e-13, 1.94691797e-13]], [[9.07253439e-10, 9.07253439e-10, 9.07253439e-10], [2.03742006e-11, 2.03742006e-11, 2.03742006e-11], [4.22197737e-12, 4.22197737e-12, 4.22197737e-12], [4.22197737e-12, 4.22197737e-12, 4.22197737e-12], [1.33076568e-12, 1.33076568e-12, 1.33076568e-12], [4.53986973e-13, 4.53986973e-13, 4.53986973e-13], [1.57964665e-13, 1.57964665e-13, 1.57964665e-13]], [[7.25874416e-10, 7.25874416e-10, 7.25874416e-10], [1.64815158e-11, 1.64815158e-11, 1.64815158e-11], [3.42319207e-12, 3.42319207e-12, 3.42319207e-12], [3.42319207e-12, 3.42319207e-12, 3.42319207e-12], [1.06919042e-12, 1.06919042e-12, 1.06919042e-12], [3.68114827e-13, 3.68114827e-13, 3.68114827e-13], [1.27252267e-13, 1.27252267e-13, 1.27252267e-13]]], dtype=float32)
- H(time, NC, sample)float32-19.0 -19.0 -19.0 ... -50.47 -50.47
- units :
- W m-2
- long_name :
- Surface sensible heat flux
array([[[-18.997353 , -18.997353 , -18.997353 ], [-20.139051 , -20.139051 , -20.139051 ], [-21.261312 , -21.261312 , -21.261312 ], [-21.261312 , -21.261312 , -21.261312 ], [-20.46846 , -20.46846 , -20.46846 ], [-20.164072 , -20.164072 , -20.164072 ], [-20.499102 , -20.499102 , -20.499102 ]], [[ 6.284144 , 6.284144 , 6.284144 ], [ 5.213218 , 5.213218 , 5.213218 ], [ 3.8945694, 3.8945694, 3.8945694], [ 3.8945694, 3.8945694, 3.8945694], [ 4.702566 , 4.702566 , 4.702566 ], [ 4.8372655, 4.8372655, 4.8372655], [ 4.3312125, 4.3312125, 4.3312125]], [[ 31.912851 , 31.912851 , 31.912851 ], [ 31.704275 , 31.704275 , 31.704275 ], [ 30.116907 , 30.116907 , 30.116907 ], [ 30.116907 , 30.116907 , 30.116907 ], ... [-53.348072 , -53.348072 , -53.348072 ], [-53.754948 , -53.754948 , -53.754948 ], [-53.44678 , -53.44678 , -53.44678 ], [-53.647137 , -53.647137 , -53.647137 ]], [[-51.30929 , -51.30929 , -51.30929 ], [-50.652637 , -50.652637 , -50.652637 ], [-50.80778 , -50.80778 , -50.80778 ], [-50.80778 , -50.80778 , -50.80778 ], [-51.38061 , -51.38061 , -51.38061 ], [-50.927532 , -50.927532 , -50.927532 ], [-51.253414 , -51.253414 , -51.253414 ]], [[-50.553238 , -50.553238 , -50.553238 ], [-49.77367 , -49.77367 , -49.77367 ], [-49.912403 , -49.912403 , -49.912403 ], [-49.912403 , -49.912403 , -49.912403 ], [-50.485126 , -50.485126 , -50.485126 ], [-50.21583 , -50.21583 , -50.21583 ], [-50.468983 , -50.468983 , -50.468983 ]]], dtype=float32)
- LE(time, NC, sample)float3266.26 66.26 66.26 ... 11.2 11.2
- units :
- W m-2
- long_name :
- Surface latent heat flux
array([[[ 66.26182 , 66.26182 , 66.26182 ], [ 66.98254 , 66.98254 , 66.98254 ], [ 67.52323 , 67.52323 , 67.52323 ], [ 67.52323 , 67.52323 , 67.52323 ], [ 67.222275 , 67.222275 , 67.222275 ], [ 66.88033 , 66.88033 , 66.88033 ], [ 67.02857 , 67.02857 , 67.02857 ]], [[101.17887 , 101.17887 , 101.17887 ], [101.93517 , 101.93517 , 101.93517 ], [102.766174 , 102.766174 , 102.766174 ], [102.766174 , 102.766174 , 102.766174 ], [102.350914 , 102.350914 , 102.350914 ], [102.092125 , 102.092125 , 102.092125 ], [102.41309 , 102.41309 , 102.41309 ]], [[137.85616 , 137.85616 , 137.85616 ], [138.19829 , 138.19829 , 138.19829 ], [139.31342 , 139.31342 , 139.31342 ], [139.31342 , 139.31342 , 139.31342 ], ... [ 15.981533 , 15.981533 , 15.981533 ], [ 16.073704 , 16.073704 , 16.073704 ], [ 16.003082 , 16.003082 , 16.003082 ], [ 15.981828 , 15.981828 , 15.981828 ]], [[ 11.780869 , 11.780869 , 11.780869 ], [ 11.6443815, 11.6443815, 11.6443815], [ 11.620026 , 11.620026 , 11.620026 ], [ 11.620026 , 11.620026 , 11.620026 ], [ 11.721504 , 11.721504 , 11.721504 ], [ 11.655372 , 11.655372 , 11.655372 ], [ 11.647546 , 11.647546 , 11.647546 ]], [[ 11.330916 , 11.330916 , 11.330916 ], [ 11.178574 , 11.178574 , 11.178574 ], [ 11.154429 , 11.154429 , 11.154429 ], [ 11.154429 , 11.154429 , 11.154429 ], [ 11.259244 , 11.259244 , 11.259244 ], [ 11.21286 , 11.21286 , 11.21286 ], [ 11.197764 , 11.197764 , 11.197764 ]]], dtype=float32)
- G(time, NC, sample)float32-5.177 -5.177 ... -18.46 -18.46
- units :
- W m-2
- long_name :
- Surface soil heat flux
array([[[ -5.1767116, -5.1767116, -5.1767116], [ -4.816609 , -4.816609 , -4.816609 ], [ -4.1233964, -4.1233964, -4.1233964], [ -4.1233964, -4.1233964, -4.1233964], [ -4.614084 , -4.614084 , -4.614084 ], [ -4.57466 , -4.57466 , -4.57466 ], [ -4.3756943, -4.3756943, -4.3756943]], [[ 7.223437 , 7.223437 , 7.223437 ], [ 7.4831276, 7.4831276, 7.4831276], [ 8.123116 , 8.123116 , 8.123116 ], [ 8.123116 , 8.123116 , 8.123116 ], [ 7.7030005, 7.7030005, 7.7030005], [ 7.8169436, 7.8169436, 7.8169436], [ 8.025334 , 8.025334 , 8.025334 ]], [[ 20.93782 , 20.93782 , 20.93782 ], [ 20.833899 , 20.833899 , 20.833899 ], [ 21.417398 , 21.417398 , 21.417398 ], [ 21.417398 , 21.417398 , 21.417398 ], ... [-16.132286 , -16.132286 , -16.132286 ], [-15.959836 , -15.959836 , -15.959836 ], [-16.0374 , -16.0374 , -16.0374 ], [-15.908249 , -15.908249 , -15.908249 ]], [[-17.994987 , -17.994987 , -17.994987 ], [-18.252869 , -18.252869 , -18.252869 ], [-18.096838 , -18.096838 , -18.096838 ], [-18.096838 , -18.096838 , -18.096838 ], [-17.796556 , -17.796556 , -17.796556 ], [-17.983747 , -17.983747 , -17.983747 ], [-17.770483 , -17.770483 , -17.770483 ]], [[-18.65776 , -18.65776 , -18.65776 ], [-19.000933 , -19.000933 , -19.000933 ], [-18.860939 , -18.860939 , -18.860939 ], [-18.860939 , -18.860939 , -18.860939 ], [-18.541285 , -18.541285 , -18.541285 ], [-18.627825 , -18.627825 , -18.627825 ], [-18.460947 , -18.460947 , -18.460947 ]]], dtype=float32)
- S(time, NC, sample)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- units :
- W m-2
- long_name :
- Surface storage heat flux
array([[[0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.]], [[0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.]], [[0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], ... [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.]], [[0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.]], [[0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.], [0., 0., 0.]]], dtype=float32)
- t(time, NC, sample, zs)float32289.0 293.7 298.8 ... nan nan nan
- units :
- K
- long_name :
- Soil temperature
array([[[[289.03824, 293.70065, 298.79984, 298.7689 ], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], [[289.03824, 293.70065, 298.7403 , 298.75833], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], [[289.03824, 293.70065, 298.67136, 298.694 ], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], ..., [[289.03824, 293.70065, 298.7486 , 298.73926], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], [[289.03824, 293.70065, 298.71335, 298.717 ], [ nan, nan, nan, nan], ... [ nan, nan, nan, nan], [ nan, nan, nan, nan]], [[289.03824, 293.70065, 299.9181 , 301.92776], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], ..., [[289.03824, 293.70065, 299.9243 , 301.95224], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], [[289.03824, 293.70065, 299.91248, 301.93015], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], [[289.03824, 293.70065, 299.8967 , 301.90506], [ nan, nan, nan, nan], [ nan, nan, nan, nan]]]], dtype=float32)
- theta(time, NC, sample, zs)float320.2032 0.2371 0.2555 ... nan nan
- units :
- -
- long_name :
- Soil volumetric water content
array([[[[0.20322801, 0.2371441 , 0.25546244, 0.22602469], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], [[0.20322801, 0.2371407 , 0.25543445, 0.22603874], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], [[0.20322801, 0.23715757, 0.25552115, 0.22601976], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], ..., [[0.20322801, 0.23714079, 0.25544548, 0.22604729], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], [[0.20322801, 0.23715223, 0.25550047, 0.22603868], [ nan, nan, nan, nan], ... [ nan, nan, nan, nan]], [[0.20322801, 0.23485728, 0.24125348, 0.21718068], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], ..., [[0.20322801, 0.23480965, 0.24122532, 0.21721815], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], [[0.20322801, 0.23485337, 0.2412508 , 0.21725322], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], [[0.20322801, 0.23480846, 0.24141274, 0.21726756], [ nan, nan, nan, nan], [ nan, nan, nan, nan]]]], dtype=float32)
- lw_flux_up(time, NC, sample, zh)float32445.0 446.4 446.4 ... nan nan nan
- units :
- W m-2
- long_name :
- Longwave upwelling flux
array([[[[445.0265 , 446.35837, 446.4403 , ..., 364.7571 , 363.8837 , 363.0109 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[445.17557, 446.59384, 446.6914 , ..., 364.77982, 363.90405, 363.0282 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[445.21436, 446.68265, 446.7891 , ..., 364.81882, 363.94394, 363.06577], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[456.81015, 461.64322, 462.3478 , ..., 374.00693, 373.28098, 372.5634 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[456.62854, 461.4978 , 462.21036, ..., 373.84634, 373.10107, 372.36563], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[456.57205, 461.39066, 462.09384, ..., 373.7816 , 373.04922, 372.32578], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- lw_flux_dn(time, NC, sample, zh)float32380.2 380.1 379.4 ... nan nan nan
- units :
- W m-2
- long_name :
- Longwave downwelling flux
array([[[[380.1896 , 380.09146, 379.4249 , ..., 203.56685, 201.97766, 200.39409], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[380.2678 , 380.2161 , 379.55106, ..., 203.61003, 202.01842, 200.43097], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[380.42636, 380.40213, 379.73813, ..., 203.57153, 201.97871, 200.38753], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[399.06577, 400.9324 , 400.38538, ..., 199.40503, 197.89543, 196.39917], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[399.02063, 400.91116, 400.36948, ..., 199.44334, 197.91737, 196.40819], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[398.86282, 400.72476, 400.1813 , ..., 199.50005, 197.97804, 196.46545], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- lw_flux_up_clear(time, NC, sample, zh)float32445.0 446.4 446.4 ... nan nan nan
- units :
- W m-2
- long_name :
- Clear-sky longwave upwelling flux
array([[[[445.0265 , 446.35837, 446.4403 , ..., 364.7571 , 363.8837 , 363.0109 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[445.17557, 446.59384, 446.6914 , ..., 364.77982, 363.90405, 363.0282 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[445.21436, 446.68265, 446.7891 , ..., 364.81882, 363.94394, 363.06577], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[456.81015, 461.64322, 462.3478 , ..., 374.00693, 373.28098, 372.5634 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[456.62854, 461.4978 , 462.21036, ..., 373.84634, 373.10107, 372.36563], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[456.57205, 461.39066, 462.09384, ..., 373.7816 , 373.04922, 372.32578], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- lw_flux_dn_clear(time, NC, sample, zh)float32380.2 380.1 379.4 ... nan nan nan
- units :
- W m-2
- long_name :
- Clear-sky longwave downwelling flux
array([[[[380.1896 , 380.09146, 379.4249 , ..., 203.56685, 201.97766, 200.39409], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[380.2678 , 380.2161 , 379.55106, ..., 203.61003, 202.01842, 200.43097], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[380.42636, 380.40213, 379.73813, ..., 203.57153, 201.97871, 200.38753], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[399.06577, 400.9324 , 400.38538, ..., 199.40503, 197.89543, 196.39917], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[399.02063, 400.91116, 400.36948, ..., 199.44334, 197.91737, 196.40819], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[398.86282, 400.72476, 400.1813 , ..., 199.50005, 197.97804, 196.46545], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- sw_flux_up(time, NC, sample, zh)float3222.85 22.88 22.9 ... nan nan nan
- units :
- W m-2
- long_name :
- Shortwave upwelling flux
array([[[[22.854927 , 22.878485 , 22.896006 , ..., 29.351837 , 29.419485 , 29.487097 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[22.857103 , 22.880682 , 22.898224 , ..., 29.354013 , 29.42166 , 29.489271 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[22.854868 , 22.878471 , 22.896027 , ..., 29.352058 , 29.41971 , 29.487326 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- sw_flux_dn(time, NC, sample, zh)float32130.0 130.1 130.2 ... nan nan nan
- units :
- W m-2
- long_name :
- Shortwave downwelling flux
array([[[[129.98727 , 130.12338 , 130.22444 , ..., 164.56998 , 164.88786 , 165.20677 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[129.99965 , 130.13536 , 130.23607 , ..., 164.55981 , 164.87746 , 165.19621 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[129.98694 , 130.12222 , 130.22266 , ..., 164.56802 , 164.88564 , 165.20433 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- sw_flux_dn_dir(time, NC, sample, zh)float32103.2 103.4 103.5 ... nan nan nan
- units :
- W m-2
- long_name :
- Shortwave direct downwelling flux
array([[[[103.22776 , 103.38087 , 103.49451 , ..., 142.94357 , 143.32072 , 143.6989 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[103.239845 , 103.392555 , 103.50587 , ..., 142.93344 , 143.31035 , 143.6884 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[103.227325 , 103.379616 , 103.49264 , ..., 142.94176 , 143.3186 , 143.69661 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- sw_flux_up_clear(time, NC, sample, zh)float3222.85 22.88 22.9 ... nan nan nan
- units :
- W m-2
- long_name :
- Clear-sky shortwave upwelling flux
array([[[[22.854927 , 22.878485 , 22.896006 , ..., 29.351837 , 29.419485 , 29.487097 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[22.857103 , 22.880682 , 22.898224 , ..., 29.354013 , 29.42166 , 29.489271 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[22.854868 , 22.878471 , 22.896027 , ..., 29.352058 , 29.41971 , 29.487326 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- sw_flux_dn_clear(time, NC, sample, zh)float32130.0 130.1 130.2 ... nan nan nan
- units :
- W m-2
- long_name :
- Clear-sky shortwave downwelling flux
array([[[[129.98727 , 130.12338 , 130.22444 , ..., 164.56998 , 164.88786 , 165.20677 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[129.99965 , 130.13536 , 130.23607 , ..., 164.55981 , 164.87746 , 165.19621 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[129.98694 , 130.12222 , 130.22266 , ..., 164.56802 , 164.88564 , 165.20433 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- sw_flux_dn_dir_clear(time, NC, sample, zh)float32103.2 103.4 103.5 ... nan nan nan
- units :
- W m-2
- long_name :
- Clear-sky shortwave direct downwelling flux
array([[[[103.22776 , 103.38087 , 103.49451 , ..., 142.94357 , 143.32072 , 143.6989 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[103.239845 , 103.392555 , 103.50587 , ..., 142.93344 , 143.31035 , 143.6884 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[103.227325 , 103.379616 , 103.49264 , ..., 142.94176 , 143.3186 , 143.69661 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- sw_flux_up_ref(time, NC, sample, lev)float3223.2 23.88 24.69 ... 0.1828 0.183
- units :
- W m-2
- long_name :
- Shortwave upwelling flux of reference column
array([[[[2.3201559e+01, 2.3880873e+01, 2.4692039e+01, ..., 4.8085110e+01, 4.8085346e+01, 4.8085571e+01], [2.3201559e+01, 2.3880873e+01, 2.4692039e+01, ..., 4.8085110e+01, 4.8085346e+01, 4.8085571e+01], [2.3201559e+01, 2.3880873e+01, 2.4692039e+01, ..., 4.8085110e+01, 4.8085346e+01, 4.8085571e+01]], [[2.3201559e+01, 2.3880873e+01, 2.4692039e+01, ..., 4.8085110e+01, 4.8085346e+01, 4.8085571e+01], [2.3201559e+01, 2.3880873e+01, 2.4692039e+01, ..., 4.8085110e+01, 4.8085346e+01, 4.8085571e+01], [2.3201559e+01, 2.3880873e+01, 2.4692039e+01, ..., 4.8085110e+01, 4.8085346e+01, 4.8085571e+01]], [[2.3201559e+01, 2.3880873e+01, 2.4692039e+01, ..., 4.8085110e+01, 4.8085346e+01, 4.8085571e+01], [2.3201559e+01, 2.3880873e+01, 2.4692039e+01, ..., 4.8085110e+01, 4.8085346e+01, 4.8085571e+01], [2.3201559e+01, 2.3880873e+01, 2.4692039e+01, ..., 4.8085110e+01, 4.8085346e+01, 4.8085571e+01]], ... [[2.2036446e-02, 2.2283874e-02, 2.2595389e-02, ..., 1.8251310e-01, 1.8275519e-01, 1.8299709e-01], [2.2036446e-02, 2.2283874e-02, 2.2595389e-02, ..., 1.8251310e-01, 1.8275519e-01, 1.8299709e-01], [2.2036446e-02, 2.2283874e-02, 2.2595389e-02, ..., 1.8251310e-01, 1.8275519e-01, 1.8299709e-01]], [[2.2036446e-02, 2.2283874e-02, 2.2595389e-02, ..., 1.8251310e-01, 1.8275519e-01, 1.8299709e-01], [2.2036446e-02, 2.2283874e-02, 2.2595389e-02, ..., 1.8251310e-01, 1.8275519e-01, 1.8299709e-01], [2.2036446e-02, 2.2283874e-02, 2.2595389e-02, ..., 1.8251310e-01, 1.8275519e-01, 1.8299709e-01]], [[2.2036446e-02, 2.2283874e-02, 2.2595389e-02, ..., 1.8251310e-01, 1.8275519e-01, 1.8299709e-01], [2.2036446e-02, 2.2283874e-02, 2.2595389e-02, ..., 1.8251310e-01, 1.8275519e-01, 1.8299709e-01], [2.2036446e-02, 2.2283874e-02, 2.2595389e-02, ..., 1.8251310e-01, 1.8275519e-01, 1.8299709e-01]]]], dtype=float32)
- sw_flux_dn_ref(time, NC, sample, lev)float32132.0 135.4 139.5 ... 0.6187 0.6207
- units :
- W m-2
- long_name :
- Shortwave downwelling flux of reference column
array([[[[1.3195872e+02, 1.3537129e+02, 1.3946521e+02, ..., 2.3650829e+02, 2.3651221e+02, 2.3651843e+02], [1.3195872e+02, 1.3537129e+02, 1.3946521e+02, ..., 2.3650829e+02, 2.3651221e+02, 2.3651843e+02], [1.3195872e+02, 1.3537129e+02, 1.3946521e+02, ..., 2.3650829e+02, 2.3651221e+02, 2.3651843e+02]], [[1.3195872e+02, 1.3537129e+02, 1.3946521e+02, ..., 2.3650829e+02, 2.3651221e+02, 2.3651843e+02], [1.3195872e+02, 1.3537129e+02, 1.3946521e+02, ..., 2.3650829e+02, 2.3651221e+02, 2.3651843e+02], [1.3195872e+02, 1.3537129e+02, 1.3946521e+02, ..., 2.3650829e+02, 2.3651221e+02, 2.3651843e+02]], [[1.3195872e+02, 1.3537129e+02, 1.3946521e+02, ..., 2.3650829e+02, 2.3651221e+02, 2.3651843e+02], [1.3195872e+02, 1.3537129e+02, 1.3946521e+02, ..., 2.3650829e+02, 2.3651221e+02, 2.3651843e+02], [1.3195872e+02, 1.3537129e+02, 1.3946521e+02, ..., 2.3650829e+02, 2.3651221e+02, 2.3651843e+02]], ... [[1.2533219e-01, 1.2690376e-01, 1.2873481e-01, ..., 6.1719519e-01, 6.1869735e-01, 6.2071377e-01], [1.2533219e-01, 1.2690376e-01, 1.2873481e-01, ..., 6.1719519e-01, 6.1869735e-01, 6.2071377e-01], [1.2533219e-01, 1.2690376e-01, 1.2873481e-01, ..., 6.1719519e-01, 6.1869735e-01, 6.2071377e-01]], [[1.2533219e-01, 1.2690376e-01, 1.2873481e-01, ..., 6.1719519e-01, 6.1869735e-01, 6.2071377e-01], [1.2533219e-01, 1.2690376e-01, 1.2873481e-01, ..., 6.1719519e-01, 6.1869735e-01, 6.2071377e-01], [1.2533219e-01, 1.2690376e-01, 1.2873481e-01, ..., 6.1719519e-01, 6.1869735e-01, 6.2071377e-01]], [[1.2533219e-01, 1.2690376e-01, 1.2873481e-01, ..., 6.1719519e-01, 6.1869735e-01, 6.2071377e-01], [1.2533219e-01, 1.2690376e-01, 1.2873481e-01, ..., 6.1719519e-01, 6.1869735e-01, 6.2071377e-01], [1.2533219e-01, 1.2690376e-01, 1.2873481e-01, ..., 6.1719519e-01, 6.1869735e-01, 6.2071377e-01]]]], dtype=float32)
- sw_flux_dn_dir_ref(time, NC, sample, lev)float32105.2 109.1 113.8 ... 0.6185 0.6207
- units :
- W m-2
- long_name :
- Shortwave direct downwelling flux of reference column
array([[[[1.05154472e+02, 1.09057487e+02, 1.13751366e+02, ..., 2.36507736e+02, 2.36511948e+02, 2.36518433e+02], [1.05154472e+02, 1.09057487e+02, 1.13751366e+02, ..., 2.36507736e+02, 2.36511948e+02, 2.36518433e+02], [1.05154472e+02, 1.09057487e+02, 1.13751366e+02, ..., 2.36507736e+02, 2.36511948e+02, 2.36518433e+02]], [[1.05154472e+02, 1.09057487e+02, 1.13751366e+02, ..., 2.36507736e+02, 2.36511948e+02, 2.36518433e+02], [1.05154472e+02, 1.09057487e+02, 1.13751366e+02, ..., 2.36507736e+02, 2.36511948e+02, 2.36518433e+02], [1.05154472e+02, 1.09057487e+02, 1.13751366e+02, ..., 2.36507736e+02, 2.36511948e+02, 2.36518433e+02]], [[1.05154472e+02, 1.09057487e+02, 1.13751366e+02, ..., 2.36507736e+02, 2.36511948e+02, 2.36518433e+02], [1.05154472e+02, 1.09057487e+02, 1.13751366e+02, ..., 2.36507736e+02, 2.36511948e+02, 2.36518433e+02], [1.05154472e+02, 1.09057487e+02, 1.13751366e+02, ..., 2.36507736e+02, 2.36511948e+02, 2.36518433e+02]], ... 6.16711199e-01, 6.18455350e-01, 6.20713770e-01], [3.12444676e-21, 1.64182313e-18, 1.10723977e-15, ..., 6.16711199e-01, 6.18455350e-01, 6.20713770e-01], [3.12444676e-21, 1.64182313e-18, 1.10723977e-15, ..., 6.16711199e-01, 6.18455350e-01, 6.20713770e-01]], [[3.12444676e-21, 1.64182313e-18, 1.10723977e-15, ..., 6.16711199e-01, 6.18455350e-01, 6.20713770e-01], [3.12444676e-21, 1.64182313e-18, 1.10723977e-15, ..., 6.16711199e-01, 6.18455350e-01, 6.20713770e-01], [3.12444676e-21, 1.64182313e-18, 1.10723977e-15, ..., 6.16711199e-01, 6.18455350e-01, 6.20713770e-01]], [[3.12444676e-21, 1.64182313e-18, 1.10723977e-15, ..., 6.16711199e-01, 6.18455350e-01, 6.20713770e-01], [3.12444676e-21, 1.64182313e-18, 1.10723977e-15, ..., 6.16711199e-01, 6.18455350e-01, 6.20713770e-01], [3.12444676e-21, 1.64182313e-18, 1.10723977e-15, ..., 6.16711199e-01, 6.18455350e-01, 6.20713770e-01]]]], dtype=float32)
- lw_flux_up_ref(time, NC, sample, lev)float32476.5 465.4 447.4 ... 282.1 282.1
- units :
- W m-2
- long_name :
- Longwave upwelling flux of reference column
array([[[[476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072]], [[476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072]], [[476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072]], ... [[476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072]], [[476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072]], [[476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072]]]], dtype=float32)
- lw_flux_dn_ref(time, NC, sample, lev)float32386.1 359.6 329.6 ... 0.0216 0.0
- units :
- W m-2
- long_name :
- Longwave downwelling flux of reference column
array([[[[3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00]], [[3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00]], [[3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00]], ... [[3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00]], [[3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00]], [[3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00]]]], dtype=float32)
- sza(time, NC, sample)float321.39 1.39 1.39 ... 1.743 1.743
- units :
- rad
- long_name :
- solar zenith angle
array([[[1.3903567 , 1.3903567 , 1.3903567 ], [1.3903567 , 1.3903567 , 1.3903567 ], [1.3903567 , 1.3903567 , 1.3903567 ], [1.3903567 , 1.3903567 , 1.3903567 ], [1.3903567 , 1.3903567 , 1.3903567 ], [1.3903567 , 1.3903567 , 1.3903567 ], [1.3903567 , 1.3903567 , 1.3903567 ]], [[1.2909904 , 1.2909904 , 1.2909904 ], [1.2909904 , 1.2909904 , 1.2909904 ], [1.2909904 , 1.2909904 , 1.2909904 ], [1.2909904 , 1.2909904 , 1.2909904 ], [1.2909904 , 1.2909904 , 1.2909904 ], [1.2909904 , 1.2909904 , 1.2909904 ], [1.2909904 , 1.2909904 , 1.2909904 ]], [[1.1894778 , 1.1894778 , 1.1894778 ], [1.1894778 , 1.1894778 , 1.1894778 ], [1.1894778 , 1.1894778 , 1.1894778 ], [1.1894778 , 1.1894778 , 1.1894778 ], ... [1.5686334 , 1.5686334 , 1.5686334 ], [1.5686334 , 1.5686334 , 1.5686334 ], [1.5686334 , 1.5686334 , 1.5686334 ], [1.5686334 , 1.5686334 , 1.5686334 ]], [[1.6583372 , 1.6583372 , 1.6583372 ], [1.6583372 , 1.6583372 , 1.6583372 ], [1.6583372 , 1.6583372 , 1.6583372 ], [1.6583372 , 1.6583372 , 1.6583372 ], [1.6583372 , 1.6583372 , 1.6583372 ], [1.6583372 , 1.6583372 , 1.6583372 ], [1.6583372 , 1.6583372 , 1.6583372 ]], [[1.7431656 , 1.7431656 , 1.7431656 ], [1.7431656 , 1.7431656 , 1.7431656 ], [1.7431656 , 1.7431656 , 1.7431656 ], [1.7431656 , 1.7431656 , 1.7431656 ], [1.7431656 , 1.7431656 , 1.7431656 ], [1.7431656 , 1.7431656 , 1.7431656 ], [1.7431656 , 1.7431656 , 1.7431656 ]]], dtype=float32)
- sw_flux_dn_toa(time, NC, sample)float32236.5 236.5 236.5 ... 0.6207 0.6207
- units :
- W m-2
- long_name :
- shortwave downwelling flux at toa
array([[[2.36518433e+02, 2.36518433e+02, 2.36518433e+02], [2.36518433e+02, 2.36518433e+02, 2.36518433e+02], [2.36518433e+02, 2.36518433e+02, 2.36518433e+02], [2.36518433e+02, 2.36518433e+02, 2.36518433e+02], [2.36518433e+02, 2.36518433e+02, 2.36518433e+02], [2.36518433e+02, 2.36518433e+02, 2.36518433e+02], [2.36518433e+02, 2.36518433e+02, 2.36518433e+02]], [[3.63963165e+02, 3.63963165e+02, 3.63963165e+02], [3.63963165e+02, 3.63963165e+02, 3.63963165e+02], [3.63963165e+02, 3.63963165e+02, 3.63963165e+02], [3.63963165e+02, 3.63963165e+02, 3.63963165e+02], [3.63963165e+02, 3.63963165e+02, 3.63963165e+02], [3.63963165e+02, 3.63963165e+02, 3.63963165e+02], [3.63963165e+02, 3.63963165e+02, 3.63963165e+02]], [[4.90439331e+02, 4.90439331e+02, 4.90439331e+02], [4.90439331e+02, 4.90439331e+02, 4.90439331e+02], [4.90439331e+02, 4.90439331e+02, 4.90439331e+02], [4.90439331e+02, 4.90439331e+02, 4.90439331e+02], ... [1.68698406e+01, 1.68698406e+01, 1.68698406e+01], [1.68698406e+01, 1.68698406e+01, 1.68698406e+01], [1.68698406e+01, 1.68698406e+01, 1.68698406e+01], [1.68698406e+01, 1.68698406e+01, 1.68698406e+01]], [[6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01]], [[6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01]]], dtype=float32)
- zPandasIndex
PandasIndex(Float64Index([ 10.0, 21.0, 33.0, 46.0, 60.0, 75.0, 92.0, 111.0, 132.0, 155.0, ... 3620.0, 3660.0, 3700.0, 3740.0, 3780.0, 3820.0, 3860.0, 3900.0, 3940.0, 3980.0], dtype='float64', name='z', length=107))
- zhPandasIndex
PandasIndex(Float64Index([ 0.0, 15.5, 27.0, 39.5, 53.0, 67.5, 83.5, 101.5, 121.5, 143.5, ... 3640.0, 3680.0, 3720.0, 3760.0, 3800.0, 3840.0, 3880.0, 3920.0, 3960.0, 4000.0], dtype='float64', name='zh', length=108))
- zsPandasIndex
PandasIndex(Float64Index([ -1.8899999856948853, -0.7200000286102295, -0.20999999344348907, -0.07000000029802322], dtype='float64', name='zs'))
- zshPandasIndex
PandasIndex(Float64Index([ -2.619999885559082, -1.1600000858306885, -0.2799999713897705, -0.14000000059604645, 0.0], dtype='float64', name='zsh'))
- samplePandasIndex
PandasIndex(Index(['default', 'ql', 'qlcore'], dtype='object', name='sample'))
- NCPandasIndex
PandasIndex(Int64Index([10, 50, 80, 100, 200, 400, 800], dtype='int64', name='NC'))
- timePandasIndex
PandasIndex(DatetimeIndex(['2016-06-11 12:00:00', '2016-06-11 12:30:00', '2016-06-11 13:00:00', '2016-06-11 13:30:00', '2016-06-11 14:00:00', '2016-06-11 14:30:00', '2016-06-11 15:00:00', '2016-06-11 15:30:00', '2016-06-11 16:00:00', '2016-06-11 16:30:00', '2016-06-11 17:00:00', '2016-06-11 17:30:00', '2016-06-11 18:00:00', '2016-06-11 18:30:00', '2016-06-11 19:00:00', '2016-06-11 19:30:00', '2016-06-11 20:00:00', '2016-06-11 20:30:00', '2016-06-11 21:00:00', '2016-06-11 21:30:00', '2016-06-11 22:00:00', '2016-06-11 22:30:00', '2016-06-11 23:00:00', '2016-06-11 23:30:00', '2016-06-12 00:00:00', '2016-06-12 00:30:00', '2016-06-12 01:00:00', '2016-06-12 01:30:00', '2016-06-12 02:00:00', '2016-06-12 02:30:00'], dtype='datetime64[ns]', name='time', freq='30T'))
# We first explore a few basic statistics, such as overall cloud cover, LWP, cloud base height, and cloud top height
plt.figure()
prof.sel(NC=100, sample='default')['ql_cover'].plot()
plt.figure()
prof.sel(NC=100, sample='default')['ql_path'].plot()
plt.figure()
prof.sel(NC=100, sample='default')['qr_path'].plot()
[<matplotlib.lines.Line2D at 0x7f7fc0ca8e80>]
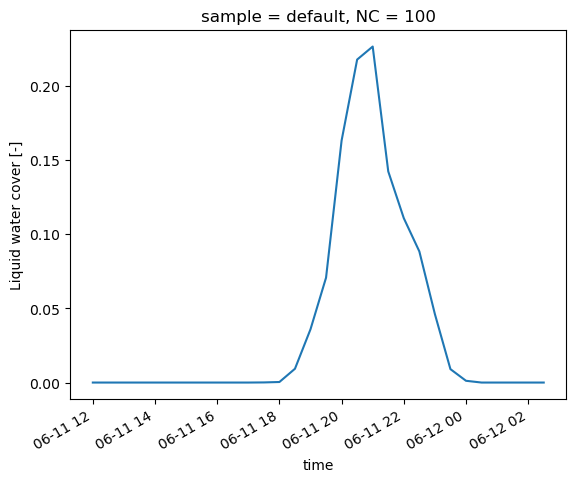
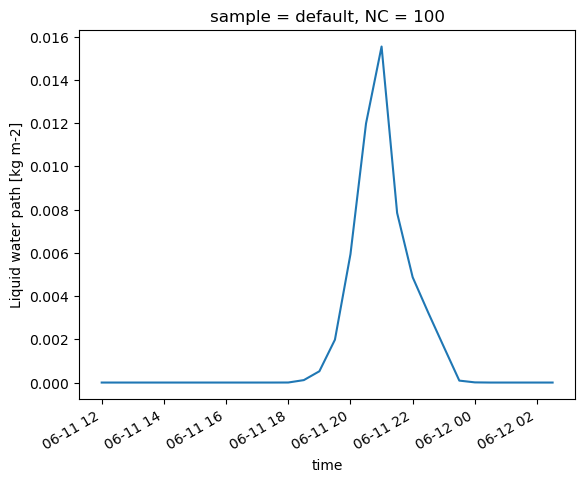
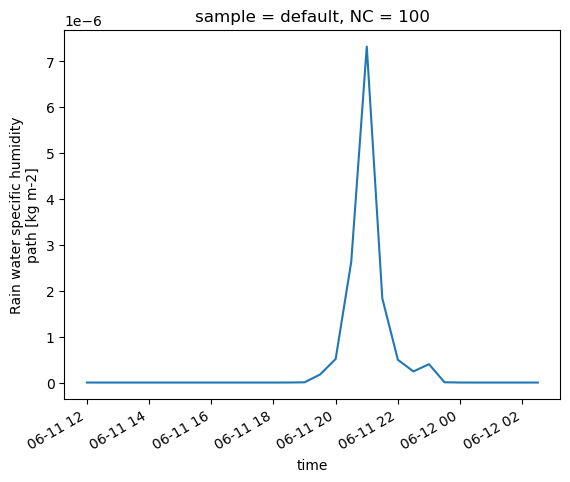
prof.sel(NC=100, sample='default')['ql'].where(prof.sel(NC=100, sample='default').ql>0).plot(x='time')
<matplotlib.collections.QuadMesh at 0x7f7fc0b5bd30>
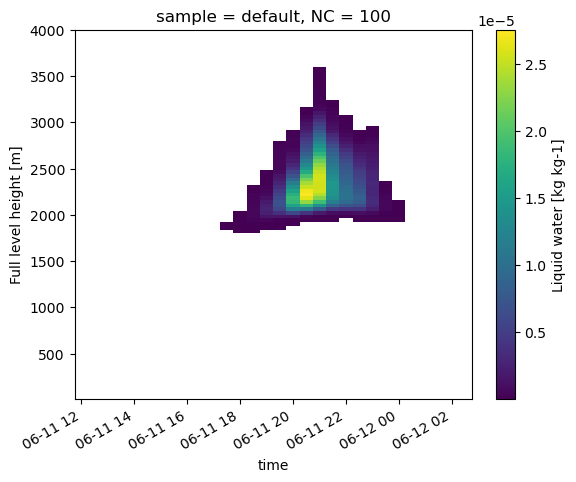
#At 22:00, we are at peak cloudiness. Let's explore the vertical structure of the cloud at this time
plt.figure()
prof.sel(time='2016-06-11T22:00', NC=100).thl.plot.line(y='z', hue='sample')
plt.figure()
prof.sel(time='2016-06-11T22:00', NC=100).qt.plot.line(y='z', hue='sample')
plt.figure()
prof.sel(time='2016-06-11T22:00', NC=100).w.plot.line(y='zh', hue='sample')
[<matplotlib.lines.Line2D at 0x7f7fc0ad2b60>,
<matplotlib.lines.Line2D at 0x7f7fc0ad2b00>,
<matplotlib.lines.Line2D at 0x7f7fc0ad2d10>]
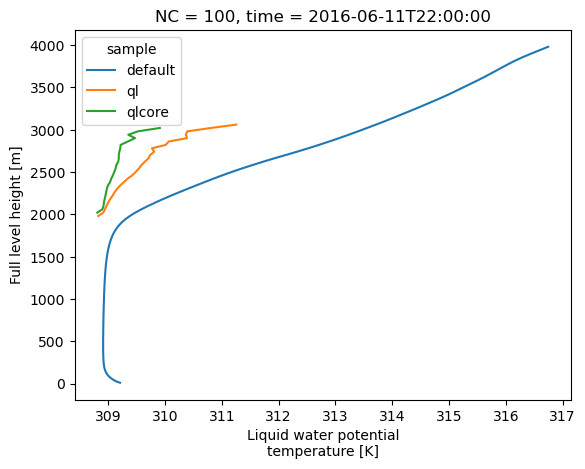
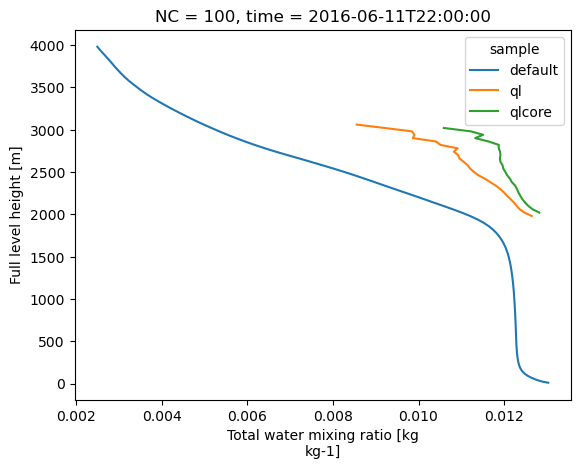
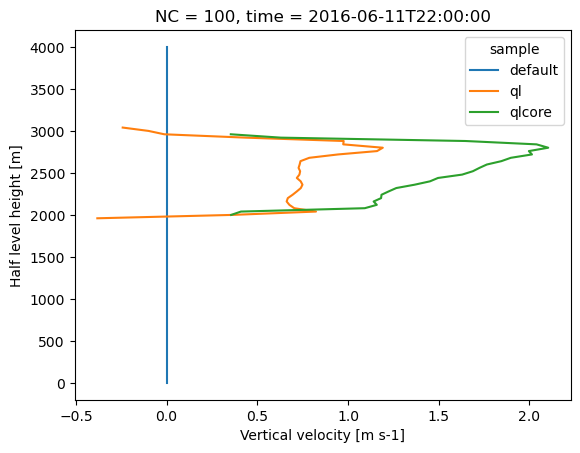
#Now for the moisture flux: Is this correct??
prof.sel(sample='default', NC=100, time="2016-06-11T22:00").qt_w.plot(y='zh')
#We should not forget to add the Sub Filter Scale Fluxes; the MicroHH output has that automatically as '_flux'. What do you notice about the following plot?
prof.sel(sample='default', NC=100, time="2016-06-11T22:00").qt_w.plot(y='zh')
prof.sel(sample='default', NC=100, time="2016-06-11T22:00").qt_diff.plot(y='zh')
prof.sel(sample='default', NC=100, time="2016-06-11T22:00").qt_flux.plot(y='zh')
[<matplotlib.lines.Line2D at 0x7f7fc05f63e0>]
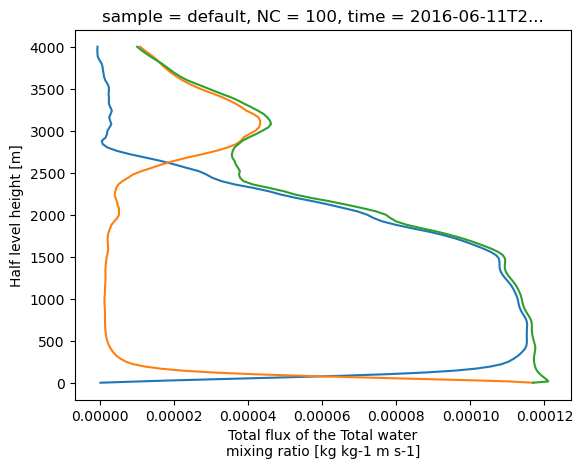
# Calculate the cloud base and cloud top heights, that is, the first and last point where the liquid water content is larger than zero
prof['cb'] = (prof['ql']>0).idxmax(dim='z')
prof['cb'] = prof.cb.where(prof.cb>prof.z[0]) # remove cloud base if they are at the lowest level
prof['ct'] = (prof['ql']>0).isel(z=slice(None, None, -1)).idxmax(dim='z')
prof['ct'] = prof.ct.where(prof.ct<prof.z[-1]) # remove cloud top if they are at the highest level
# Plot them
prof.sel(sample='default')['cb'].plot.line(hue='NC')
prof.sel(sample='default')['ct'].plot.line(hue='NC')
# prof.sel(sample='default')['ct'].plot()
[<matplotlib.lines.Line2D at 0x7f7fc065b310>,
<matplotlib.lines.Line2D at 0x7f7fc065b340>,
<matplotlib.lines.Line2D at 0x7f7fc065b0d0>,
<matplotlib.lines.Line2D at 0x7f7fc062dde0>,
<matplotlib.lines.Line2D at 0x7f7fc065ad10>,
<matplotlib.lines.Line2D at 0x7f7fc0659300>,
<matplotlib.lines.Line2D at 0x7f7fc0659f30>]
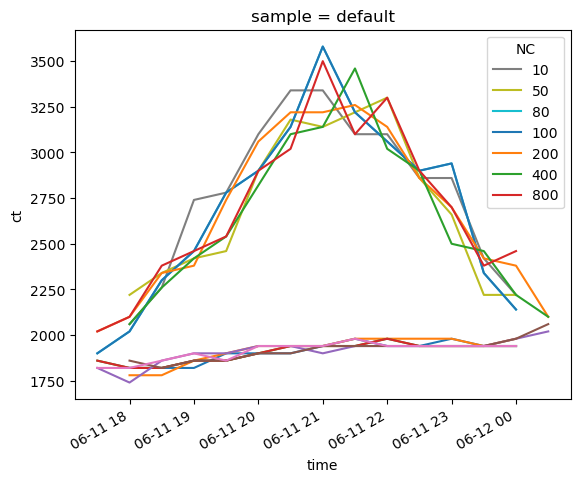
prof.sel(sample='ql').ql.plot(x='time', col='NC', col_wrap=4)
<xarray.plot.facetgrid.FacetGrid at 0x7f7fc5afbe50>
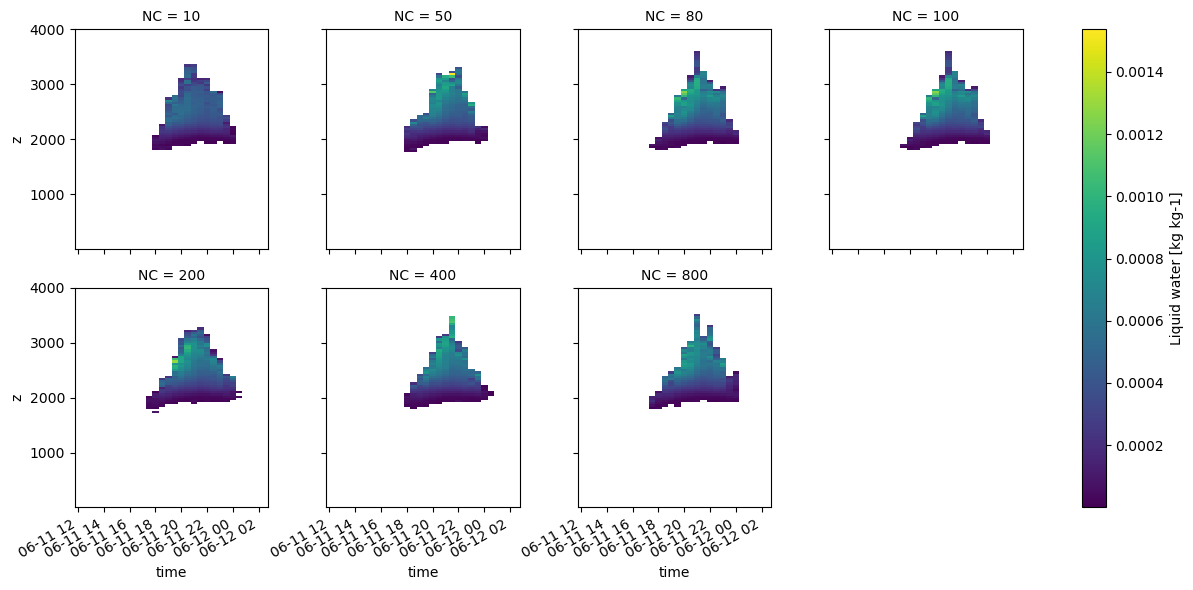
(-prof.sel(sample='default').qr_flux.sel(zh=0).cumsum()).plot(x='time', hue='NC')
[<matplotlib.lines.Line2D at 0x7f7fc10fbfa0>,
<matplotlib.lines.Line2D at 0x7f7fc0f21030>,
<matplotlib.lines.Line2D at 0x7f7fc0f20ee0>,
<matplotlib.lines.Line2D at 0x7f7fc0f20e20>,
<matplotlib.lines.Line2D at 0x7f7fc10bb8e0>,
<matplotlib.lines.Line2D at 0x7f7fc10bb910>,
<matplotlib.lines.Line2D at 0x7f7fc0f21210>]
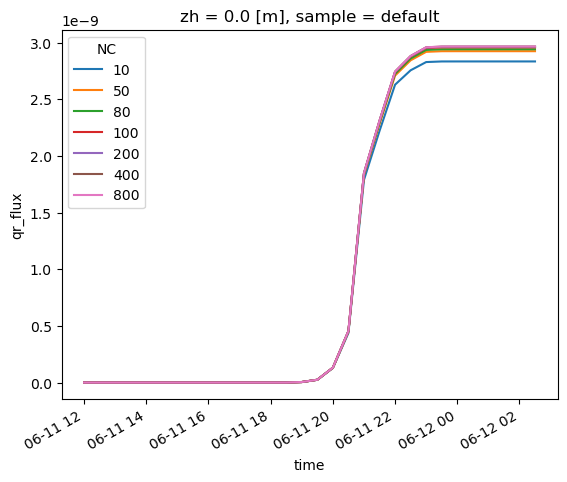
#Bulk entrainment is defined as \epsilon = -\frac{d\theta_l}{dz} / (\phi_c - \phi_e), where \phi_c is the cloud property, and \phi_e are the environmental properties.
#We will use the cloud potential temperature as the cloud property, and the environmental potential temperature as the environmental property
epsilon = prof.sel(time='2016-06-11T22:00', sample='ql').thl.differentiate(coord='z') / (prof.sel(time='2016-06-11T22:00', sample='default').thl - prof.sel(time='2016-06-11T22:00', sample='ql').thl)
epsilon.plot.line(y='z', hue='NC')
[<matplotlib.lines.Line2D at 0x7f7fc06c6e90>,
<matplotlib.lines.Line2D at 0x7f7fc06c6e30>,
<matplotlib.lines.Line2D at 0x7f7fc06c7040>,
<matplotlib.lines.Line2D at 0x7f7fc06c7160>,
<matplotlib.lines.Line2D at 0x7f7fc06c7280>,
<matplotlib.lines.Line2D at 0x7f7fc06c73a0>,
<matplotlib.lines.Line2D at 0x7f7fc06c74c0>]
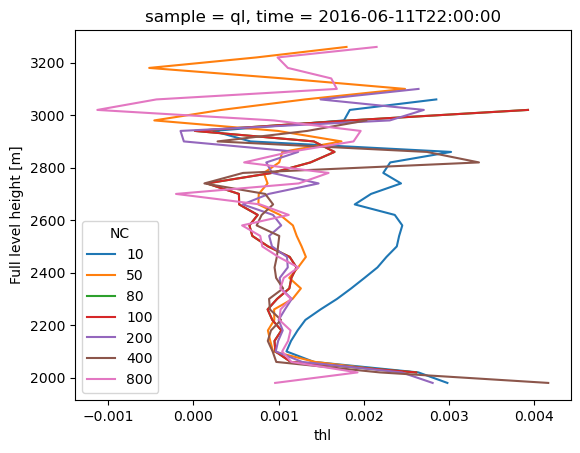
prof
<xarray.Dataset> Dimensions: (z: 107, zh: 108, zs: 4, zsh: 5, sample: 3, NC: 8, time: 30, lev: 141) Coordinates: * z (z) float32 10.0 21.0 33.0 ... 3.94e+03 3.98e+03 * zh (zh) float32 0.0 15.5 27.0 ... 3.92e+03 3.96e+03 4e+03 * zs (zs) float32 -1.89 -0.72 -0.21 -0.07 * zsh (zsh) float32 -2.62 -1.16 -0.28 -0.14 0.0 * sample (sample) object 'default' 'ql' 'qlcore' * NC (NC) int64 10 50 80 100 200 400 600 800 * time (time) datetime64[ns] 2016-06-11T12:00:00 ... 2016-... Dimensions without coordinates: lev Data variables: (12/141) p_rad (time, NC, sample, lev) float32 9.645e+04 ... 3.53 iter (time, NC, sample) float64 1.738e+05 ... 2.484e+05 area (time, NC, sample, z) float32 1.0 1.0 1.0 ... 0.0 0.0 areah (time, NC, sample, zh) float32 1.0 1.0 1.0 ... 0.0 0.0 u (time, NC, sample, z) float32 -0.09499 ... nan u_3 (time, NC, sample, z) float32 -0.001409 ... nan ... ... sw_flux_dn_ref (time, NC, sample, lev) float32 132.0 135.4 ... 0.6207 sw_flux_dn_dir_ref (time, NC, sample, lev) float32 105.2 109.1 ... 0.6207 lw_flux_up_ref (time, NC, sample, lev) float32 476.5 465.4 ... 282.1 lw_flux_dn_ref (time, NC, sample, lev) float32 386.1 359.6 ... 0.0 sza (time, NC, sample) float32 1.39 1.39 ... 1.743 1.743 sw_flux_dn_toa (time, NC, sample) float32 236.5 236.5 ... 0.6207
xarray.Dataset
- z: 107
- zh: 108
- zs: 4
- zsh: 5
- sample: 3
- NC: 8
- time: 30
- lev: 141
- z(z)float3210.0 21.0 ... 3.94e+03 3.98e+03
- units :
- m
- long_name :
- Full level height
array([ 10., 21., 33., 46., 60., 75., 92., 111., 132., 155., 180., 207., 237., 267., 300., 340., 380., 420., 460., 500., 540., 580., 620., 660., 700., 740., 780., 820., 860., 900., 940., 980., 1020., 1060., 1100., 1140., 1180., 1220., 1260., 1300., 1340., 1380., 1420., 1460., 1500., 1540., 1580., 1620., 1660., 1700., 1740., 1780., 1820., 1860., 1900., 1940., 1980., 2020., 2060., 2100., 2140., 2180., 2220., 2260., 2300., 2340., 2380., 2420., 2460., 2500., 2540., 2580., 2620., 2660., 2700., 2740., 2780., 2820., 2860., 2900., 2940., 2980., 3020., 3060., 3100., 3140., 3180., 3220., 3260., 3300., 3340., 3380., 3420., 3460., 3500., 3540., 3580., 3620., 3660., 3700., 3740., 3780., 3820., 3860., 3900., 3940., 3980.], dtype=float32)
- zh(zh)float320.0 15.5 27.0 ... 3.96e+03 4e+03
- units :
- m
- long_name :
- Half level height
array([ 0. , 15.5, 27. , 39.5, 53. , 67.5, 83.5, 101.5, 121.5, 143.5, 167.5, 193.5, 222. , 252. , 283.5, 320. , 360. , 400. , 440. , 480. , 520. , 560. , 600. , 640. , 680. , 720. , 760. , 800. , 840. , 880. , 920. , 960. , 1000. , 1040. , 1080. , 1120. , 1160. , 1200. , 1240. , 1280. , 1320. , 1360. , 1400. , 1440. , 1480. , 1520. , 1560. , 1600. , 1640. , 1680. , 1720. , 1760. , 1800. , 1840. , 1880. , 1920. , 1960. , 2000. , 2040. , 2080. , 2120. , 2160. , 2200. , 2240. , 2280. , 2320. , 2360. , 2400. , 2440. , 2480. , 2520. , 2560. , 2600. , 2640. , 2680. , 2720. , 2760. , 2800. , 2840. , 2880. , 2920. , 2960. , 3000. , 3040. , 3080. , 3120. , 3160. , 3200. , 3240. , 3280. , 3320. , 3360. , 3400. , 3440. , 3480. , 3520. , 3560. , 3600. , 3640. , 3680. , 3720. , 3760. , 3800. , 3840. , 3880. , 3920. , 3960. , 4000. ], dtype=float32)
- zs(zs)float32-1.89 -0.72 -0.21 -0.07
- units :
- m
- long_name :
- Full level height soil
array([-1.89, -0.72, -0.21, -0.07], dtype=float32)
- zsh(zsh)float32-2.62 -1.16 -0.28 -0.14 0.0
- units :
- m
- long_name :
- Half level height soil
array([-2.62, -1.16, -0.28, -0.14, 0. ], dtype=float32)
- sample(sample)object'default' 'ql' 'qlcore'
array(['default', 'ql', 'qlcore'], dtype=object)
- NC(NC)int6410 50 80 100 200 400 600 800
array([ 10, 50, 80, 100, 200, 400, 600, 800])
- time(time)datetime64[ns]2016-06-11T12:00:00 ... 2016-06-...
array(['2016-06-11T12:00:00.000000000', '2016-06-11T12:30:00.000000000', '2016-06-11T13:00:00.000000000', '2016-06-11T13:30:00.000000000', '2016-06-11T14:00:00.000000000', '2016-06-11T14:30:00.000000000', '2016-06-11T15:00:00.000000000', '2016-06-11T15:30:00.000000000', '2016-06-11T16:00:00.000000000', '2016-06-11T16:30:00.000000000', '2016-06-11T17:00:00.000000000', '2016-06-11T17:30:00.000000000', '2016-06-11T18:00:00.000000000', '2016-06-11T18:30:00.000000000', '2016-06-11T19:00:00.000000000', '2016-06-11T19:30:00.000000000', '2016-06-11T20:00:00.000000000', '2016-06-11T20:30:00.000000000', '2016-06-11T21:00:00.000000000', '2016-06-11T21:30:00.000000000', '2016-06-11T22:00:00.000000000', '2016-06-11T22:30:00.000000000', '2016-06-11T23:00:00.000000000', '2016-06-11T23:30:00.000000000', '2016-06-12T00:00:00.000000000', '2016-06-12T00:30:00.000000000', '2016-06-12T01:00:00.000000000', '2016-06-12T01:30:00.000000000', '2016-06-12T02:00:00.000000000', '2016-06-12T02:30:00.000000000'], dtype='datetime64[ns]')
- p_rad(time, NC, sample, lev)float329.645e+04 9.188e+04 ... 3.871 3.53
- units :
- Pa
- long_name :
- Pressure of radiation reference column
array([[[[9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00]], [[9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00]], [[9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00]], ... [[9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00]], [[9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00]], [[9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00], [9.6446820e+04, 9.1882547e+04, 8.6768148e+04, ..., 4.2274017e+00, 3.8710907e+00, 3.5295606e+00]]]], dtype=float32)
- iter(time, NC, sample)float641.738e+05 1.738e+05 ... 2.484e+05
- units :
- -
- long_name :
- Iteration number
array([[[173824.83333333, 173824.83333333, 173824.83333333], [173710. , 173710. , 173710. ], [171538. , 171538. , 171538. ], [171538. , 171538. , 171538. ], [173173.5 , 173173.5 , 173173.5 ], [173498.16666667, 173498.16666667, 173498.16666667], [172095.5 , 172095.5 , 172095.5 ], [171815.16666667, 171815.16666667, 171815.16666667]], [[176302.16666667, 176302.16666667, 176302.16666667], [176024.66666667, 176024.66666667, 176024.66666667], [173800.5 , 173800.5 , 173800.5 ], [173800.5 , 173800.5 , 173800.5 ], [175536. , 175536. , 175536. ], [175847.66666667, 175847.66666667, 175847.66666667], [174439. , 174439. , 174439. ], [174150. , 174150. , 174150. ]], [[178840.33333333, 178840.33333333, 178840.33333333], [178431.16666667, 178431.16666667, 178431.16666667], ... [ nan, nan, nan], [245470.83333333, 245470.83333333, 245470.83333333]], [[249770.5 , 249770.5 , 249770.5 ], [249189.16666667, 249189.16666667, 249189.16666667], [245713.83333333, 245713.83333333, 245713.83333333], [245713.83333333, 245713.83333333, 245713.83333333], [248669.5 , 248669.5 , 248669.5 ], [248487.16666667, 248487.16666667, 248487.16666667], [ nan, nan, nan], [246900.5 , 246900.5 , 246900.5 ]], [[251346.16666667, 251346.16666667, 251346.16666667], [250717.33333333, 250717.33333333, 250717.33333333], [247236.16666667, 247236.16666667, 247236.16666667], [247236.16666667, 247236.16666667, 247236.16666667], [250206.66666667, 250206.66666667, 250206.66666667], [250015.33333333, 250015.33333333, 250015.33333333], [ nan, nan, nan], [248411.16666667, 248411.16666667, 248411.16666667]]])
- area(time, NC, sample, z)float321.0 1.0 1.0 1.0 ... 0.0 0.0 0.0 0.0
- units :
- -
- long_name :
- Fractional area contained in mask
array([[[[ 1., 1., 1., ..., 1., 1., 1.], [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.]], [[ 1., 1., 1., ..., 1., 1., 1.], [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.]], [[ 1., 1., 1., ..., 1., 1., 1.], [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.]], ..., [[ 1., 1., 1., ..., 1., 1., 1.], [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.]], [[ 1., 1., 1., ..., 1., 1., 1.], [ 0., 0., 0., ..., 0., 0., 0.], ... [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.]], [[ 1., 1., 1., ..., 1., 1., 1.], [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.]], ..., [[ 1., 1., 1., ..., 1., 1., 1.], [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.]], [[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 1., 1., 1., ..., 1., 1., 1.], [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.]]]], dtype=float32)
- areah(time, NC, sample, zh)float321.0 1.0 1.0 1.0 ... 0.0 0.0 0.0 0.0
- units :
- -
- long_name :
- Fractional area contained in mask
array([[[[ 1., 1., 1., ..., 1., 1., 1.], [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.]], [[ 1., 1., 1., ..., 1., 1., 1.], [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.]], [[ 1., 1., 1., ..., 1., 1., 1.], [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.]], ..., [[ 1., 1., 1., ..., 1., 1., 1.], [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.]], [[ 1., 1., 1., ..., 1., 1., 1.], [ 0., 0., 0., ..., 0., 0., 0.], ... [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.]], [[ 1., 1., 1., ..., 1., 1., 1.], [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.]], ..., [[ 1., 1., 1., ..., 1., 1., 1.], [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.]], [[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 1., 1., 1., ..., 1., 1., 1.], [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.]]]], dtype=float32)
- u(time, NC, sample, z)float32-0.09499 -0.09687 ... nan nan
- units :
- m s-1
- long_name :
- U velocity
array([[[[-9.49917212e-02, -9.68650654e-02, -8.95264447e-02, ..., -1.30459642e+01, -1.31258478e+01, -1.31902342e+01], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-1.67487394e-02, 5.63607842e-04, 2.34647561e-02, ..., -1.30383606e+01, -1.31147003e+01, -1.31708059e+01], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 5.64690344e-02, 9.15815011e-02, 1.28741562e-01, ..., -1.30466280e+01, -1.31305094e+01, -1.31981239e+01], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -1.46103466e+00, -1.93254316e+00, -2.41721559e+00], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-2.08752060e+00, -2.48598742e+00, -2.80993962e+00, ..., -1.50068474e+00, -1.97253859e+00, -2.45660615e+00], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- u_3(time, NC, sample, z)float32-0.001409 -0.0009854 ... nan nan
- units :
- m3 s-3
- long_name :
- Moment 3 of the U velocity
array([[[[-1.40902773e-03, -9.85385966e-04, -1.59479052e-04, ..., 2.62038526e-03, 1.91816140e-03, 9.36055323e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 1.43977255e-03, 2.87981145e-03, 4.21256153e-03, ..., 2.38896420e-04, 7.26173923e-04, 1.20713946e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 1.00412208e-03, 1.59067556e-03, 2.04086746e-03, ..., -1.82128523e-03, -5.86811570e-04, -1.91333165e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -3.49943265e-02, -3.38279046e-02, -2.12429855e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 5.94858965e-03, 7.49488873e-03, 7.74336653e-03, ..., -3.00069642e-03, -2.56332080e-03, -9.12722957e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- u_4(time, NC, sample, z)float320.01919 0.03403 0.04558 ... nan nan
- units :
- m4 s-4
- long_name :
- Moment 4 of the U velocity
array([[[[0.01918945, 0.03403257, 0.04558229, ..., 0.01016053, 0.00863376, 0.0082782 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00906352, 0.01653056, 0.02278082, ..., 0.00661484, 0.00576363, 0.0057016 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00407337, 0.00726029, 0.01000305, ..., 0.01024383, 0.00929487, 0.00795281], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[0.01229064, 0.021283 , 0.02697428, ..., 0.2518671 , 0.28112355, 0.30377305], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.01996377, 0.03464783, 0.0410837 , ..., 0.28225797, 0.3465459 , 0.4004334 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- u_diff(time, NC, sample, zh)float320.004834 -0.0002237 ... nan nan
- units :
- m2 s-2
- long_name :
- Diffusive flux of the U velocity
array([[[[ 0.00483373, -0.0002237 , -0.00303758, ..., 0.00500831, 0.00447566, 0.00371043], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00026824, -0.00513147, -0.00829199, ..., 0.00512256, 0.00420302, 0.00311806], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.00356263, -0.00930617, -0.01277095, ..., 0.00589648, 0.00501473, 0.00400078], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 0.08840257, 0.08108174, 0.07588992, ..., 0.04764106, 0.04839069, 0.0486617 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.08999104, 0.08229417, 0.07678535, ..., 0.04484082, 0.04512601, 0.0462656 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- u_w(time, NC, sample, zh)float320.0 -0.000416 ... nan nan
- units :
- m2 s-2
- long_name :
- Turbulent flux of the U velocity
array([[[[ 0.00000000e+00, -4.16043913e-04, -9.52353410e-04, ..., 3.13887000e-03, 2.08051922e-03, 1.08090532e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, -2.60635716e-04, -6.17534562e-04, ..., 3.31096421e-03, 3.08820326e-03, 2.80047231e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, -2.76613369e-04, -6.60845137e-04, ..., 3.51774110e-03, 3.21261119e-03, 2.75658048e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 1.66938379e-02, 1.77665502e-02, 1.87248122e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 1.42514578e-03, 2.94300099e-03, ..., 1.76848453e-02, 1.90284271e-02, 1.90232154e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- u_grad(time, NC, sample, zh)float32-0.009499 -0.0001703 ... nan nan
- units :
- s-1
- long_name :
- Gradient of the U velocity
array([[[[-9.4991727e-03, -1.7030416e-04, 6.1155146e-04, ..., -1.9970788e-03, -1.6096552e-03, -1.1898429e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-1.6748741e-03, 1.5738503e-03, 1.9084289e-03, ..., -1.9084993e-03, -1.4026305e-03, -9.3591778e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 5.6469031e-03, 3.1920422e-03, 3.0966718e-03, ..., -2.0970355e-03, -1.6903189e-03, -1.2418026e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -1.1787713e-02, -1.2116813e-02, -1.2607314e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-2.0875205e-01, -3.6224280e-02, -2.6996009e-02, ..., -1.1796349e-02, -1.2101694e-02, -1.2382776e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- u_2(time, NC, sample, z)float320.07758 0.1033 0.1193 ... nan nan
- units :
- m2 s-2
- long_name :
- Moment 2 of the U velocity
array([[[[0.07757594, 0.10334253, 0.11932987, ..., 0.05822359, 0.05638469, 0.05637448], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.05485221, 0.07437593, 0.08756799, ..., 0.04853062, 0.04446862, 0.04261628], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.03722739, 0.04992924, 0.05872838, ..., 0.05875093, 0.05609078, 0.05301555], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[0.06079409, 0.08316822, 0.09457625, ..., 0.30183765, 0.3181478 , 0.32992613], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.07831373, 0.10593799, 0.11652273, ..., 0.31453082, 0.34970632, 0.3716754 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- u_path(time, NC, sample)float32-4.716e+03 nan nan ... nan nan
- units :
- m-1 s-1 kg
- long_name :
- U velocity path
array([[[-4715.812 , nan, nan], [-4754.7944 , nan, nan], [-4701.4146 , nan, nan], [-4701.4146 , nan, nan], [-4761.2563 , nan, nan], [-4768.281 , nan, nan], [-4746.144 , nan, nan], [-4731.2886 , nan, nan]], [[-4502.8438 , nan, nan], [-4536.906 , nan, nan], [-4475.775 , nan, nan], [-4475.775 , nan, nan], [-4532.352 , nan, nan], [-4542.9644 , nan, nan], [-4524.7446 , nan, nan], [-4498.607 , nan, nan]], [[-3741.0918 , nan, nan], [-3767.9307 , nan, nan], ... [ nan, nan, nan], [44734.082 , nan, nan]], [[41304.848 , nan, nan], [41262.37 , nan, nan], [41446.03 , nan, nan], [41446.03 , nan, nan], [41474.35 , nan, nan], [41373.203 , nan, nan], [ nan, nan, nan], [41437.78 , nan, nan]], [[37084.523 , nan, nan], [37066.5 , nan, nan], [37194.44 , nan, nan], [37194.44 , nan, nan], [37222.44 , nan, nan], [37134.44 , nan, nan], [ nan, nan, nan], [37184.668 , nan, nan]]], dtype=float32)
- u_flux(time, NC, sample, zh)float320.004834 -0.0006397 ... nan nan
- units :
- m2 s-2
- long_name :
- Total flux of the U velocity
array([[[[ 0.00483373, -0.00063975, -0.00398993, ..., 0.00814718, 0.00655618, 0.00479134], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00026824, -0.0053921 , -0.00890952, ..., 0.00843352, 0.00729122, 0.00591853], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.00356263, -0.00958278, -0.0134318 , ..., 0.00941422, 0.00822734, 0.00675736], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 0.08840257, 0.08228742, 0.07843512, ..., 0.06433491, 0.06615724, 0.06738651], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.08999104, 0.08371932, 0.07972834, ..., 0.06252567, 0.06415442, 0.06528881], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- v(time, NC, sample, z)float324.354 5.359 6.127 ... nan nan nan
- units :
- m s-1
- long_name :
- V velocity
array([[[[ 4.354159 , 5.3590884, 6.127005 , ..., 11.668595 , 12.139609 , 12.615883 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 4.307282 , 5.3010173, 6.0629115, ..., 11.610049 , 12.0808 , 12.559289 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 4.3404784, 5.3431535, 6.112883 , ..., 11.667278 , 12.140002 , 12.618789 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 3.0726795, 3.6933982, 4.2173495, ..., -19.530668 , -20.165392 , -20.789743 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 3.1292334, 3.761398 , 4.292082 , ..., -19.47424 , -20.089907 , -20.687008 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- v_3(time, NC, sample, z)float320.09091 0.1656 0.2339 ... nan nan
- units :
- m3 s-3
- long_name :
- Moment 3 of the V velocity
array([[[[ 9.09094736e-02, 1.65625244e-01, 2.33898476e-01, ..., -4.20214562e-03, -6.94942242e-03, -9.19930264e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 7.45725036e-02, 1.44534886e-01, 2.17775390e-01, ..., 9.46714543e-03, 5.61530283e-03, 2.56581022e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 3.98850851e-02, 7.11039528e-02, 9.92931947e-02, ..., 1.14700375e-02, 8.35625082e-03, 1.29812525e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -5.84175773e-02, -2.25789603e-02, -6.37704181e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-2.57698540e-03, -4.94174147e-03, -8.35200679e-03, ..., -3.55744660e-02, -1.76107455e-02, 1.75447911e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- v_4(time, NC, sample, z)float320.8115 1.85 3.014 ... nan nan nan
- units :
- m4 s-4
- long_name :
- Moment 4 of the V velocity
array([[[[8.11496735e-01, 1.84975803e+00, 3.01371121e+00, ..., 7.19377995e-02, 7.78881088e-02, 8.58658180e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[3.89646530e-01, 9.07469809e-01, 1.51135457e+00, ..., 7.03007132e-02, 6.54432550e-02, 6.27623722e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[1.18167073e-01, 2.59517938e-01, 4.14338678e-01, ..., 1.13024659e-01, 1.20911896e-01, 1.22352071e-01], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 1.37845516e+00, 1.19354188e+00, 1.13856626e+00], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[4.41932194e-02, 1.18256591e-01, 2.03074574e-01, ..., 1.31161869e+00, 1.16126144e+00, 9.71093714e-01], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- v_diff(time, NC, sample, zh)float32-0.2723 -0.2668 -0.2613 ... nan nan
- units :
- m2 s-2
- long_name :
- Diffusive flux of the V velocity
array([[[[-0.2723187 , -0.26684368, -0.26133433, ..., -0.02283219, -0.02311927, -0.0225108 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.26330158, -0.25924253, -0.25516912, ..., -0.02359941, -0.02435344, -0.02418181], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.26414326, -0.2608383 , -0.25767645, ..., -0.0237529 , -0.02484685, -0.02519906], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[-0.12980865, -0.12709188, -0.12384892, ..., 0.0711123 , 0.06792122, 0.06305306], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.13495374, -0.13168573, -0.12774041, ..., 0.06274878, 0.05928886, 0.05707845], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- v_w(time, NC, sample, zh)float320.0 -0.003076 -0.006489 ... nan nan
- units :
- m2 s-2
- long_name :
- Turbulent flux of the V velocity
array([[[[ 0. , -0.00307555, -0.00648921, ..., -0.00540954, -0.00548873, -0.00607088], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , -0.00212908, -0.00454533, ..., -0.0042045 , -0.00400367, -0.00405313], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , -0.00118444, -0.00250309, ..., -0.00531726, -0.00499956, -0.00502317], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 0. , -0.00234464, -0.00511139, ..., 0.03162116, 0.02963656, 0.02944005], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , -0.00291575, -0.00637811, ..., 0.02793687, 0.02730381, 0.02530385], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- v_grad(time, NC, sample, zh)float320.4354 0.09136 0.06399 ... nan nan
- units :
- s-1
- long_name :
- Gradient of the V velocity
array([[[[ 0.43541586, 0.09135725, 0.06399304, ..., 0.01177533, 0.01190685, 0.01195674], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.4307282 , 0.09033957, 0.06349116, ..., 0.01176882, 0.0119622 , 0.01214385], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.43404785, 0.09115225, 0.06414418, ..., 0.0118181 , 0.01196966, 0.01203723], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 0.30726793, 0.05642899, 0.04366258, ..., -0.0158681 , -0.01560878, -0.01507068], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.31292334, 0.05746952, 0.04422364, ..., -0.0153917 , -0.01492754, -0.0145243 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- v_2(time, NC, sample, z)float320.5385 0.8205 1.053 ... nan nan nan
- units :
- m2 s-2
- long_name :
- Moment 2 of the V velocity
array([[[[0.5384955 , 0.8205159 , 1.0525634 , ..., 0.15860043, 0.16706336, 0.17569675], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.39135894, 0.59841627, 0.77161473, ..., 0.14564537, 0.14447454, 0.14592505], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.19480985, 0.29185885, 0.37168288, ..., 0.1869903 , 0.19423968, 0.20012741], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[0.13425352, 0.2085383 , 0.26403055, ..., 0.7255812 , 0.6681343 , 0.6372035 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.14048027, 0.23067643, 0.30136383, ..., 0.67165256, 0.63084364, 0.5739154 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- v_path(time, NC, sample)float321.625e+04 nan nan ... nan nan
- units :
- m-1 s-1 kg
- long_name :
- V velocity path
array([[[ 1.6245251e+04, nan, nan], [ 1.6327103e+04, nan, nan], [ 1.6450805e+04, nan, nan], [ 1.6450805e+04, nan, nan], [ 1.6378855e+04, nan, nan], [ 1.6380281e+04, nan, nan], [ 1.6328070e+04, nan, nan], [ 1.6439955e+04, nan, nan]], [[ 2.0423604e+04, nan, nan], [ 2.0521787e+04, nan, nan], [ 2.0640174e+04, nan, nan], [ 2.0640174e+04, nan, nan], [ 2.0575502e+04, nan, nan], [ 2.0576330e+04, nan, nan], [ 2.0523135e+04, nan, nan], [ 2.0628928e+04, nan, nan]], [[ 2.4335977e+04, nan, nan], [ 2.4438525e+04, nan, nan], ... [ nan, nan, nan], [ 6.0980713e+02, nan, nan]], [[-3.3100574e+03, nan, nan], [-3.1599929e+03, nan, nan], [-3.4127236e+03, nan, nan], [-3.4127236e+03, nan, nan], [-3.4946768e+03, nan, nan], [-3.3898298e+03, nan, nan], [ nan, nan, nan], [-3.4215486e+03, nan, nan]], [[-7.0398613e+03, nan, nan], [-6.8704263e+03, nan, nan], [-7.1494868e+03, nan, nan], [-7.1494868e+03, nan, nan], [-7.2409648e+03, nan, nan], [-7.1154976e+03, nan, nan], [ nan, nan, nan], [-7.1654790e+03, nan, nan]]], dtype=float32)
- v_flux(time, NC, sample, zh)float32-0.2723 -0.2699 -0.2678 ... nan nan
- units :
- m2 s-2
- long_name :
- Total flux of the V velocity
array([[[[-0.2723187 , -0.26991922, -0.26782352, ..., -0.02824172, -0.02860799, -0.02858169], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.26330158, -0.26137164, -0.25971445, ..., -0.02780391, -0.02835711, -0.02823495], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.26414326, -0.26202273, -0.26017955, ..., -0.02907016, -0.02984641, -0.03022222], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[-0.12980865, -0.12943651, -0.12896031, ..., 0.10273346, 0.09755778, 0.09249311], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.13495374, -0.1346015 , -0.13411851, ..., 0.09068566, 0.08659267, 0.0823823 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- w(time, NC, sample, zh)float320.0 1.16e-10 1.775e-10 ... nan nan
- units :
- m s-1
- long_name :
- Vertical velocity
array([[[[ 0.00000000e+00, 1.15976194e-10, 1.77543605e-10, ..., -6.43586684e-10, -6.70604905e-10, -6.13613771e-10], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 1.20320087e-10, 1.70441050e-10, ..., -4.28033692e-10, -4.29768859e-10, -4.59531885e-10], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 1.30374656e-10, 4.05349893e-10, ..., -5.25977761e-10, -4.81743478e-10, -5.50631041e-10], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -1.37780243e-09, -1.54771840e-09, -1.47588708e-09], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 2.68065098e-10, 5.87139670e-10, ..., -9.50123091e-10, -1.01147857e-09, -9.78468084e-10], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- w_3(time, NC, sample, zh)float320.0 -9.067e-07 ... nan nan
- units :
- m3 s-3
- long_name :
- Moment 3 of the Vertical velocity
array([[[[ 0.00000000e+00, -9.06703065e-07, -4.93891685e-06, ..., 2.80323817e-04, 2.41136935e-04, 1.95793502e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, -3.21992076e-07, -1.77402251e-06, ..., -2.30133810e-05, 1.30888320e-05, 1.89649963e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, -7.35410097e-08, -4.65083644e-07, ..., 3.61101207e-04, 3.98091885e-04, 4.33752866e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 1.29378506e-03, 1.47577142e-03, 1.69295853e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, -2.97157612e-07, -1.46258469e-06, ..., -5.94276353e-04, -5.99161664e-04, -5.01382805e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- w_4(time, NC, sample, zh)float320.0 6.668e-08 6.486e-07 ... nan nan
- units :
- m4 s-4
- long_name :
- Moment 4 of the Vertical velocity
array([[[[0.00000000e+00, 6.66771030e-08, 6.48565674e-07, ..., 9.40940401e-04, 9.42035345e-04, 9.61839047e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00000000e+00, 2.27871340e-08, 2.25901502e-07, ..., 5.23299619e-04, 5.03925898e-04, 4.90895996e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00000000e+00, 1.25350823e-08, 1.34344319e-07, ..., 1.23084930e-03, 1.23891840e-03, 1.26626110e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 4.87371767e-03, 5.12770889e-03, 5.47287846e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00000000e+00, 7.93894586e-08, 6.87945487e-07, ..., 2.03512283e-03, 2.13561649e-03, 2.21739407e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- w_2(time, NC, sample, zh)float320.0 9.161e-05 0.000298 ... nan nan
- units :
- m2 s-2
- long_name :
- Moment 2 of the Vertical velocity
array([[[[0.00000000e+00, 9.16085264e-05, 2.97962717e-04, ..., 1.74643099e-02, 1.75024848e-02, 1.77055653e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00000000e+00, 5.97967301e-05, 1.95054061e-04, ..., 1.37713207e-02, 1.34718912e-02, 1.32834027e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00000000e+00, 5.90166273e-05, 1.95237444e-04, ..., 2.14855373e-02, 2.14047786e-02, 2.13972572e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 3.96590754e-02, 4.07278873e-02, 4.19532470e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00000000e+00, 1.41293727e-04, 4.27006744e-04, ..., 2.54677460e-02, 2.61678938e-02, 2.67648324e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- p(time, NC, sample, z)float32-0.108 -0.1084 -0.1087 ... nan nan
- units :
- Pa
- long_name :
- Pressure
array([[[[-0.10800928, -0.10835731, -0.1086956 , ..., -0.11452613, -0.11444441, -0.1144923 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.10794923, -0.10819447, -0.10844407, ..., -0.11419653, -0.11386812, -0.11358403], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.13556617, -0.1357502 , -0.13596681, ..., -0.14468706, -0.14449061, -0.14439411], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[-0.23685719, -0.23728843, -0.23767434, ..., -0.27016094, -0.27144003, -0.27255103], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.23667555, -0.23717429, -0.23758115, ..., -0.25887594, -0.25940368, -0.25994244], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- p_grad(time, NC, sample, zh)float320.0 -3.164e-05 ... nan nan
- units :
- Pa m-1
- long_name :
- Gradient of the Pressure
array([[[[ 0.00000000e+00, -3.16387777e-05, -2.81903576e-05, ..., 2.04312460e-06, -1.19728975e-06, -2.85893657e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, -2.22944054e-05, -2.08010752e-05, ..., 8.21014601e-06, 7.10233735e-06, 5.41984264e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, -1.67290782e-05, -1.80501866e-05, ..., 4.91127457e-06, 2.41244084e-06, 4.58316372e-08], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -3.19765386e-05, -2.77755607e-05, -2.66761272e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, -4.53412904e-05, -3.39050421e-05, ..., -1.31937495e-05, -1.34686043e-05, -1.23689542e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- p_2(time, NC, sample, z)float322.858 2.824 2.792 ... nan nan nan
- units :
- Pa2
- long_name :
- Moment 2 of the Pressure
array([[[[ 2.8579495 , 2.8240483 , 2.7924984 , ..., 2.207691 , 2.1574059 , 2.1123304 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 3.171086 , 3.1687863 , 3.1685035 , ..., 1.1986715 , 1.1707826 , 1.1495337 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 2.9313374 , 2.9286213 , 2.927327 , ..., 2.0324907 , 2.0163167 , 2.0076082 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 2.6819513 , 2.6608226 , 2.6425488 , ..., 4.36505 , 4.3610935 , 4.358871 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.47701955, 0.46205878, 0.44835815, ..., 1.9038005 , 1.9109792 , 1.9085034 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- p_w(time, NC, sample, zh)float320.0 -0.002575 -0.004335 ... nan nan
- units :
- Pa m s-1
- long_name :
- Turbulent flux of the Pressure
array([[[[ 0. , -0.00257495, -0.00433491, ..., 0.0253437 , 0.02622891, 0.02714764], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , -0.00148246, -0.00244596, ..., 0.0190824 , 0.01892645, 0.01881476], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , -0.00158928, -0.00264439, ..., 0.01583462, 0.01583607, 0.01576538], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 0. , -0.00164386, -0.00259885, ..., 0.07761899, 0.08227109, 0.08783305], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , -0.00115905, -0.00175072, ..., 0.00810947, 0.00820806, 0.0077717 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- ke(time, NC, sample, z)float329.795 14.83 19.37 ... nan nan nan
- units :
- m2 s-2
- long_name :
- Kinetic energy
array([[[[ 9.795363 , 14.830974 , 19.365995 , ..., 153.5522 , 160.21724 , 166.9752 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 9.502869 , 14.390937 , 18.814737 , ..., 152.76085 , 159.34296 , 165.98346 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 9.542724 , 14.456715 , 18.916265 , ..., 153.56331 , 160.30305 , 167.13416 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 7.021042 , 10.084592 , 13.051422 , ..., 192.77563 , 206.17482 , 220.02295 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 7.1824226, 10.330031 , 13.365296 , ..., 191.69432 , 204.71935 , 217.97585 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- tke(time, NC, sample, z)float320.3072 0.4609 0.5849 ... nan nan
- units :
- m2 s-2
- long_name :
- Turbulent kinetic energy
array([[[[0.30719957, 0.46090594, 0.5848716 , ..., 0.11605256, 0.1193805 , 0.1237849 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.2225483 , 0.3356966 , 0.42883086, ..., 0.10304159, 0.10031692, 0.10005572], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.11558611, 0.17035629, 0.21464165, ..., 0.13234799, 0.13468122, 0.13618617], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[0.0953754 , 0.1430042 , 0.17612737, ..., 0.5271252 , 0.5071241 , 0.49798715], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.10700534, 0.1651404 , 0.20549679, ..., 0.49899924, 0.49636483, 0.47923926], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- lat(time, NC, sample)float3236.5 36.5 36.5 ... 36.5 36.5 36.5
- units :
- degrees
- long_name :
- Latitude
array([[[36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5]], [[36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5]], [[36.5, 36.5, 36.5], [36.5, 36.5, 36.5], ... [ nan, nan, nan], [36.5, 36.5, 36.5]], [[36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [ nan, nan, nan], [36.5, 36.5, 36.5]], [[36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [36.5, 36.5, 36.5], [ nan, nan, nan], [36.5, 36.5, 36.5]]], dtype=float32)
- lon(time, NC, sample)float32-97.5 -97.5 -97.5 ... -97.5 -97.5
- units :
- degrees
- long_name :
- Longitude
array([[[-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5]], [[-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5]], [[-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], ... [ nan, nan, nan], [-97.5, -97.5, -97.5]], [[-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [ nan, nan, nan], [-97.5, -97.5, -97.5]], [[-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [-97.5, -97.5, -97.5], [ nan, nan, nan], [-97.5, -97.5, -97.5]]], dtype=float32)
- evisc(time, NC, sample, z)float322.098 3.572 4.354 ... nan nan nan
- units :
- m2 s-1
- long_name :
- Eddy viscosity
array([[[[2.0977395, 3.5720208, 4.3541985, ..., 1.6772243, 1.7104617, 1.6868349], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.0781243, 3.5316608, 4.319552 , ..., 1.7484323, 1.7766293, 1.7778598], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.0941918, 3.567627 , 4.3743362, ..., 1.6959138, 1.7910227, 1.8332195], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[1.9394323, 2.46546 , 3.0798695, ..., 3.6931698, 3.6100519, 3.5069816], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[1.9597093, 2.5042717, 3.120716 , ..., 3.447755 , 3.299697 , 3.2448044], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- evisc_2(time, NC, sample, z)float320.1074 0.4291 0.6318 ... nan nan
- units :
- m4 s-2
- long_name :
- Moment 2 of the Eddy viscosity
array([[[[0.10735131, 0.4290662 , 0.63181734, ..., 1.9052709 , 1.9713782 , 1.959446 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.0788307 , 0.32360795, 0.49348748, ..., 1.7801892 , 1.8902756 , 1.9255518 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.0399599 , 0.15465891, 0.25249264, ..., 2.0641997 , 2.038348 , 2.0201557 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[0.04886763, 0.20157452, 0.31760672, ..., 6.110246 , 6.153991 , 6.1157136 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.04385162, 0.23412722, 0.3406634 , ..., 5.7889557 , 5.812475 , 5.8039165 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- nr(time, NC, sample, z)float320.003148 0.003262 ... nan nan
- units :
- m-3
- long_name :
- Number density rain
array([[[[3.1479627e-03, 3.2623836e-03, 3.3518311e-03, ..., 5.4207473e-04, 4.9476390e-04, 4.5082203e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[7.9370715e-05, 8.2239239e-05, 8.4471299e-05, ..., 1.9437164e-06, 1.7863771e-06, 1.6419261e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[8.2359758e-05, 8.5319982e-05, 8.7609260e-05, ..., 2.0887869e-06, 1.8857172e-06, 1.7119846e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[2.8147741e-05, 2.9195335e-05, 3.0110217e-05, ..., 1.1235340e-03, 9.9690992e-04, 8.7939418e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.5819822e-05, 2.6785527e-05, 2.7636333e-05, ..., 2.6740483e-04, 2.3645627e-04, 2.0816055e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- nr_3(time, NC, sample, z)float324.025e-12 4.235e-12 ... nan nan
- units :
- m-9
- long_name :
- Moment 3 of the Number density rain
array([[[[ 4.02455673e-12, 4.23546051e-12, 4.23310475e-12, ..., 9.15468466e-15, 1.13323903e-14, -9.41237834e-16], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 3.81188550e-17, 4.20001519e-17, 4.53645402e-17, ..., 1.18068077e-21, 4.99992512e-22, 1.57913684e-22], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-7.63993785e-19, -8.18762034e-19, -8.24912638e-19, ..., 2.09834981e-21, 7.20141755e-22, 5.26168709e-22], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 3.87546982e-13, 6.99197305e-14, -3.50754311e-14], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-1.13686680e-18, -1.18958777e-18, -1.10628707e-18, ..., 2.87408973e-15, 1.63446686e-15, -5.34798127e-16], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- nr_4(time, NC, sample, z)float328.206e-15 9.307e-15 ... nan nan
- units :
- m-12
- long_name :
- Moment 4 of the Number density rain
array([[[[8.20607467e-15, 9.30712772e-15, 1.02686626e-14, ..., 1.07605039e-17, 7.81798424e-18, 6.64601200e-18], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[7.33173182e-22, 8.21558723e-22, 8.92098539e-22, ..., 1.65672230e-27, 1.03001728e-27, 6.45728536e-28], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[5.94375832e-23, 6.24402150e-23, 6.19400645e-23, ..., 5.78941513e-27, 2.87919690e-27, 1.41365656e-27], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 9.87319073e-16, 6.99550059e-16, 5.18060650e-16], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[1.27811029e-22, 1.42831837e-22, 1.58222539e-22, ..., 2.25692280e-18, 1.66019605e-18, 1.33599761e-18], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- nr_diff(time, NC, sample, zh)float32-8.264e-05 -8.754e-05 ... nan nan
- units :
- m-2 s-1
- long_name :
- Diffusive flux of the Number density rain
array([[[[-8.26411342e-05, -8.75351907e-05, -8.73357276e-05, ..., 4.57057695e-06, 4.19627349e-06, 3.84657460e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-2.06252389e-06, -2.17984439e-06, -2.16897593e-06, ..., 1.63124039e-08, 1.51442077e-08, 1.41378758e-08], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-2.16566855e-06, -2.28179920e-06, -2.26528732e-06, ..., 2.01636166e-08, 1.82692173e-08, 1.64759886e-08], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 2.88765150e-05, 2.59080116e-05, 2.31031572e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-5.00880844e-07, -5.82132259e-07, -5.83734334e-07, ..., 6.11107635e-06, 5.40975316e-06, 4.81929555e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- nr_w(time, NC, sample, zh)float320.0 5.269e-07 1.01e-06 ... nan nan
- units :
- m-2 s-1
- long_name :
- Turbulent flux of the Number density rain
array([[[[ 0.00000000e+00, 5.26867780e-07, 1.01025432e-06, ..., -4.83350107e-07, -4.66095003e-07, -4.69549263e-07], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 6.60265620e-09, 1.26158506e-08, ..., -1.31457123e-09, -1.10652276e-09, -9.84318071e-10], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 1.35220990e-09, 2.44131604e-09, ..., -2.53168975e-09, -2.11530549e-09, -1.53443536e-09], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -4.83853910e-07, -6.24774771e-07, -9.15997987e-07], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 1.07969322e-08, 1.93824761e-08, ..., -1.61054814e-07, -9.23061805e-08, -9.81890764e-08], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- nr_grad(time, NC, sample, zh)float320.0003148 1.04e-05 ... nan nan
- units :
- m-4
- long_name :
- Gradient of the Number density rain
array([[[[ 3.1479631e-04, 1.0401895e-05, 7.4539862e-06, ..., -1.1827725e-06, -1.0985465e-06, -1.0664845e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 7.9370720e-06, 2.6077541e-07, 1.8600430e-07, ..., -3.9334851e-09, -3.6112651e-09, -3.4333925e-09], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 8.2359766e-06, 2.6911150e-07, 1.9077375e-07, ..., -5.0767421e-09, -4.3433164e-09, -3.8263535e-09], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -3.1656018e-06, -2.9378941e-06, -2.7921958e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 2.5819825e-06, 8.7791385e-08, 7.0900384e-08, ..., -7.7371465e-07, -7.0739270e-07, -6.3186127e-07], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- nr_2(time, NC, sample, z)float327.015e-08 7.486e-08 ... nan nan
- units :
- m-6
- long_name :
- Moment 2 of the Number density rain
array([[[[7.01540444e-08, 7.48610915e-08, 7.88278172e-08, ..., 1.87821958e-09, 1.59875269e-09, 1.48401968e-09], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[1.92220077e-11, 2.04218864e-11, 2.14027546e-11, ..., 2.24398493e-14, 1.74261376e-14, 1.41901498e-14], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[4.60553514e-12, 4.73854853e-12, 4.73959977e-12, ..., 4.28393181e-14, 3.08440034e-14, 2.22561820e-14], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 1.71576939e-08, 1.46726755e-08, 1.31231639e-08], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[6.32279689e-12, 6.67718094e-12, 7.01210617e-12, ..., 8.25486179e-10, 6.98727021e-10, 6.33837427e-10], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- nr_path(time, NC, sample)float3236.91 nan nan ... 3.88 nan nan
- units :
- m-5 kg
- long_name :
- Number density rain path
array([[[3.69077530e+01, nan, nan], [6.73391819e-01, nan, nan], [6.18919075e-01, nan, nan], [6.18919075e-01, nan, nan], [2.29406312e-01, nan, nan], [1.67284310e-01, nan, nan], [1.83360338e-01, nan, nan], [1.07336678e-01, nan, nan]], [[3.67975502e+01, nan, nan], [6.71401322e-01, nan, nan], [6.16400123e-01, nan, nan], [6.16400123e-01, nan, nan], [2.28601739e-01, nan, nan], [1.66589156e-01, nan, nan], [1.82385579e-01, nan, nan], [1.06941499e-01, nan, nan]], [[3.66250458e+01, nan, nan], [6.67640865e-01, nan, nan], ... [ nan, nan, nan], [4.12121153e+00, nan, nan]], [[1.55574424e+04, nan, nan], [9.53789673e+01, nan, nan], [7.58012161e+01, nan, nan], [7.58012161e+01, nan, nan], [2.24761887e+01, nan, nan], [1.31636772e+01, nan, nan], [ nan, nan, nan], [3.98081136e+00, nan, nan]], [[1.52495342e+04, nan, nan], [9.27691345e+01, nan, nan], [7.38655090e+01, nan, nan], [7.38655090e+01, nan, nan], [2.21070080e+01, nan, nan], [1.29008636e+01, nan, nan], [ nan, nan, nan], [3.87951922e+00, nan, nan]]], dtype=float32)
- nr_flux(time, NC, sample, zh)float32-8.264e-05 -8.701e-05 ... nan nan
- units :
- m-2 s-1
- long_name :
- Total flux of the Number density rain
array([[[[-8.2641134e-05, -8.7008324e-05, -8.6325475e-05, ..., 4.0872269e-06, 3.7301782e-06, 3.3770255e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-2.0625239e-06, -2.1732415e-06, -2.1563601e-06, ..., 1.4997832e-08, 1.4037685e-08, 1.3153557e-08], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-2.1656685e-06, -2.2804472e-06, -2.2628458e-06, ..., 1.7631924e-08, 1.6153910e-08, 1.4941554e-08], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 2.8392658e-05, 2.5283238e-05, 2.2187158e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-5.0088084e-07, -5.7133525e-07, -5.6435186e-07, ..., 5.9500217e-06, 5.3174476e-06, 4.7211070e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qr(time, NC, sample, z)float322.22e-16 2.236e-16 ... nan nan
- units :
- kg kg-1
- long_name :
- Rain water specific humidity
array([[[[2.2204460e-16, 2.2362739e-16, 2.2486890e-16, ..., 2.2372507e-16, 2.2360579e-16, 2.2349343e-16], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.2204460e-16, 2.2397325e-16, 2.2548194e-16, ..., 2.2266781e-16, 2.2262173e-16, 2.2257943e-16], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.2204460e-16, 2.2435806e-16, 2.2615915e-16, ..., 2.2273168e-16, 2.2267482e-16, 2.2262575e-16], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[3.7554593e-16, 3.8938062e-16, 4.0137673e-16, ..., 2.2911876e-16, 2.2878299e-16, 2.2843370e-16], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[3.3423052e-16, 3.4655646e-16, 3.5728064e-16, ..., 2.7610122e-16, 2.7304941e-16, 2.6992166e-16], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qr_3(time, NC, sample, z)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- kg3 kg-3
- long_name :
- Moment 3 of the Rain water specific humidity
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qr_4(time, NC, sample, z)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- kg4 kg-4
- long_name :
- Moment 4 of the Rain water specific humidity
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qr_diff(time, NC, sample, zh)float32-5.882e-18 -1.192e-18 ... nan nan
- units :
- kg kg-1 m s-1
- long_name :
- Diffusive flux of the Rain water specific humidity
array([[[[-5.88247011e-18, -1.19247670e-18, -1.19681837e-18, ..., 1.15305690e-20, 1.07268039e-20, 1.00071592e-20], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-5.79815683e-18, -1.45152630e-18, -1.45543670e-18, ..., 4.77716958e-21, 4.43206401e-21, 4.15000350e-21], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-5.83872880e-18, -1.78690141e-18, -1.79049820e-18, ..., 5.64627710e-21, 5.16263130e-21, 4.71428205e-21], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 7.68798011e-20, 7.71060092e-20, 7.70576837e-20], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-6.49633118e-18, -7.45220497e-18, -7.39610393e-18, ..., 6.05926291e-19, 6.00330724e-19, 5.95907272e-19], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qr_w(time, NC, sample, zh)float320.0 4.027e-22 2.304e-21 ... nan nan
- units :
- kg kg-1 m s-1
- long_name :
- Turbulent flux of the Rain water specific humidity
array([[[[ 0.00000000e+00, 4.02657199e-22, 2.30372244e-21, ..., -1.16953413e-21, -1.14102366e-21, -1.17543658e-21], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 2.60299638e-22, 1.58521006e-21, ..., -3.88918145e-22, -3.24997339e-22, -2.87731222e-22], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 2.32686499e-23, 4.16562179e-22, ..., -7.11142935e-22, -5.97616233e-22, -4.40953455e-22], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -6.49325320e-22, -9.53422086e-22, -1.85437636e-21], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 1.02804566e-19, 1.84055196e-19, ..., -1.19520369e-20, -8.93570742e-21, -1.28143161e-20], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qr_grad(time, NC, sample, zh)float322.22e-17 1.439e-19 ... nan nan
- units :
- kg kg-1 m-1
- long_name :
- Gradient of the Rain water specific humidity
array([[[[ 2.22044608e-17, 1.43890885e-19, 1.03456339e-19, ..., -2.98230660e-21, -2.80853607e-21, -2.77556254e-21], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 2.22044608e-17, 1.75330094e-19, 1.25726374e-19, ..., -1.15224149e-21, -1.05684864e-21, -1.00836487e-21], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 2.22044608e-17, 2.10314845e-19, 1.50088613e-19, ..., -1.42096462e-21, -1.22710578e-21, -1.09481030e-21], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -8.39438638e-21, -8.73202632e-21, -9.31879141e-21], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 3.34230499e-17, 1.12054322e-18, 8.93679937e-19, ..., -7.62959925e-20, -7.81935449e-20, -7.78816073e-20], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qr_2(time, NC, sample, z)float320.0 2.166e-37 6.78e-37 ... nan nan
- units :
- kg2 kg-2
- long_name :
- Moment 2 of the Rain water specific humidity
array([[[[0.0000000e+00, 2.1656054e-37, 6.7797053e-37, ..., 1.1542481e-38, 1.0030577e-38, 9.6560366e-39], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.0000000e+00, 2.0457695e-37, 6.2866168e-37, ..., 1.9484691e-39, 1.4900455e-39, 1.2097494e-39], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.0000000e+00, 1.6911261e-37, 4.8701700e-37, ..., 3.2856708e-39, 2.3879331e-39, 1.7555047e-39], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[5.4105401e-34, 5.6271185e-34, 5.8222368e-34, ..., 1.0040613e-37, 1.1197119e-37, 1.2679795e-37], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.8337466e-34, 2.9799792e-34, 3.1175986e-34, ..., 6.7424524e-36, 7.3239870e-36, 8.3071692e-36], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qr_path(time, NC, sample)float322.477e-12 nan nan ... nan nan
- units :
- kg m-2
- long_name :
- Rain water specific humidity path
array([[[2.47702184e-12, nan, nan], [2.48390132e-12, nan, nan], [2.50920487e-12, nan, nan], [2.50920487e-12, nan, nan], [2.58992936e-12, nan, nan], [2.60257701e-12, nan, nan], [2.56040675e-12, nan, nan], [2.61850589e-12, nan, nan]], [[2.47666818e-12, nan, nan], [2.48302138e-12, nan, nan], [2.50766573e-12, nan, nan], [2.50766573e-12, nan, nan], [2.58592995e-12, nan, nan], [2.59927171e-12, nan, nan], [2.55750217e-12, nan, nan], [2.61752447e-12, nan, nan]], [[2.47584006e-12, nan, nan], [2.48183678e-12, nan, nan], ... [ nan, nan, nan], [3.32723541e-12, nan, nan]], [[3.39538640e-12, nan, nan], [3.16361715e-12, nan, nan], [3.34187279e-12, nan, nan], [3.34187279e-12, nan, nan], [3.44044433e-12, nan, nan], [3.33945480e-12, nan, nan], [ nan, nan, nan], [3.29048247e-12, nan, nan]], [[3.36109504e-12, nan, nan], [3.13615171e-12, nan, nan], [3.30498584e-12, nan, nan], [3.30498584e-12, nan, nan], [3.40443927e-12, nan, nan], [3.30392137e-12, nan, nan], [ nan, nan, nan], [3.25813009e-12, nan, nan]]], dtype=float32)
- qr_flux(time, NC, sample, zh)float32-5.882e-18 -1.192e-18 ... nan nan
- units :
- kg kg-1 m s-1
- long_name :
- Total flux of the Rain water specific humidity
array([[[[-5.88247011e-18, -1.19207397e-18, -1.19451467e-18, ..., 1.03610357e-20, 9.58578011e-21, 8.83172258e-21], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-5.79815683e-18, -1.45126605e-18, -1.45385140e-18, ..., 4.38825131e-21, 4.10706685e-21, 3.86227188e-21], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-5.83872880e-18, -1.78687825e-18, -1.79008171e-18, ..., 4.93513402e-21, 4.56501553e-21, 4.27332854e-21], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 7.62304837e-20, 7.61525930e-20, 7.52033063e-20], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-6.49633118e-18, -7.34940131e-18, -7.21204797e-18, ..., 5.93974255e-19, 5.91395054e-19, 5.83093004e-19], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qt(time, NC, sample, z)float320.01287 0.01284 0.01281 ... nan nan
- units :
- kg kg-1
- long_name :
- Total water mixing ratio
array([[[[0.01287029, 0.01283774, 0.01281243, ..., 0.0034652 , 0.00343089, 0.00339768], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.01280969, 0.01277671, 0.01275115, ..., 0.00346343, 0.00343073, 0.00339915], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.01276983, 0.01273711, 0.0127118 , ..., 0.00346159, 0.00342722, 0.00339585], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[0.01324369, 0.01323528, 0.01322762, ..., 0.00264176, 0.00258578, 0.00253013], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.01325383, 0.01324546, 0.01323776, ..., 0.00266066, 0.00259785, 0.00253677], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qt_3(time, NC, sample, z)float32-6.863e-14 -6.587e-14 ... nan nan
- units :
- kg3 kg-3
- long_name :
- Moment 3 of the Total water mixing ratio
array([[[[-6.86283644e-14, -6.58671590e-14, -5.68206879e-14, ..., -7.04244103e-16, 1.56713885e-15, -1.99117011e-15], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-1.20937697e-13, -1.35849760e-13, -1.47553668e-13, ..., 2.98773910e-15, 1.43305015e-15, 9.20618935e-17], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-1.41049017e-15, -1.16092081e-15, -9.25048387e-16, ..., 1.55012959e-15, -9.68085814e-16, 3.01423742e-16], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 4.89087359e-16, -1.85081239e-14, -2.10561159e-14], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 3.32524298e-14, 3.34542608e-14, 3.34335695e-14, ..., 1.15985661e-15, -5.79176735e-15, -2.28340652e-14], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qt_4(time, NC, sample, z)float321.377e-16 1.562e-16 ... nan nan
- units :
- kg4 kg-4
- long_name :
- Moment 4 of the Total water mixing ratio
array([[[[1.37723759e-16, 1.56152258e-16, 1.73939539e-16, ..., 2.10297270e-18, 1.88720781e-18, 1.99201552e-18], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.87338259e-17, 3.32124861e-17, 3.75803141e-17, ..., 1.93598872e-18, 1.73045729e-18, 1.35549094e-18], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[1.40590171e-18, 1.28933748e-18, 1.25269928e-18, ..., 2.94173964e-18, 2.02081154e-18, 1.27786780e-18], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 2.65940205e-17, 2.69076377e-17, 2.71175729e-17], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[5.55513448e-18, 5.67886291e-18, 5.80068470e-18, ..., 3.08245447e-17, 2.82880119e-17, 2.94486721e-17], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qt_diff(time, NC, sample, zh)float322.366e-05 2.446e-05 ... nan nan
- units :
- kg kg-1 m s-1
- long_name :
- Diffusive flux of the Total water mixing ratio
array([[[[2.36589749e-05, 2.44553794e-05, 2.43059276e-05, ..., 3.34273159e-06, 3.19907463e-06, 3.02014246e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.39176807e-05, 2.46977634e-05, 2.44985131e-05, ..., 3.42919043e-06, 3.33454477e-06, 3.19206470e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.41104226e-05, 2.49883960e-05, 2.48563501e-05, ..., 3.44928799e-06, 3.33063940e-06, 3.14382191e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 1.27992416e-05, 1.22783786e-05, 1.17636655e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[4.02239175e-06, 4.96608163e-06, 5.19141986e-06, ..., 1.24142507e-05, 1.16805832e-05, 1.10967221e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qt_w(time, NC, sample, zh)float320.0 -1.786e-07 ... nan nan
- units :
- kg kg-1 m s-1
- long_name :
- Turbulent flux of the Total water mixing ratio
array([[[[ 0.0000000e+00, -1.7859433e-07, -3.6079166e-07, ..., -2.5410040e-07, -2.6241665e-07, -2.8879438e-07], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.0000000e+00, -9.1905285e-08, -1.9050391e-07, ..., -2.3490503e-07, -2.0891366e-07, -1.9314109e-07], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.0000000e+00, -2.1584393e-08, -4.8769127e-08, ..., -3.6157221e-07, -3.2599658e-07, -2.5277868e-07], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -1.7362777e-07, -2.4139334e-07, -3.8677965e-07], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.0000000e+00, -1.8173621e-07, -3.2947253e-07, ..., -3.0179135e-07, -2.0541707e-07, -2.5850099e-07], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qt_grad(time, NC, sample, zh)float32-9.193e-05 -2.959e-06 ... nan nan
- units :
- kg kg-1 m-1
- long_name :
- Gradient of the Total water mixing ratio
array([[[[-9.1927293e-05, -2.9594521e-06, -2.1085491e-06, ..., -8.5772155e-07, -8.3040692e-07, -8.2973361e-07], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-9.3494098e-05, -2.9988857e-06, -2.1292781e-06, ..., -8.1757361e-07, -7.8938689e-07, -7.7220784e-07], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-9.2585622e-05, -2.9747891e-06, -2.1093197e-06, ..., -8.5918646e-07, -7.8420959e-07, -7.2455032e-07], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -1.3995486e-06, -1.3912368e-06, -1.4229577e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-2.1160449e-05, -7.6053402e-07, -6.4207347e-07, ..., -1.5704244e-06, -1.5269998e-06, -1.4548618e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qt_2(time, NC, sample, z)float329.334e-09 1.002e-08 ... nan nan
- units :
- kg2 kg-2
- long_name :
- Moment 2 of the Total water mixing ratio
array([[[[9.3335766e-09, 1.0018840e-08, 1.0625603e-08, ..., 8.3812363e-10, 7.9615309e-10, 8.1174178e-10], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[3.8155821e-09, 4.1742236e-09, 4.5006936e-09, ..., 8.1970358e-10, 7.3845979e-10, 6.5589961e-10], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[7.0571343e-10, 6.8789358e-10, 6.8623168e-10, ..., 9.8909736e-10, 8.3449442e-10, 6.8084488e-10], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[1.7287322e-09, 1.7309620e-09, 1.7380382e-09, ..., 2.8781697e-09, 2.8805580e-09, 2.9996368e-09], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[1.1571061e-09, 1.1714539e-09, 1.1858753e-09, ..., 3.0509042e-09, 2.9214273e-09, 3.0023859e-09], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qt_path(time, NC, sample)float3235.09 nan nan ... 35.23 nan nan
- units :
- kg m-2
- long_name :
- Total water mixing ratio path
array([[[35.09441 , nan, nan], [35.075603 , nan, nan], [35.08698 , nan, nan], [35.08698 , nan, nan], [35.0945 , nan, nan], [35.093426 , nan, nan], [35.086 , nan, nan], [35.07809 , nan, nan]], [[34.916935 , nan, nan], [34.89986 , nan, nan], [34.911453 , nan, nan], [34.911453 , nan, nan], [34.9231 , nan, nan], [34.924908 , nan, nan], [34.91966 , nan, nan], [34.90865 , nan, nan]], [[34.736526 , nan, nan], [34.72115 , nan, nan], ... [ nan, nan, nan], [36.099503 , nan, nan]], [[35.64555 , nan, nan], [35.613373 , nan, nan], [35.591663 , nan, nan], [35.591663 , nan, nan], [35.586906 , nan, nan], [35.60393 , nan, nan], [ nan, nan, nan], [35.586308 , nan, nan]], [[35.284374 , nan, nan], [35.255238 , nan, nan], [35.230633 , nan, nan], [35.230633 , nan, nan], [35.22034 , nan, nan], [35.242916 , nan, nan], [ nan, nan, nan], [35.229443 , nan, nan]]], dtype=float32)
- qt_flux(time, NC, sample, zh)float322.366e-05 2.428e-05 ... nan nan
- units :
- kg kg-1 m s-1
- long_name :
- Total flux of the Total water mixing ratio
array([[[[2.36589749e-05, 2.42767856e-05, 2.39451347e-05, ..., 3.08863127e-06, 2.93665812e-06, 2.73134810e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.39176807e-05, 2.46058571e-05, 2.43080085e-05, ..., 3.19428545e-06, 3.12563066e-06, 2.99892349e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.41104226e-05, 2.49668101e-05, 2.48075794e-05, ..., 3.08771564e-06, 3.00464239e-06, 2.89104287e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 1.26256127e-05, 1.20369850e-05, 1.13768847e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[4.02239175e-06, 4.78434504e-06, 4.86194767e-06, ..., 1.21124604e-05, 1.14751665e-05, 1.08382219e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thl(time, NC, sample, z)float32301.9 301.9 302.0 ... nan nan nan
- units :
- K
- long_name :
- Liquid water potential temperature
array([[[[301.8982 , 301.92798, 301.95386, ..., 315.44577, 315.50064, 315.55667], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[301.96854, 301.9997 , 302.02646, ..., 315.45566, 315.50854, 315.56235], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[302.00003, 302.03192, 302.05902, ..., 315.4506 , 315.5055 , 315.55826], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[305.4972 , 305.58676, 305.66403, ..., 316.71423, 316.8317 , 316.95273], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[305.46396, 305.5525 , 305.62918, ..., 316.69632, 316.82654, 316.95792], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thl_3(time, NC, sample, z)float320.0001191 0.0001081 ... nan nan
- units :
- K3
- long_name :
- Moment 3 of the Liquid water potential temperature
array([[[[ 1.19082608e-04, 1.08137996e-04, 7.05633138e-05, ..., 1.02648119e-05, 6.94127721e-07, 1.85313511e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 1.43887548e-04, 1.68289713e-04, 1.91936459e-04, ..., -7.71501436e-06, -1.76041738e-06, 6.52918015e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 1.24668650e-05, 1.17692034e-05, 1.09536277e-05, ..., 1.86890259e-06, 1.10436013e-05, 5.85230237e-06], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 9.09579467e-05, 2.67619384e-04, 2.93127930e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-1.16207253e-03, -1.12733431e-03, -1.08166819e-03, ..., 8.50136639e-05, 1.56937647e-04, 3.27268732e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thl_4(time, NC, sample, z)float320.0007332 0.0008012 ... nan nan
- units :
- K4
- long_name :
- Moment 4 of the Liquid water potential temperature
array([[[[7.33237190e-04, 8.01223796e-04, 8.65319103e-04, ..., 1.25900433e-05, 1.34630964e-05, 1.80966836e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[1.00472163e-04, 1.09841116e-04, 1.18703116e-04, ..., 1.27009125e-05, 1.38067408e-05, 1.31977240e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[7.21509650e-06, 7.25783457e-06, 7.25815653e-06, ..., 1.68208189e-05, 1.39780577e-05, 1.10715519e-05], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 4.61318094e-04, 5.60232962e-04, 6.52994961e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[6.04892382e-04, 5.74022008e-04, 5.32370759e-04, ..., 5.15479071e-04, 5.44183247e-04, 6.62755396e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thl_diff(time, NC, sample, zh)float32-0.01687 -0.02254 ... nan nan
- units :
- K m s-1
- long_name :
- Diffusive flux of the Liquid water potential temperature
array([[[[-1.6871180e-02, -2.2537628e-02, -2.4876773e-02, ..., -5.3352290e-03, -5.3698174e-03, -5.3868666e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-1.7886596e-02, -2.3501547e-02, -2.5716608e-02, ..., -5.5510304e-03, -5.6670480e-03, -5.7420996e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-1.8883569e-02, -2.4556058e-02, -2.6759094e-02, ..., -5.4966123e-03, -5.5814814e-03, -5.5973320e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... -2.6878783e-02, -2.6723184e-02, -2.6560239e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-4.5115355e-02, -5.3048674e-02, -5.2460879e-02, ..., -2.5752222e-02, -2.5138177e-02, -2.4810081e-02], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thl_w(time, NC, sample, zh)float320.0 0.0002958 0.0005635 ... nan nan
- units :
- K m s-1
- long_name :
- Turbulent flux of the Liquid water potential temperature
array([[[[ 0.00000000e+00, 2.95782025e-04, 5.63540787e-04, ..., 4.08346794e-04, 4.40372387e-04, 5.08862955e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 1.39292955e-04, 2.68803560e-04, ..., 4.30678105e-04, 3.89658991e-04, 3.65182961e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 4.30848741e-05, 8.13320294e-05, ..., 6.11364783e-04, 5.90903859e-04, 5.19957393e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 3.31949908e-04, 4.74436121e-04, 7.91185594e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.00000000e+00, 3.59592028e-04, 6.83759805e-04, ..., 5.99523366e-04, 4.53586021e-04, 6.19524217e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thl_grad(time, NC, sample, zh)float320.07533 0.002707 ... nan nan
- units :
- K m-1
- long_name :
- Gradient of the Liquid water potential temperature
array([[[[ 7.53307045e-02, 2.70666461e-03, 2.15476588e-03, ..., 1.37130928e-03, 1.40075746e-03, 1.49094232e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 7.97946900e-02, 2.83365906e-03, 2.23094551e-03, ..., 1.32258143e-03, 1.34466635e-03, 1.39815826e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 8.24372470e-02, 2.89949193e-03, 2.25984608e-03, ..., 1.37308950e-03, 1.31893356e-03, 1.29700371e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 2.93585868e-03, 3.02611780e-03, 3.21307569e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 2.47533903e-01, 8.04916769e-03, 6.39063865e-03, ..., 3.25494842e-03, 3.28469952e-03, 3.25188995e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thl_2(time, NC, sample, z)float320.02109 0.02211 0.02302 ... nan nan
- units :
- K2
- long_name :
- Moment 2 of the Liquid water potential temperature
array([[[[0.02109147, 0.02210594, 0.0230155 , ..., 0.00205197, 0.00212711, 0.00243432], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00717329, 0.00751971, 0.00783541, ..., 0.00210765, 0.00206895, 0.00201906], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00162212, 0.00163538, 0.00164291, ..., 0.00237253, 0.0021978 , 0.00200051], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[0.0121158 , 0.01144692, 0.01085408, ..., 0.01206279, 0.01312798, 0.01472034], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.01039871, 0.00998918, 0.0094676 , ..., 0.01247361, 0.01291636, 0.01432262], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thl_path(time, NC, sample)float323.368e+06 nan nan ... nan nan
- units :
- K kg m-2
- long_name :
- Liquid water potential temperature path
array([[[3368451.2 , nan, nan], [3368494.2 , nan, nan], [3368427.8 , nan, nan], [3368427.8 , nan, nan], [3368492. , nan, nan], [3368512.8 , nan, nan], [3368386.2 , nan, nan], [3368517.8 , nan, nan]], [[3368989.8 , nan, nan], [3369042. , nan, nan], [3368985.2 , nan, nan], [3368985.2 , nan, nan], [3369041.2 , nan, nan], [3369012. , nan, nan], [3368860.8 , nan, nan], [3368941.2 , nan, nan]], [[3369425. , nan, nan], [3369521.2 , nan, nan], ... [ nan, nan, nan], [3384074.2 , nan, nan]], [[3383881.2 , nan, nan], [3384029.2 , nan, nan], [3383773.2 , nan, nan], [3383773.2 , nan, nan], [3383835. , nan, nan], [3383924. , nan, nan], [ nan, nan, nan], [3383843.8 , nan, nan]], [[3383808. , nan, nan], [3383709.2 , nan, nan], [3383818.8 , nan, nan], [3383818.8 , nan, nan], [3383766.2 , nan, nan], [3383831. , nan, nan], [ nan, nan, nan], [3383922.2 , nan, nan]]], dtype=float32)
- thl_flux(time, NC, sample, zh)float32-0.01687 -0.02224 ... nan nan
- units :
- K m s-1
- long_name :
- Total flux of the Liquid water potential temperature
array([[[[-0.01687118, -0.02224185, -0.02431323, ..., -0.00492688, -0.00492944, -0.004878 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.0178866 , -0.02336225, -0.0254478 , ..., -0.00512035, -0.00527739, -0.00537692], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.01888357, -0.02451297, -0.02667776, ..., -0.00488525, -0.00499058, -0.00507737], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[-0.04489011, -0.05259382, -0.05170287, ..., -0.02654684, -0.02624875, -0.02576905], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.04511536, -0.05268908, -0.05177712, ..., -0.0251527 , -0.02468459, -0.02419056], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- nr_bot(time, NC, sample)float320.0 0.0 0.0 0.0 ... nan 0.0 0.0 0.0
- units :
- m-3
- long_name :
- Surface Number density rain
array([[[ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.]], [[ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.]], [[ 0., 0., 0.], [ 0., 0., 0.], ... [nan, nan, nan], [ 0., 0., 0.]], [[ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [nan, nan, nan], [ 0., 0., 0.]], [[ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [nan, nan, nan], [ 0., 0., 0.]]], dtype=float32)
- qr_bot(time, NC, sample)float320.0 0.0 0.0 0.0 ... nan 0.0 0.0 0.0
- units :
- kg kg-1
- long_name :
- Surface Rain water specific humidity
array([[[ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.]], [[ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.]], [[ 0., 0., 0.], [ 0., 0., 0.], ... [nan, nan, nan], [ 0., 0., 0.]], [[ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [nan, nan, nan], [ 0., 0., 0.]], [[ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [nan, nan, nan], [ 0., 0., 0.]]], dtype=float32)
- qt_bot(time, NC, sample)float320.01379 0.01379 ... 0.01347 0.01347
- units :
- kg kg-1
- long_name :
- Surface Total water mixing ratio
array([[[0.01378956, 0.01378956, 0.01378956], [0.01374463, 0.01374463, 0.01374463], [0.01369569, 0.01369569, 0.01369569], [0.01369569, 0.01369569, 0.01369569], [0.01372141, 0.01372141, 0.01372141], [0.01373716, 0.01373716, 0.01373716], [0.01372998, 0.01372998, 0.01372998], [0.01372085, 0.01372085, 0.01372085]], [[0.01435342, 0.01435342, 0.01435342], [0.01431251, 0.01431251, 0.01431251], [0.01424903, 0.01424903, 0.01424903], [0.01424903, 0.01424903, 0.01424903], [0.01428383, 0.01428383, 0.01428383], [0.01429186, 0.01429186, 0.01429186], [0.01427338, 0.01427338, 0.01427338], [0.01426818, 0.01426818, 0.01426818]], [[0.01495252, 0.01495252, 0.01495252], [0.01490177, 0.01490177, 0.01490177], ... [ nan, nan, nan], [0.01363285, 0.01363285, 0.01363285]], [[0.01343158, 0.01343158, 0.01343158], [0.01349973, 0.01349973, 0.01349973], [0.01348393, 0.01348393, 0.01348393], [0.01348393, 0.01348393, 0.01348393], [0.01346918, 0.01346918, 0.01346918], [0.01347318, 0.01347318, 0.01347318], [ nan, nan, nan], [0.01347779, 0.01347779, 0.01347779]], [[0.0134158 , 0.0134158 , 0.0134158 ], [0.01348866, 0.01348866, 0.01348866], [0.01347347, 0.01347347, 0.01347347], [0.01347347, 0.01347347, 0.01347347], [0.0134562 , 0.0134562 , 0.0134562 ], [0.01345891, 0.01345891, 0.01345891], [ nan, nan, nan], [0.01346543, 0.01346543, 0.01346543]]], dtype=float32)
- thl_bot(time, NC, sample)float32301.1 301.1 301.1 ... 303.0 303.0
- units :
- K
- long_name :
- Surface Liquid water potential temperature
array([[[301.1449 , 301.1449 , 301.1449 ], [301.1706 , 301.1706 , 301.1706 ], [301.17566, 301.17566, 301.17566], [301.17566, 301.17566, 301.17566], [301.17178, 301.17178, 301.17178], [301.1533 , 301.1533 , 301.1533 ], [301.14688, 301.14688, 301.14688], [301.15213, 301.15213, 301.15213]], [[302.35922, 302.35922, 302.35922], [302.3748 , 302.3748 , 302.3748 ], [302.37445, 302.37445, 302.37445], [302.37445, 302.37445, 302.37445], [302.3777 , 302.3777 , 302.3777 ], [302.3667 , 302.3667 , 302.3667 ], [302.36362, 302.36362, 302.36362], [302.36658, 302.36658, 302.36658]], [[303.74527, 303.74527, 303.74527], [303.72552, 303.72552, 303.72552], ... [ nan, nan, nan], [303.70557, 303.70557, 303.70557]], [[303.35815, 303.35815, 303.35815], [303.3218 , 303.3218 , 303.3218 ], [303.28793, 303.28793, 303.28793], [303.28793, 303.28793, 303.28793], [303.34378, 303.34378, 303.34378], [303.30133, 303.30133, 303.30133], [ nan, nan, nan], [303.29636, 303.29636, 303.29636]], [[303.04904, 303.04904, 303.04904], [303.00464, 303.00464, 303.00464], [302.97113, 302.97113, 302.97113], [302.97113, 302.97113, 302.97113], [303.02817, 303.02817, 303.02817], [302.99704, 302.99704, 302.99704], [ nan, nan, nan], [302.98862, 302.98862, 302.98862]]], dtype=float32)
- rhoref(time, NC, sample, z)float321.099 1.098 1.097 ... 0.7671 0.7642
- units :
- kg m-3
- long_name :
- Full level basic state density
array([[[[1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521]], [[1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521]], [[1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521]], ... [[1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521]], [[1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521]], [[1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521], [1.0990218, 1.0980556, 1.0970019, ..., 0.7700085, 0.7670752, 0.7641521]]]], dtype=float32)
- rhorefh(time, NC, sample, zh)float321.1 1.099 1.098 ... 0.7656 0.7627
- units :
- kg m-3
- long_name :
- Half level basic state density
array([[[[1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ]], [[1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ]], [[1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ]], ... [[1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ]], [[1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ]], [[1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ], [1.0999006 , 1.0985384 , 1.0975285 , ..., 0.7685406 , 0.76561254, 0.7627004 ]]]], dtype=float32)
- thvref(time, NC, sample, z)float32308.7 308.7 308.7 ... 317.2 317.3
- units :
- K
- long_name :
- Full level basic state virtual potential temperature
array([[[[308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ]], [[308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ]], [[308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ]], ... [[308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ]], [[308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ]], [[308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.12262, 317.20667, 317.2898 ]]]], dtype=float32)
- thvrefh(time, NC, sample, zh)float32308.7 308.7 308.7 ... 317.2 317.3
- units :
- K
- long_name :
- Half level basic state virtual potential temperature
array([[[[308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ]], [[308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ]], [[308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ]], ... [[308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ]], [[308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ]], [[308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ], [308.6595 , 308.6595 , 308.6595 , ..., 317.16467, 317.24823, 317.3285 ]]]], dtype=float32)
- phydro(time, NC, sample, z)float329.657e+04 9.645e+04 ... 6.045e+04
- units :
- Pa
- long_name :
- Full level hydrostatic pressure
array([[[[96566.07 , 96445.625, 96314.35 , ..., 60917.598, 60614.652, 60312.83 ], [96566.07 , 96445.625, 96314.35 , ..., 60917.598, 60614.652, 60312.83 ], [96566.07 , 96445.625, 96314.35 , ..., 60917.598, 60614.652, 60312.83 ]], [[96566.09 , 96445.66 , 96314.414, ..., 60917.777, 60614.832, 60313.02 ], [96566.09 , 96445.66 , 96314.414, ..., 60917.777, 60614.832, 60313.02 ], [96566.09 , 96445.66 , 96314.414, ..., 60917.777, 60614.832, 60313.02 ]], [[96566.09 , 96445.664, 96314.44 , ..., 60916.824, 60613.887, 60312.066], [96566.09 , 96445.664, 96314.44 , ..., 60916.824, 60613.887, 60312.066], [96566.09 , 96445.664, 96314.44 , ..., 60916.824, 60613.887, 60312.066]], ... [[96546.22 , 96427.24 , 96297.6 , ..., 61050.344, 60748.02 , 60446.863], [96546.22 , 96427.24 , 96297.6 , ..., 61050.344, 60748.02 , 60446.863], [96546.22 , 96427.24 , 96297.6 , ..., 61050.344, 60748.02 , 60446.863]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[96546.21 , 96427.22 , 96297.56 , ..., 61049.152, 60746.816, 60445.676], [96546.21 , 96427.22 , 96297.56 , ..., 61049.152, 60746.816, 60445.676], [96546.21 , 96427.22 , 96297.56 , ..., 61049.152, 60746.816, 60445.676]]]], dtype=float32)
- phydroh(time, NC, sample, zh)float329.668e+04 9.651e+04 ... 6.03e+04
- units :
- Pa
- long_name :
- Half level hydrostatic pressure
array([[[[96675.9 , 96506.03 , 96380.164, ..., 60766.13 , 60463.75 , 60162.484], [96675.9 , 96506.03 , 96380.164, ..., 60766.13 , 60463.75 , 60162.484], [96675.9 , 96506.03 , 96380.164, ..., 60766.13 , 60463.75 , 60162.484]], [[96675.9 , 96506.06 , 96380.23 , ..., 60766.3 , 60463.934, 60162.676], [96675.9 , 96506.06 , 96380.23 , ..., 60766.3 , 60463.934, 60162.676], [96675.9 , 96506.06 , 96380.23 , ..., 60766.3 , 60463.934, 60162.676]], [[96675.9 , 96506.086, 96380.25 , ..., 60765.375, 60462.996, 60161.746], [96675.9 , 96506.086, 96380.25 , ..., 60765.375, 60462.996, 60161.746], [96675.9 , 96506.086, 96380.25 , ..., 60765.375, 60462.996, 60161.746]], ... [[96655.35 , 96487.555, 96363.24 , ..., 60899.52 , 60597.777, 60297.207], [96655.35 , 96487.555, 96363.24 , ..., 60899.52 , 60597.777, 60297.207], [96655.35 , 96487.555, 96363.24 , ..., 60899.52 , 60597.777, 60297.207]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[96655.35 , 96487.54 , 96363.21 , ..., 60898.332, 60596.594, 60296.03 ], [96655.35 , 96487.54 , 96363.21 , ..., 60898.332, 60596.594, 60296.03 ], [96655.35 , 96487.54 , 96363.21 , ..., 60898.332, 60596.594, 60296.03 ]]]], dtype=float32)
- rho(time, NC, sample, z)float321.117 1.116 1.115 ... 0.769 0.7659
- units :
- kg m-3
- long_name :
- Full level density
array([[[[1.1167909 , 1.1157075 , 1.114544 , ..., 0.7734673 , 0.77059954, 0.7677355 ], [1.1167909 , 1.1157075 , 1.114544 , ..., 0.7734673 , 0.77059954, 0.7677355 ], [1.1167909 , 1.1157075 , 1.114544 , ..., 0.7734673 , 0.77059954, 0.7677355 ]], [[1.1165718 , 1.1154839 , 1.1143175 , ..., 0.7734456 , 0.7705819 , 0.7677228 ], [1.1165718 , 1.1154839 , 1.1143175 , ..., 0.7734456 , 0.7705819 , 0.7677228 ], [1.1165718 , 1.1154839 , 1.1143175 , ..., 0.7734456 , 0.7705819 , 0.7677228 ]], [[1.1164823 , 1.1153917 , 1.1142241 , ..., 0.7734502 , 0.7705824 , 0.76772565], [1.1164823 , 1.1153917 , 1.1142241 , ..., 0.7734502 , 0.7705824 , 0.76772565], [1.1164823 , 1.1153917 , 1.1142241 , ..., 0.7734502 , 0.7705824 , 0.76772565]], ... [[1.1032239 , 1.101935 , 1.1006032 , ..., 0.7719539 , 0.76896197, 0.76597005], [1.1032239 , 1.101935 , 1.1006032 , ..., 0.7719539 , 0.76896197, 0.76597005], [1.1032239 , 1.101935 , 1.1006032 , ..., 0.7719539 , 0.76896197, 0.76597005]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[1.103337 , 1.1020517 , 1.1007217 , ..., 0.7719779 , 0.76895803, 0.7659435 ], [1.103337 , 1.1020517 , 1.1007217 , ..., 0.7719779 , 0.76895803, 0.7659435 ], [1.103337 , 1.1020517 , 1.1007217 , ..., 0.7719779 , 0.76895803, 0.7659435 ]]]], dtype=float32)
- rhoh(time, NC, sample, zh)float321.12 1.116 1.115 ... 0.7675 0.7644
- units :
- kg m-3
- long_name :
- Half level density
array([[[[1.1200031 , 1.1162508 , 1.1151272 , ..., 0.77203375, 0.7691679 , 0.76630306], [1.1200031 , 1.1162508 , 1.1151272 , ..., 0.77203375, 0.7691679 , 0.76630306], [1.1200031 , 1.1162508 , 1.1151272 , ..., 0.77203375, 0.7691679 , 0.76630306]], [[1.1199381 , 1.1160295 , 1.1149024 , ..., 0.77201414, 0.7691527 , 0.7662943 ], [1.1199381 , 1.1160295 , 1.1149024 , ..., 0.77201414, 0.7691527 , 0.7662943 ], [1.1199381 , 1.1160295 , 1.1149024 , ..., 0.77201414, 0.7691527 , 0.7662943 ]], [[1.1199505 , 1.1159387 , 1.1148096 , ..., 0.7720168 , 0.76915455, 0.7663018 ], [1.1199505 , 1.1159387 , 1.1148096 , ..., 0.7720168 , 0.76915455, 0.7663018 ], [1.1199505 , 1.1159387 , 1.1148096 , ..., 0.7720168 , 0.76915455, 0.7663018 ]], ... [[1.1130743 , 1.1025862 , 1.1012758 , ..., 0.77046126, 0.76746935, 0.76447296], [1.1130743 , 1.1025862 , 1.1012758 , ..., 0.77046126, 0.76746935, 0.76447296], [1.1130743 , 1.1025862 , 1.1012758 , ..., 0.77046126, 0.76746935, 0.76447296]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[1.113101 , 1.102701 , 1.1013933 , ..., 0.7704713 , 0.76745415, 0.7644451 ], [1.113101 , 1.102701 , 1.1013933 , ..., 0.7704713 , 0.76745415, 0.7644451 ], [1.113101 , 1.102701 , 1.1013933 , ..., 0.7704713 , 0.76745415, 0.7644451 ]]]], dtype=float32)
- thv(time, NC, sample, z)float32304.3 304.3 304.3 ... nan nan nan
- units :
- K
- long_name :
- Virtual potential temperature
array([[[[304.2598 , 304.28384, 304.3052 , ..., 316.11017, 316.15854, 316.20834], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[304.31955, 304.3449 , 304.36722, ..., 316.1197 , 316.16644, 316.21426], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[304.34396, 304.3701 , 304.39276, ..., 316.11426, 316.16272, 316.20956], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[307.95627, 308.04495, 308.12143, ..., 317.22275, 317.32962, 317.4401 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[307.92462, 308.0123 , 308.08817, ..., 317.20847, 317.32678, 317.44662], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thv_diff(time, NC, sample, zh)float32-0.01266 -0.01823 ... nan nan
- units :
- K m s-1
- long_name :
- Diffusive flux of the Virtual potential temperature
array([[[[-0.01266166, -0.01822587, -0.02060974, ..., -0.00470547, -0.00476744, -0.00481864], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.01363593, -0.0191509 , -0.02141886, ..., -0.00490513, -0.00503932, -0.00514163], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.01460437, -0.02015915, -0.02240271, ..., -0.00484676, -0.00495431, -0.00500586], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[-0.04450354, -0.05244974, -0.0518119 , ..., -0.02445727, -0.02439992, -0.02433401], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.04473199, -0.05255364, -0.05191881, ..., -0.02340343, -0.0229278 , -0.02270985], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thv_w(time, NC, sample, zh)float320.0 0.0002653 0.0005017 ... nan nan
- units :
- K m s-1
- long_name :
- Turbulent flux of the Virtual potential temperature
array([[[[ 0.0000000e+00, 2.6532100e-04, 5.0172850e-04, ..., 3.6051343e-04, 3.9098645e-04, 4.5450762e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.0000000e+00, 1.2350435e-04, 2.3591476e-04, ..., 3.8650425e-04, 3.5039542e-04, 3.2887291e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.0000000e+00, 3.9456529e-05, 7.3007592e-05, ..., 5.4332259e-04, 5.2961096e-04, 4.7254600e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 2.9908062e-04, 4.2867320e-04, 7.1788952e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0.0000000e+00, 3.2875236e-04, 6.2808598e-04, ..., 5.4240174e-04, 4.1473648e-04, 5.7072745e-04], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thv_grad(time, NC, sample, zh)float320.05913 0.002185 ... nan nan
- units :
- K m-1
- long_name :
- Gradient of the Virtual potential temperature
array([[[[ 5.9131678e-02, 2.1846897e-03, 1.7845514e-03, ..., 1.2097259e-03, 1.2444091e-03, 1.3348531e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 6.3342802e-02, 2.3052201e-03, 1.8573670e-03, ..., 1.1685865e-03, 1.1960714e-03, 1.2529133e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 6.6170953e-02, 2.3758756e-03, 1.8900806e-03, ..., 1.2112254e-03, 1.1712750e-03, 1.1606993e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... 2.6710581e-03, 2.7628576e-03, 2.9437726e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 2.4566364e-01, 7.9727611e-03, 6.3228137e-03, ..., 2.9578058e-03, 2.9957118e-03, 2.9765144e-03], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thv_2(time, NC, sample, z)float320.01709 0.01786 0.01854 ... nan nan
- units :
- K2
- long_name :
- Moment 2 of the Virtual potential temperature
array([[[[0.01709154, 0.01785871, 0.01854122, ..., 0.00159176, 0.00167007, 0.00193939], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00581397, 0.00605531, 0.00627067, ..., 0.00164499, 0.00163403, 0.00161434], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00130853, 0.00132475, 0.00133275, ..., 0.00183297, 0.00172056, 0.00158957], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[0.01127811, 0.01060609, 0.01000253, ..., 0.00995218, 0.01091951, 0.0123275 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00978331, 0.00934559, 0.00879309, ..., 0.01025839, 0.01070793, 0.01196146], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- thv_flux(time, NC, sample, zh)float32-0.01266 -0.01796 ... nan nan
- units :
- K m s-1
- long_name :
- Total flux of the Virtual potential temperature
array([[[[-0.01266166, -0.01796055, -0.02010801, ..., -0.00434496, -0.00437645, -0.00436413], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.01363593, -0.0190274 , -0.02118294, ..., -0.00451862, -0.00468892, -0.00481276], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.01460437, -0.02011969, -0.0223297 , ..., -0.00430344, -0.00442469, -0.00453331], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[-0.04450354, -0.05212678, -0.05121532, ..., -0.02415819, -0.02397125, -0.02361612], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[-0.04473199, -0.05222489, -0.05129072, ..., -0.02286103, -0.02251306, -0.02213912], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- T(time, NC, sample, z)float32298.9 298.8 298.7 ... nan nan nan
- units :
- K
- long_name :
- Absolute temperature
array([[[[298.90024, 298.8232 , 298.73257, ..., 273.807 , 273.46497, 273.12387], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[298.96994, 298.8942 , 298.80447, ..., 273.81577, 273.47202, 273.129 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[299.00107, 298.92612, 298.8367 , ..., 273.81018, 273.46817, 273.12424], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[302.44568, 302.42783, 302.38806, ..., 275.0787 , 274.7908 , 274.50586], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[302.4128 , 302.3939 , 302.3536 , ..., 275.0618 , 274.78497, 274.509 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- T_2(time, NC, sample, z)float320.02067 0.02165 0.02253 ... nan nan
- units :
- K2
- long_name :
- Moment 2 of the Absolute temperature
array([[[[0.0206748 , 0.02165378, 0.0225272 , ..., 0.001546 , 0.00159805, 0.00182364], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00703154, 0.00736588, 0.00766915, ..., 0.00158796, 0.00155439, 0.00151258], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00159006, 0.00160192, 0.00160804, ..., 0.00178752, 0.00165116, 0.00149866], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[0.01187499, 0.01121152, 0.0106227 , ..., 0.00909968, 0.00987519, 0.01104161], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.01019207, 0.00978379, 0.0092658 , ..., 0.00940949, 0.00971589, 0.01074316], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- ql(time, NC, sample, z)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- kg kg-1
- long_name :
- Liquid water
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- ql_diff(time, NC, sample, zh)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- kg kg-1 m s-1
- long_name :
- Diffusive flux of the Liquid water
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- ql_w(time, NC, sample, zh)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- kg kg-1 m s-1
- long_name :
- Turbulent flux of the Liquid water
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- ql_frac(time, NC, sample, z)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- -
- long_name :
- Liquid water fraction
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- ql_grad(time, NC, sample, zh)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- kg kg-1 m-1
- long_name :
- Gradient of the Liquid water
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- ql_cover(time, NC, sample)float320.0 0.0 0.0 0.0 ... nan 0.0 0.0 0.0
- units :
- -
- long_name :
- Liquid water cover
array([[[0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00]], [[0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00]], [[0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], ... [ nan, nan, nan], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00]], [[0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [ nan, nan, nan], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00]], [[0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [ nan, nan, nan], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00]]], dtype=float32)
- ql_path(time, NC, sample)float320.0 nan nan 0.0 ... nan 0.0 nan nan
- units :
- kg m-2
- long_name :
- Liquid water path
array([[[0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan]], [[0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan]], [[0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], ... [ nan, nan, nan], [0.00000000e+00, nan, nan]], [[0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [ nan, nan, nan], [0.00000000e+00, nan, nan]], [[0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [ nan, nan, nan], [0.00000000e+00, nan, nan]]], dtype=float32)
- ql_flux(time, NC, sample, zh)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- kg kg-1 m s-1
- long_name :
- Total flux of the Liquid water
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qi(time, NC, sample, z)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- kg kg-1
- long_name :
- Ice
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qi_frac(time, NC, sample, z)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- -
- long_name :
- Ice fraction
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qi_cover(time, NC, sample)float320.0 0.0 0.0 0.0 ... nan 0.0 0.0 0.0
- units :
- -
- long_name :
- Ice cover
array([[[ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.]], [[ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.]], [[ 0., 0., 0.], [ 0., 0., 0.], ... [nan, nan, nan], [ 0., 0., 0.]], [[ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [nan, nan, nan], [ 0., 0., 0.]], [[ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [nan, nan, nan], [ 0., 0., 0.]]], dtype=float32)
- qi_path(time, NC, sample)float320.0 nan nan 0.0 ... nan 0.0 nan nan
- units :
- kg m-2
- long_name :
- Ice path
array([[[ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan]], [[ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan]], [[ 0., nan, nan], [ 0., nan, nan], ... [nan, nan, nan], [ 0., nan, nan]], [[ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [nan, nan, nan], [ 0., nan, nan]], [[ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [ 0., nan, nan], [nan, nan, nan], [ 0., nan, nan]]], dtype=float32)
- qlqi(time, NC, sample, z)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- kg kg-1
- long_name :
- Liquid water and ice
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qlqi_frac(time, NC, sample, z)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- -
- long_name :
- Liquid water and ice fraction
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qlqi_cover(time, NC, sample)float320.0 0.0 0.0 0.0 ... nan 0.0 0.0 0.0
- units :
- -
- long_name :
- Liquid water and ice cover
array([[[0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00]], [[0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00]], [[0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], ... [ nan, nan, nan], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00]], [[0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [ nan, nan, nan], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00]], [[0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [ nan, nan, nan], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00]]], dtype=float32)
- qlqi_path(time, NC, sample)float320.0 nan nan 0.0 ... nan 0.0 nan nan
- units :
- kg m-2
- long_name :
- Liquid water and ice path
array([[[0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan]], [[0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan]], [[0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], ... [ nan, nan, nan], [0.00000000e+00, nan, nan]], [[0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [ nan, nan, nan], [0.00000000e+00, nan, nan]], [[0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [0.00000000e+00, nan, nan], [ nan, nan, nan], [0.00000000e+00, nan, nan]]], dtype=float32)
- qsat(time, NC, sample, z)float320.02161 0.02154 0.02145 ... nan nan
- units :
- kg kg-1
- long_name :
- Saturated water vapor
array([[[[0.02161005, 0.02153743, 0.02144999, ..., 0.00657082, 0.00644176, 0.00631528], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.02170012, 0.02162902, 0.02154239, ..., 0.00657497, 0.00644505, 0.00631761], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.02174058, 0.02167029, 0.02158395, ..., 0.00657241, 0.00644335, 0.00631553], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[0.02667759, 0.02668304, 0.02665732, ..., 0.00718671, 0.00707472, 0.00696567], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.02662607, 0.02662994, 0.02660333, ..., 0.00717813, 0.00707188, 0.00696737], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- qsat_path(time, NC, sample)float3262.67 nan nan ... 70.2 nan nan
- units :
- kg m-2
- long_name :
- Saturated water vapor path
array([[[62.673542 , nan, nan], [62.68858 , nan, nan], [62.602005 , nan, nan], [62.601994 , nan, nan], [62.60074 , nan, nan], [62.61755 , nan, nan], [62.57175 , nan, nan], [62.593243 , nan, nan]], [[62.90163 , nan, nan], [62.912807 , nan, nan], [62.845795 , nan, nan], [62.845768 , nan, nan], [62.83283 , nan, nan], [62.85103 , nan, nan], [62.816936 , nan, nan], [62.837982 , nan, nan]], [[63.261566 , nan, nan], [63.273342 , nan, nan], ... [ nan, nan, nan], [70.79688 , nan, nan]], [[70.70423 , nan, nan], [70.737206 , nan, nan], [70.6531 , nan, nan], [70.65298 , nan, nan], [70.62322 , nan, nan], [70.61326 , nan, nan], [ nan, nan, nan], [70.519394 , nan, nan]], [[70.382965 , nan, nan], [70.43129 , nan, nan], [70.346855 , nan, nan], [70.346794 , nan, nan], [70.32101 , nan, nan], [70.28391 , nan, nan], [ nan, nan, nan], [70.20156 , nan, nan]]], dtype=float32)
- rh(time, NC, sample, z)float320.5957 0.5962 0.5974 ... nan nan
- units :
- -
- long_name :
- Relative humidity
array([[[[0.5956568 , 0.5961567 , 0.5974106 , ..., 0.52737933, 0.53261906, 0.5380284 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.5903401 , 0.5907562 , 0.5919464 , ..., 0.5267768 , 0.5323208 , 0.5380596 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.5873893 , 0.58778363, 0.5889613 , ..., 0.5267046 , 0.5319185 , 0.5377149 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[0.49648312, 0.4960666 , 0.4962586 , ..., 0.3676584 , 0.3655691 , 0.36331007], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.49782053, 0.4974346 , 0.49764284, ..., 0.37073568, 0.36742303, 0.36417365], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- zi(time, NC, sample)float322.818e+04 2.818e+04 ... 3.054e+04
- units :
- m
- long_name :
- Boundary Layer Depth
array([[[28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ]], [[28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ]], [[28181. , 28181. , 28181. ], [28181. , 28181. , 28181. ], ... [ nan, nan, nan], [29752.334, 29752.334, 29752.334]], [[30538. , 30538. , 30538. ], [30538. , 30538. , 30538. ], [30538. , 30538. , 30538. ], [30538. , 30538. , 30538. ], [30538. , 30538. , 30538. ], [30538. , 30538. , 30538. ], [ nan, nan, nan], [30538. , 30538. , 30538. ]], [[30538. , 30538. , 30538. ], [30538. , 30538. , 30538. ], [30538. , 30538. , 30538. ], [30538. , 30538. , 30538. ], [30538. , 30538. , 30538. ], [30538. , 30538. , 30538. ], [ nan, nan, nan], [30538. , 30538. , 30538. ]]], dtype=float32)
- rr(time, NC, sample)float320.0 0.0 0.0 0.0 ... nan 0.0 0.0 0.0
- units :
- kg m-2 s-1
- long_name :
- Mean surface rain rate
array([[[0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00]], [[0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00]], [[0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], ... [ nan, nan, nan], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00]], [[0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [ nan, nan, nan], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00]], [[0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [ nan, nan, nan], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00]]], dtype=float32)
- qr_cover(time, NC, sample)float320.0 0.0 0.0 0.0 ... nan 0.0 0.0 0.0
- units :
- -
- long_name :
- Rain water specific humidity cover
array([[[0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00]], [[0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00]], [[0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], ... [ nan, nan, nan], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00]], [[0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [ nan, nan, nan], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00]], [[0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00], [ nan, nan, nan], [0.0000000e+00, 0.0000000e+00, 0.0000000e+00]]], dtype=float32)
- qr_frac(time, NC, sample, z)float320.0 0.0 0.0 0.0 ... nan nan nan nan
- units :
- -
- long_name :
- Rain water specific humidity fraction
array([[[[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], ... [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], ..., [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], [[ 0., 0., 0., ..., 0., 0., 0.], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- ustar(time, NC, sample)float320.5149 0.5149 ... 0.3984 0.3984
- units :
- m s-1
- long_name :
- Surface friction velocity
array([[[0.51489836, 0.51489836, 0.51489836], [0.50795513, 0.50795513, 0.50795513], [0.5115656 , 0.5115656 , 0.5115656 ], [0.5115656 , 0.5115656 , 0.5115656 ], [0.5100952 , 0.5100952 , 0.5100952 ], [0.51190597, 0.51190597, 0.51190597], [0.5090806 , 0.5090806 , 0.5090806 ], [0.510747 , 0.510747 , 0.510747 ]], [[0.50229007, 0.50229007, 0.50229007], [0.49997345, 0.49997345, 0.49997345], [0.5008376 , 0.5008376 , 0.5008376 ], [0.5008376 , 0.5008376 , 0.5008376 ], [0.4982412 , 0.4982412 , 0.4982412 ], [0.49858966, 0.49858966, 0.49858966], [0.49941555, 0.49941555, 0.49941555], [0.49980858, 0.49980858, 0.49980858]], [[0.49452648, 0.49452648, 0.49452648], [0.5044152 , 0.5044152 , 0.5044152 ], ... [ nan, nan, nan], [0.38511586, 0.38511586, 0.38511586]], [[0.388016 , 0.388016 , 0.388016 ], [0.37931672, 0.37931672, 0.37931672], [0.38153347, 0.38153347, 0.38153347], [0.38153347, 0.38153347, 0.38153347], [0.3879046 , 0.3879046 , 0.3879046 ], [0.37961766, 0.37961766, 0.37961766], [ nan, nan, nan], [0.3847156 , 0.3847156 , 0.3847156 ]], [[0.39959124, 0.39959124, 0.39959124], [0.3908273 , 0.3908273 , 0.3908273 ], [0.393356 , 0.393356 , 0.393356 ], [0.393356 , 0.393356 , 0.393356 ], [0.39712453, 0.39712453, 0.39712453], [0.39250827, 0.39250827, 0.39250827], [ nan, nan, nan], [0.39838925, 0.39838925, 0.39838925]]], dtype=float32)
- obuk(time, NC, sample)float32885.5 885.5 885.5 ... 109.3 109.3
- units :
- m
- long_name :
- Obukhov length
array([[[ 8.85527405e+02, 8.85527405e+02, 8.85527405e+02], [ 7.39732483e+02, 7.39732483e+02, 7.39732483e+02], [ 6.76298645e+02, 6.76298645e+02, 6.76298645e+02], [ 6.76298645e+02, 6.76298645e+02, 6.76298645e+02], [ 7.26304199e+02, 7.26304199e+02, 7.26304199e+02], [ 7.37503906e+02, 7.37503906e+02, 7.37503906e+02], [ 7.11164856e+02, 7.11164856e+02, 7.11164856e+02], [ 7.26206543e+02, 7.26206543e+02, 7.26206543e+02]], [[ 5.24071924e+03, 5.24071924e+03, 5.24071924e+03], [ 6.93332336e+02, 6.93332336e+02, 6.93332336e+02], [-1.95544275e+03, -1.95544275e+03, -1.95544275e+03], [-1.95544275e+03, -1.95544275e+03, -1.95544275e+03], [ 2.78458569e+03, 2.78458569e+03, 2.78458569e+03], [ 6.03595264e+03, 6.03595264e+03, 6.03595264e+03], [-2.41990430e+03, -2.41990430e+03, -2.41990430e+03], [ 2.23545850e+03, 2.23545850e+03, 2.23545850e+03]], [[-3.09008179e+02, -3.09008179e+02, -3.09008179e+02], [-3.31303986e+02, -3.31303986e+02, -3.31303986e+02], ... [ 9.13753281e+01, 9.13753281e+01, 9.13753281e+01]], [[ 9.85804214e+01, 9.85804214e+01, 9.85804214e+01], [ 9.36997604e+01, 9.36997604e+01, 9.36997604e+01], [ 9.42589417e+01, 9.42589417e+01, 9.42589417e+01], [ 9.42589417e+01, 9.42589417e+01, 9.42589417e+01], [ 9.80484390e+01, 9.80484390e+01, 9.80484390e+01], [ 9.25349503e+01, 9.25349503e+01, 9.25349503e+01], [ nan, nan, nan], [ 9.55584946e+01, 9.55584946e+01, 9.55584946e+01]], [[ 1.09874290e+02, 1.09874290e+02, 1.09874290e+02], [ 1.05215157e+02, 1.05215157e+02, 1.05215157e+02], [ 1.06735481e+02, 1.06735481e+02, 1.06735481e+02], [ 1.06735481e+02, 1.06735481e+02, 1.06735481e+02], [ 1.08037148e+02, 1.08037148e+02, 1.08037148e+02], [ 1.04659515e+02, 1.04659515e+02, 1.04659515e+02], [ nan, nan, nan], [ 1.09322395e+02, 1.09322395e+02, 1.09322395e+02]]], dtype=float32)
- wl(time, NC, sample)float327.758e-15 7.758e-15 ... 1.273e-13
- units :
- m
- long_name :
- Liquid water reservoir
array([[[7.75759445e-15, 7.75759445e-15, 7.75759445e-15], [3.43826767e-16, 3.43826767e-16, 3.43826767e-16], [1.47517326e-16, 1.47517326e-16, 1.47517326e-16], [1.47517326e-16, 1.47517326e-16, 1.47517326e-16], [3.40821143e-17, 3.40821143e-17, 3.40821143e-17], [7.41979438e-18, 7.41979438e-18, 7.41979438e-18], [3.40113011e-18, 3.40113011e-18, 3.40113011e-18], [1.72483877e-18, 1.72483877e-18, 1.72483877e-18]], [[5.16716483e-15, 5.16716483e-15, 5.16716483e-15], [2.28339621e-16, 2.28339621e-16, 2.28339621e-16], [9.67383425e-17, 9.67383425e-17, 9.67383425e-17], [9.67383425e-17, 9.67383425e-17, 9.67383425e-17], [2.25519528e-17, 2.25519528e-17, 2.25519528e-17], [4.90102238e-18, 4.90102238e-18, 4.90102238e-18], [2.23066168e-18, 2.23066168e-18, 2.23066168e-18], [1.14763741e-18, 1.14763741e-18, 1.14763741e-18]], [[3.25202905e-15, 3.25202905e-15, 3.25202905e-15], [1.42786872e-16, 1.42786872e-16, 1.42786872e-16], ... [ nan, nan, nan], [1.94691797e-13, 1.94691797e-13, 1.94691797e-13]], [[9.07253439e-10, 9.07253439e-10, 9.07253439e-10], [2.03742006e-11, 2.03742006e-11, 2.03742006e-11], [4.22197737e-12, 4.22197737e-12, 4.22197737e-12], [4.22197737e-12, 4.22197737e-12, 4.22197737e-12], [1.33076568e-12, 1.33076568e-12, 1.33076568e-12], [4.53986973e-13, 4.53986973e-13, 4.53986973e-13], [ nan, nan, nan], [1.57964665e-13, 1.57964665e-13, 1.57964665e-13]], [[7.25874416e-10, 7.25874416e-10, 7.25874416e-10], [1.64815158e-11, 1.64815158e-11, 1.64815158e-11], [3.42319207e-12, 3.42319207e-12, 3.42319207e-12], [3.42319207e-12, 3.42319207e-12, 3.42319207e-12], [1.06919042e-12, 1.06919042e-12, 1.06919042e-12], [3.68114827e-13, 3.68114827e-13, 3.68114827e-13], [ nan, nan, nan], [1.27252267e-13, 1.27252267e-13, 1.27252267e-13]]], dtype=float32)
- H(time, NC, sample)float32-19.0 -19.0 -19.0 ... -50.47 -50.47
- units :
- W m-2
- long_name :
- Surface sensible heat flux
array([[[-18.997353 , -18.997353 , -18.997353 ], [-20.139051 , -20.139051 , -20.139051 ], [-21.261312 , -21.261312 , -21.261312 ], [-21.261312 , -21.261312 , -21.261312 ], [-20.46846 , -20.46846 , -20.46846 ], [-20.164072 , -20.164072 , -20.164072 ], [-20.472204 , -20.472204 , -20.472204 ], [-20.499102 , -20.499102 , -20.499102 ]], [[ 6.284144 , 6.284144 , 6.284144 ], [ 5.213218 , 5.213218 , 5.213218 ], [ 3.8945694, 3.8945694, 3.8945694], [ 3.8945694, 3.8945694, 3.8945694], [ 4.702566 , 4.702566 , 4.702566 ], [ 4.8372655, 4.8372655, 4.8372655], [ 4.3127 , 4.3127 , 4.3127 ], [ 4.3312125, 4.3312125, 4.3312125]], [[ 31.912851 , 31.912851 , 31.912851 ], [ 31.704275 , 31.704275 , 31.704275 ], ... [ nan, nan, nan], [-53.647137 , -53.647137 , -53.647137 ]], [[-51.30929 , -51.30929 , -51.30929 ], [-50.652637 , -50.652637 , -50.652637 ], [-50.80778 , -50.80778 , -50.80778 ], [-50.80778 , -50.80778 , -50.80778 ], [-51.38061 , -51.38061 , -51.38061 ], [-50.927532 , -50.927532 , -50.927532 ], [ nan, nan, nan], [-51.253414 , -51.253414 , -51.253414 ]], [[-50.553238 , -50.553238 , -50.553238 ], [-49.77367 , -49.77367 , -49.77367 ], [-49.912403 , -49.912403 , -49.912403 ], [-49.912403 , -49.912403 , -49.912403 ], [-50.485126 , -50.485126 , -50.485126 ], [-50.21583 , -50.21583 , -50.21583 ], [ nan, nan, nan], [-50.468983 , -50.468983 , -50.468983 ]]], dtype=float32)
- LE(time, NC, sample)float3266.26 66.26 66.26 ... 11.2 11.2
- units :
- W m-2
- long_name :
- Surface latent heat flux
array([[[ 66.26182 , 66.26182 , 66.26182 ], [ 66.98254 , 66.98254 , 66.98254 ], [ 67.52323 , 67.52323 , 67.52323 ], [ 67.52323 , 67.52323 , 67.52323 ], [ 67.222275 , 67.222275 , 67.222275 ], [ 66.88033 , 66.88033 , 66.88033 ], [ 66.87635 , 66.87635 , 66.87635 ], [ 67.02857 , 67.02857 , 67.02857 ]], [[101.17887 , 101.17887 , 101.17887 ], [101.93517 , 101.93517 , 101.93517 ], [102.766174 , 102.766174 , 102.766174 ], [102.766174 , 102.766174 , 102.766174 ], [102.350914 , 102.350914 , 102.350914 ], [102.092125 , 102.092125 , 102.092125 ], [102.27244 , 102.27244 , 102.27244 ], [102.41309 , 102.41309 , 102.41309 ]], [[137.85616 , 137.85616 , 137.85616 ], [138.19829 , 138.19829 , 138.19829 ], ... [ nan, nan, nan], [ 15.981828 , 15.981828 , 15.981828 ]], [[ 11.780869 , 11.780869 , 11.780869 ], [ 11.6443815, 11.6443815, 11.6443815], [ 11.620026 , 11.620026 , 11.620026 ], [ 11.620026 , 11.620026 , 11.620026 ], [ 11.721504 , 11.721504 , 11.721504 ], [ 11.655372 , 11.655372 , 11.655372 ], [ nan, nan, nan], [ 11.647546 , 11.647546 , 11.647546 ]], [[ 11.330916 , 11.330916 , 11.330916 ], [ 11.178574 , 11.178574 , 11.178574 ], [ 11.154429 , 11.154429 , 11.154429 ], [ 11.154429 , 11.154429 , 11.154429 ], [ 11.259244 , 11.259244 , 11.259244 ], [ 11.21286 , 11.21286 , 11.21286 ], [ nan, nan, nan], [ 11.197764 , 11.197764 , 11.197764 ]]], dtype=float32)
- G(time, NC, sample)float32-5.177 -5.177 ... -18.46 -18.46
- units :
- W m-2
- long_name :
- Surface soil heat flux
array([[[ -5.1767116, -5.1767116, -5.1767116], [ -4.816609 , -4.816609 , -4.816609 ], [ -4.1233964, -4.1233964, -4.1233964], [ -4.1233964, -4.1233964, -4.1233964], [ -4.614084 , -4.614084 , -4.614084 ], [ -4.57466 , -4.57466 , -4.57466 ], [ -4.2012386, -4.2012386, -4.2012386], [ -4.3756943, -4.3756943, -4.3756943]], [[ 7.223437 , 7.223437 , 7.223437 ], [ 7.4831276, 7.4831276, 7.4831276], [ 8.123116 , 8.123116 , 8.123116 ], [ 8.123116 , 8.123116 , 8.123116 ], [ 7.7030005, 7.7030005, 7.7030005], [ 7.8169436, 7.8169436, 7.8169436], [ 8.222584 , 8.222584 , 8.222584 ], [ 8.025334 , 8.025334 , 8.025334 ]], [[ 20.93782 , 20.93782 , 20.93782 ], [ 20.833899 , 20.833899 , 20.833899 ], ... [ nan, nan, nan], [-15.908249 , -15.908249 , -15.908249 ]], [[-17.994987 , -17.994987 , -17.994987 ], [-18.252869 , -18.252869 , -18.252869 ], [-18.096838 , -18.096838 , -18.096838 ], [-18.096838 , -18.096838 , -18.096838 ], [-17.796556 , -17.796556 , -17.796556 ], [-17.983747 , -17.983747 , -17.983747 ], [ nan, nan, nan], [-17.770483 , -17.770483 , -17.770483 ]], [[-18.65776 , -18.65776 , -18.65776 ], [-19.000933 , -19.000933 , -19.000933 ], [-18.860939 , -18.860939 , -18.860939 ], [-18.860939 , -18.860939 , -18.860939 ], [-18.541285 , -18.541285 , -18.541285 ], [-18.627825 , -18.627825 , -18.627825 ], [ nan, nan, nan], [-18.460947 , -18.460947 , -18.460947 ]]], dtype=float32)
- S(time, NC, sample)float320.0 0.0 0.0 0.0 ... nan 0.0 0.0 0.0
- units :
- W m-2
- long_name :
- Surface storage heat flux
array([[[ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.]], [[ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.]], [[ 0., 0., 0.], [ 0., 0., 0.], ... [nan, nan, nan], [ 0., 0., 0.]], [[ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [nan, nan, nan], [ 0., 0., 0.]], [[ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [ 0., 0., 0.], [nan, nan, nan], [ 0., 0., 0.]]], dtype=float32)
- t(time, NC, sample, zs)float32289.0 293.7 298.8 ... nan nan nan
- units :
- K
- long_name :
- Soil temperature
array([[[[289.03824, 293.70065, 298.79984, 298.7689 ], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], [[289.03824, 293.70065, 298.7403 , 298.75833], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], [[289.03824, 293.70065, 298.67136, 298.694 ], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], ..., [[289.03824, 293.70065, 298.71335, 298.717 ], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], [[289.03824, 293.70065, 298.65836, 298.6733 ], [ nan, nan, nan, nan], ... [ nan, nan, nan, nan], [ nan, nan, nan, nan]], [[289.03824, 293.70065, 299.9181 , 301.92776], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], ..., [[289.03824, 293.70065, 299.91248, 301.93015], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], [[ nan, nan, nan, nan], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], [[289.03824, 293.70065, 299.8967 , 301.90506], [ nan, nan, nan, nan], [ nan, nan, nan, nan]]]], dtype=float32)
- theta(time, NC, sample, zs)float320.2032 0.2371 0.2555 ... nan nan
- units :
- -
- long_name :
- Soil volumetric water content
array([[[[0.20322801, 0.2371441 , 0.25546244, 0.22602469], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], [[0.20322801, 0.2371407 , 0.25543445, 0.22603874], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], [[0.20322801, 0.23715757, 0.25552115, 0.22601976], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], ..., [[0.20322801, 0.23715223, 0.25550047, 0.22603868], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], [[0.20322801, 0.23713781, 0.25550035, 0.22603978], [ nan, nan, nan, nan], ... [ nan, nan, nan, nan]], [[0.20322801, 0.23485728, 0.24125348, 0.21718068], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], ..., [[0.20322801, 0.23485337, 0.2412508 , 0.21725322], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], [[ nan, nan, nan, nan], [ nan, nan, nan, nan], [ nan, nan, nan, nan]], [[0.20322801, 0.23480846, 0.24141274, 0.21726756], [ nan, nan, nan, nan], [ nan, nan, nan, nan]]]], dtype=float32)
- lw_flux_up(time, NC, sample, zh)float32445.0 446.4 446.4 ... nan nan nan
- units :
- W m-2
- long_name :
- Longwave upwelling flux
array([[[[445.0265 , 446.35837, 446.4403 , ..., 364.7571 , 363.8837 , 363.0109 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[445.17557, 446.59384, 446.6914 , ..., 364.77982, 363.90405, 363.0282 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[445.21436, 446.68265, 446.7891 , ..., 364.81882, 363.94394, 363.06577], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[456.62854, 461.4978 , 462.21036, ..., 373.84634, 373.10107, 372.36563], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[456.57205, 461.39066, 462.09384, ..., 373.7816 , 373.04922, 372.32578], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- lw_flux_dn(time, NC, sample, zh)float32380.2 380.1 379.4 ... nan nan nan
- units :
- W m-2
- long_name :
- Longwave downwelling flux
array([[[[380.1896 , 380.09146, 379.4249 , ..., 203.56685, 201.97766, 200.39409], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[380.2678 , 380.2161 , 379.55106, ..., 203.61003, 202.01842, 200.43097], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[380.42636, 380.40213, 379.73813, ..., 203.57153, 201.97871, 200.38753], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[399.02063, 400.91116, 400.36948, ..., 199.44334, 197.91737, 196.40819], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[398.86282, 400.72476, 400.1813 , ..., 199.50005, 197.97804, 196.46545], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- lw_flux_up_clear(time, NC, sample, zh)float32445.0 446.4 446.4 ... nan nan nan
- units :
- W m-2
- long_name :
- Clear-sky longwave upwelling flux
array([[[[445.0265 , 446.35837, 446.4403 , ..., 364.7571 , 363.8837 , 363.0109 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[445.17557, 446.59384, 446.6914 , ..., 364.77982, 363.90405, 363.0282 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[445.21436, 446.68265, 446.7891 , ..., 364.81882, 363.94394, 363.06577], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[456.62854, 461.4978 , 462.21036, ..., 373.84634, 373.10107, 372.36563], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[456.57205, 461.39066, 462.09384, ..., 373.7816 , 373.04922, 372.32578], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- lw_flux_dn_clear(time, NC, sample, zh)float32380.2 380.1 379.4 ... nan nan nan
- units :
- W m-2
- long_name :
- Clear-sky longwave downwelling flux
array([[[[380.1896 , 380.09146, 379.4249 , ..., 203.56685, 201.97766, 200.39409], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[380.2678 , 380.2161 , 379.55106, ..., 203.61003, 202.01842, 200.43097], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[380.42636, 380.40213, 379.73813, ..., 203.57153, 201.97871, 200.38753], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[399.02063, 400.91116, 400.36948, ..., 199.44334, 197.91737, 196.40819], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[398.86282, 400.72476, 400.1813 , ..., 199.50005, 197.97804, 196.46545], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- sw_flux_up(time, NC, sample, zh)float3222.85 22.88 22.9 ... nan nan nan
- units :
- W m-2
- long_name :
- Shortwave upwelling flux
array([[[[22.854927 , 22.878485 , 22.896006 , ..., 29.351837 , 29.419485 , 29.487097 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[22.857103 , 22.880682 , 22.898224 , ..., 29.354013 , 29.42166 , 29.489271 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[22.854868 , 22.878471 , 22.896027 , ..., 29.352058 , 29.41971 , 29.487326 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- sw_flux_dn(time, NC, sample, zh)float32130.0 130.1 130.2 ... nan nan nan
- units :
- W m-2
- long_name :
- Shortwave downwelling flux
array([[[[129.98727 , 130.12338 , 130.22444 , ..., 164.56998 , 164.88786 , 165.20677 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[129.99965 , 130.13536 , 130.23607 , ..., 164.55981 , 164.87746 , 165.19621 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[129.98694 , 130.12222 , 130.22266 , ..., 164.56802 , 164.88564 , 165.20433 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- sw_flux_dn_dir(time, NC, sample, zh)float32103.2 103.4 103.5 ... nan nan nan
- units :
- W m-2
- long_name :
- Shortwave direct downwelling flux
array([[[[103.22776 , 103.38087 , 103.49451 , ..., 142.94357 , 143.32072 , 143.6989 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[103.239845 , 103.392555 , 103.50587 , ..., 142.93344 , 143.31035 , 143.6884 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[103.227325 , 103.379616 , 103.49264 , ..., 142.94176 , 143.3186 , 143.69661 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- sw_flux_up_clear(time, NC, sample, zh)float3222.85 22.88 22.9 ... nan nan nan
- units :
- W m-2
- long_name :
- Clear-sky shortwave upwelling flux
array([[[[22.854927 , 22.878485 , 22.896006 , ..., 29.351837 , 29.419485 , 29.487097 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[22.857103 , 22.880682 , 22.898224 , ..., 29.354013 , 29.42166 , 29.489271 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[22.854868 , 22.878471 , 22.896027 , ..., 29.352058 , 29.41971 , 29.487326 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- sw_flux_dn_clear(time, NC, sample, zh)float32130.0 130.1 130.2 ... nan nan nan
- units :
- W m-2
- long_name :
- Clear-sky shortwave downwelling flux
array([[[[129.98727 , 130.12338 , 130.22444 , ..., 164.56998 , 164.88786 , 165.20677 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[129.99965 , 130.13536 , 130.23607 , ..., 164.55981 , 164.87746 , 165.19621 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[129.98694 , 130.12222 , 130.22266 , ..., 164.56802 , 164.88564 , 165.20433 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- sw_flux_dn_dir_clear(time, NC, sample, zh)float32103.2 103.4 103.5 ... nan nan nan
- units :
- W m-2
- long_name :
- Clear-sky shortwave direct downwelling flux
array([[[[103.22776 , 103.38087 , 103.49451 , ..., 142.94357 , 143.32072 , 143.6989 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[103.239845 , 103.392555 , 103.50587 , ..., 142.93344 , 143.31035 , 143.6884 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[103.227325 , 103.379616 , 103.49264 , ..., 142.94176 , 143.3186 , 143.69661 ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], ... [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ 0. , 0. , 0. , ..., 0. , 0. , 0. ], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]], dtype=float32)
- sw_flux_up_ref(time, NC, sample, lev)float3223.2 23.88 24.69 ... 0.1828 0.183
- units :
- W m-2
- long_name :
- Shortwave upwelling flux of reference column
array([[[[2.3201559e+01, 2.3880873e+01, 2.4692039e+01, ..., 4.8085110e+01, 4.8085346e+01, 4.8085571e+01], [2.3201559e+01, 2.3880873e+01, 2.4692039e+01, ..., 4.8085110e+01, 4.8085346e+01, 4.8085571e+01], [2.3201559e+01, 2.3880873e+01, 2.4692039e+01, ..., 4.8085110e+01, 4.8085346e+01, 4.8085571e+01]], [[2.3201559e+01, 2.3880873e+01, 2.4692039e+01, ..., 4.8085110e+01, 4.8085346e+01, 4.8085571e+01], [2.3201559e+01, 2.3880873e+01, 2.4692039e+01, ..., 4.8085110e+01, 4.8085346e+01, 4.8085571e+01], [2.3201559e+01, 2.3880873e+01, 2.4692039e+01, ..., 4.8085110e+01, 4.8085346e+01, 4.8085571e+01]], [[2.3201559e+01, 2.3880873e+01, 2.4692039e+01, ..., 4.8085110e+01, 4.8085346e+01, 4.8085571e+01], [2.3201559e+01, 2.3880873e+01, 2.4692039e+01, ..., 4.8085110e+01, 4.8085346e+01, 4.8085571e+01], [2.3201559e+01, 2.3880873e+01, 2.4692039e+01, ..., 4.8085110e+01, 4.8085346e+01, 4.8085571e+01]], ... [[2.2036446e-02, 2.2283874e-02, 2.2595389e-02, ..., 1.8251310e-01, 1.8275519e-01, 1.8299709e-01], [2.2036446e-02, 2.2283874e-02, 2.2595389e-02, ..., 1.8251310e-01, 1.8275519e-01, 1.8299709e-01], [2.2036446e-02, 2.2283874e-02, 2.2595389e-02, ..., 1.8251310e-01, 1.8275519e-01, 1.8299709e-01]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[2.2036446e-02, 2.2283874e-02, 2.2595389e-02, ..., 1.8251310e-01, 1.8275519e-01, 1.8299709e-01], [2.2036446e-02, 2.2283874e-02, 2.2595389e-02, ..., 1.8251310e-01, 1.8275519e-01, 1.8299709e-01], [2.2036446e-02, 2.2283874e-02, 2.2595389e-02, ..., 1.8251310e-01, 1.8275519e-01, 1.8299709e-01]]]], dtype=float32)
- sw_flux_dn_ref(time, NC, sample, lev)float32132.0 135.4 139.5 ... 0.6187 0.6207
- units :
- W m-2
- long_name :
- Shortwave downwelling flux of reference column
array([[[[1.3195872e+02, 1.3537129e+02, 1.3946521e+02, ..., 2.3650829e+02, 2.3651221e+02, 2.3651843e+02], [1.3195872e+02, 1.3537129e+02, 1.3946521e+02, ..., 2.3650829e+02, 2.3651221e+02, 2.3651843e+02], [1.3195872e+02, 1.3537129e+02, 1.3946521e+02, ..., 2.3650829e+02, 2.3651221e+02, 2.3651843e+02]], [[1.3195872e+02, 1.3537129e+02, 1.3946521e+02, ..., 2.3650829e+02, 2.3651221e+02, 2.3651843e+02], [1.3195872e+02, 1.3537129e+02, 1.3946521e+02, ..., 2.3650829e+02, 2.3651221e+02, 2.3651843e+02], [1.3195872e+02, 1.3537129e+02, 1.3946521e+02, ..., 2.3650829e+02, 2.3651221e+02, 2.3651843e+02]], [[1.3195872e+02, 1.3537129e+02, 1.3946521e+02, ..., 2.3650829e+02, 2.3651221e+02, 2.3651843e+02], [1.3195872e+02, 1.3537129e+02, 1.3946521e+02, ..., 2.3650829e+02, 2.3651221e+02, 2.3651843e+02], [1.3195872e+02, 1.3537129e+02, 1.3946521e+02, ..., 2.3650829e+02, 2.3651221e+02, 2.3651843e+02]], ... [[1.2533219e-01, 1.2690376e-01, 1.2873481e-01, ..., 6.1719519e-01, 6.1869735e-01, 6.2071377e-01], [1.2533219e-01, 1.2690376e-01, 1.2873481e-01, ..., 6.1719519e-01, 6.1869735e-01, 6.2071377e-01], [1.2533219e-01, 1.2690376e-01, 1.2873481e-01, ..., 6.1719519e-01, 6.1869735e-01, 6.2071377e-01]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[1.2533219e-01, 1.2690376e-01, 1.2873481e-01, ..., 6.1719519e-01, 6.1869735e-01, 6.2071377e-01], [1.2533219e-01, 1.2690376e-01, 1.2873481e-01, ..., 6.1719519e-01, 6.1869735e-01, 6.2071377e-01], [1.2533219e-01, 1.2690376e-01, 1.2873481e-01, ..., 6.1719519e-01, 6.1869735e-01, 6.2071377e-01]]]], dtype=float32)
- sw_flux_dn_dir_ref(time, NC, sample, lev)float32105.2 109.1 113.8 ... 0.6185 0.6207
- units :
- W m-2
- long_name :
- Shortwave direct downwelling flux of reference column
array([[[[1.05154472e+02, 1.09057487e+02, 1.13751366e+02, ..., 2.36507736e+02, 2.36511948e+02, 2.36518433e+02], [1.05154472e+02, 1.09057487e+02, 1.13751366e+02, ..., 2.36507736e+02, 2.36511948e+02, 2.36518433e+02], [1.05154472e+02, 1.09057487e+02, 1.13751366e+02, ..., 2.36507736e+02, 2.36511948e+02, 2.36518433e+02]], [[1.05154472e+02, 1.09057487e+02, 1.13751366e+02, ..., 2.36507736e+02, 2.36511948e+02, 2.36518433e+02], [1.05154472e+02, 1.09057487e+02, 1.13751366e+02, ..., 2.36507736e+02, 2.36511948e+02, 2.36518433e+02], [1.05154472e+02, 1.09057487e+02, 1.13751366e+02, ..., 2.36507736e+02, 2.36511948e+02, 2.36518433e+02]], [[1.05154472e+02, 1.09057487e+02, 1.13751366e+02, ..., 2.36507736e+02, 2.36511948e+02, 2.36518433e+02], [1.05154472e+02, 1.09057487e+02, 1.13751366e+02, ..., 2.36507736e+02, 2.36511948e+02, 2.36518433e+02], [1.05154472e+02, 1.09057487e+02, 1.13751366e+02, ..., 2.36507736e+02, 2.36511948e+02, 2.36518433e+02]], ... 6.16711199e-01, 6.18455350e-01, 6.20713770e-01], [3.12444676e-21, 1.64182313e-18, 1.10723977e-15, ..., 6.16711199e-01, 6.18455350e-01, 6.20713770e-01], [3.12444676e-21, 1.64182313e-18, 1.10723977e-15, ..., 6.16711199e-01, 6.18455350e-01, 6.20713770e-01]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[3.12444676e-21, 1.64182313e-18, 1.10723977e-15, ..., 6.16711199e-01, 6.18455350e-01, 6.20713770e-01], [3.12444676e-21, 1.64182313e-18, 1.10723977e-15, ..., 6.16711199e-01, 6.18455350e-01, 6.20713770e-01], [3.12444676e-21, 1.64182313e-18, 1.10723977e-15, ..., 6.16711199e-01, 6.18455350e-01, 6.20713770e-01]]]], dtype=float32)
- lw_flux_up_ref(time, NC, sample, lev)float32476.5 465.4 447.4 ... 282.1 282.1
- units :
- W m-2
- long_name :
- Longwave upwelling flux of reference column
array([[[[476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072]], [[476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072]], [[476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072]], ... [[476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072]], [[476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072]], [[476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072], [476.4841 , 465.44806, 447.4295 , ..., 282.0554 , 282.05295, 282.05072]]]], dtype=float32)
- lw_flux_dn_ref(time, NC, sample, lev)float32386.1 359.6 329.6 ... 0.0216 0.0
- units :
- W m-2
- long_name :
- Longwave downwelling flux of reference column
array([[[[3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00]], [[3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00]], [[3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00]], ... [[3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00]], [[3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00]], [[3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00], [3.8605243e+02, 3.5958707e+02, 3.2958765e+02, ..., 2.9348550e-02, 2.1600969e-02, 0.0000000e+00]]]], dtype=float32)
- sza(time, NC, sample)float321.39 1.39 1.39 ... 1.743 1.743
- units :
- rad
- long_name :
- solar zenith angle
array([[[1.3903567 , 1.3903567 , 1.3903567 ], [1.3903567 , 1.3903567 , 1.3903567 ], [1.3903567 , 1.3903567 , 1.3903567 ], [1.3903567 , 1.3903567 , 1.3903567 ], [1.3903567 , 1.3903567 , 1.3903567 ], [1.3903567 , 1.3903567 , 1.3903567 ], [1.3903567 , 1.3903567 , 1.3903567 ], [1.3903567 , 1.3903567 , 1.3903567 ]], [[1.2909904 , 1.2909904 , 1.2909904 ], [1.2909904 , 1.2909904 , 1.2909904 ], [1.2909904 , 1.2909904 , 1.2909904 ], [1.2909904 , 1.2909904 , 1.2909904 ], [1.2909904 , 1.2909904 , 1.2909904 ], [1.2909904 , 1.2909904 , 1.2909904 ], [1.2909904 , 1.2909904 , 1.2909904 ], [1.2909904 , 1.2909904 , 1.2909904 ]], [[1.1894778 , 1.1894778 , 1.1894778 ], [1.1894778 , 1.1894778 , 1.1894778 ], ... [ nan, nan, nan], [1.5686334 , 1.5686334 , 1.5686334 ]], [[1.6583372 , 1.6583372 , 1.6583372 ], [1.6583372 , 1.6583372 , 1.6583372 ], [1.6583372 , 1.6583372 , 1.6583372 ], [1.6583372 , 1.6583372 , 1.6583372 ], [1.6583372 , 1.6583372 , 1.6583372 ], [1.6583372 , 1.6583372 , 1.6583372 ], [ nan, nan, nan], [1.6583372 , 1.6583372 , 1.6583372 ]], [[1.7431656 , 1.7431656 , 1.7431656 ], [1.7431656 , 1.7431656 , 1.7431656 ], [1.7431656 , 1.7431656 , 1.7431656 ], [1.7431656 , 1.7431656 , 1.7431656 ], [1.7431656 , 1.7431656 , 1.7431656 ], [1.7431656 , 1.7431656 , 1.7431656 ], [ nan, nan, nan], [1.7431656 , 1.7431656 , 1.7431656 ]]], dtype=float32)
- sw_flux_dn_toa(time, NC, sample)float32236.5 236.5 236.5 ... 0.6207 0.6207
- units :
- W m-2
- long_name :
- shortwave downwelling flux at toa
array([[[2.36518433e+02, 2.36518433e+02, 2.36518433e+02], [2.36518433e+02, 2.36518433e+02, 2.36518433e+02], [2.36518433e+02, 2.36518433e+02, 2.36518433e+02], [2.36518433e+02, 2.36518433e+02, 2.36518433e+02], [2.36518433e+02, 2.36518433e+02, 2.36518433e+02], [2.36518433e+02, 2.36518433e+02, 2.36518433e+02], [2.36518433e+02, 2.36518433e+02, 2.36518433e+02], [2.36518433e+02, 2.36518433e+02, 2.36518433e+02]], [[3.63963165e+02, 3.63963165e+02, 3.63963165e+02], [3.63963165e+02, 3.63963165e+02, 3.63963165e+02], [3.63963165e+02, 3.63963165e+02, 3.63963165e+02], [3.63963165e+02, 3.63963165e+02, 3.63963165e+02], [3.63963165e+02, 3.63963165e+02, 3.63963165e+02], [3.63963165e+02, 3.63963165e+02, 3.63963165e+02], [3.63963165e+02, 3.63963165e+02, 3.63963165e+02], [3.63963165e+02, 3.63963165e+02, 3.63963165e+02]], [[4.90439331e+02, 4.90439331e+02, 4.90439331e+02], [4.90439331e+02, 4.90439331e+02, 4.90439331e+02], ... [ nan, nan, nan], [1.68698406e+01, 1.68698406e+01, 1.68698406e+01]], [[6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [ nan, nan, nan], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01]], [[6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01], [ nan, nan, nan], [6.20713770e-01, 6.20713770e-01, 6.20713770e-01]]], dtype=float32)
- zPandasIndex
PandasIndex(Float64Index([ 10.0, 21.0, 33.0, 46.0, 60.0, 75.0, 92.0, 111.0, 132.0, 155.0, ... 3620.0, 3660.0, 3700.0, 3740.0, 3780.0, 3820.0, 3860.0, 3900.0, 3940.0, 3980.0], dtype='float64', name='z', length=107))
- zhPandasIndex
PandasIndex(Float64Index([ 0.0, 15.5, 27.0, 39.5, 53.0, 67.5, 83.5, 101.5, 121.5, 143.5, ... 3640.0, 3680.0, 3720.0, 3760.0, 3800.0, 3840.0, 3880.0, 3920.0, 3960.0, 4000.0], dtype='float64', name='zh', length=108))
- zsPandasIndex
PandasIndex(Float64Index([ -1.8899999856948853, -0.7200000286102295, -0.20999999344348907, -0.07000000029802322], dtype='float64', name='zs'))
- zshPandasIndex
PandasIndex(Float64Index([ -2.619999885559082, -1.1600000858306885, -0.2799999713897705, -0.14000000059604645, 0.0], dtype='float64', name='zsh'))
- samplePandasIndex
PandasIndex(Index(['default', 'ql', 'qlcore'], dtype='object', name='sample'))
- NCPandasIndex
PandasIndex(Int64Index([10, 50, 80, 100, 200, 400, 600, 800], dtype='int64', name='NC'))
- timePandasIndex
PandasIndex(DatetimeIndex(['2016-06-11 12:00:00', '2016-06-11 12:30:00', '2016-06-11 13:00:00', '2016-06-11 13:30:00', '2016-06-11 14:00:00', '2016-06-11 14:30:00', '2016-06-11 15:00:00', '2016-06-11 15:30:00', '2016-06-11 16:00:00', '2016-06-11 16:30:00', '2016-06-11 17:00:00', '2016-06-11 17:30:00', '2016-06-11 18:00:00', '2016-06-11 18:30:00', '2016-06-11 19:00:00', '2016-06-11 19:30:00', '2016-06-11 20:00:00', '2016-06-11 20:30:00', '2016-06-11 21:00:00', '2016-06-11 21:30:00', '2016-06-11 22:00:00', '2016-06-11 22:30:00', '2016-06-11 23:00:00', '2016-06-11 23:30:00', '2016-06-12 00:00:00', '2016-06-12 00:30:00', '2016-06-12 01:00:00', '2016-06-12 01:30:00', '2016-06-12 02:00:00', '2016-06-12 02:30:00'], dtype='datetime64[ns]', name='time', freq='30T'))
prof.sel(sample='default', NC=100, time="2016-06-11T22:00")[['qt_w', 'qt_diff']]
<xarray.Dataset> Dimensions: (zh: 108) Coordinates: * zh (zh) float32 0.0 15.5 27.0 39.5 ... 3.92e+03 3.96e+03 4e+03 sample <U7 'default' NC int64 100 time datetime64[ns] 2016-06-11T22:00:00 Data variables: qt_w (zh) float32 0.0 1.063e-05 1.896e-05 ... -8.208e-07 -7.689e-07 qt_diff (zh) float32 0.0001169 0.0001104 0.0001019 ... 1.185e-05 1.072e-05
xarray.Dataset
- zh: 108
- zh(zh)float320.0 15.5 27.0 ... 3.96e+03 4e+03
- units :
- m
- long_name :
- Half level height
array([ 0. , 15.5, 27. , 39.5, 53. , 67.5, 83.5, 101.5, 121.5, 143.5, 167.5, 193.5, 222. , 252. , 283.5, 320. , 360. , 400. , 440. , 480. , 520. , 560. , 600. , 640. , 680. , 720. , 760. , 800. , 840. , 880. , 920. , 960. , 1000. , 1040. , 1080. , 1120. , 1160. , 1200. , 1240. , 1280. , 1320. , 1360. , 1400. , 1440. , 1480. , 1520. , 1560. , 1600. , 1640. , 1680. , 1720. , 1760. , 1800. , 1840. , 1880. , 1920. , 1960. , 2000. , 2040. , 2080. , 2120. , 2160. , 2200. , 2240. , 2280. , 2320. , 2360. , 2400. , 2440. , 2480. , 2520. , 2560. , 2600. , 2640. , 2680. , 2720. , 2760. , 2800. , 2840. , 2880. , 2920. , 2960. , 3000. , 3040. , 3080. , 3120. , 3160. , 3200. , 3240. , 3280. , 3320. , 3360. , 3400. , 3440. , 3480. , 3520. , 3560. , 3600. , 3640. , 3680. , 3720. , 3760. , 3800. , 3840. , 3880. , 3920. , 3960. , 4000. ], dtype=float32)
- sample()<U7'default'
array('default', dtype='<U7')
- NC()int64100
array(100)
- time()datetime64[ns]2016-06-11T22:00:00
array('2016-06-11T22:00:00.000000000', dtype='datetime64[ns]')
- qt_w(zh)float320.0 1.063e-05 ... -7.689e-07
- units :
- kg kg-1 m s-1
- long_name :
- Turbulent flux of the Total water mixing ratio
array([ 0.00000000e+00, 1.06336402e-05, 1.89573275e-05, 2.93002904e-05, 4.07600928e-05, 5.29791832e-05, 6.55770200e-05, 7.75086883e-05, 8.77126222e-05, 9.56460135e-05, 1.01437450e-04, 1.05531282e-04, 1.08513072e-04, 1.10333960e-04, 1.11617606e-04, 1.12837668e-04, 1.13830145e-04, 1.14665345e-04, 1.15116738e-04, 1.15244729e-04, 1.15237402e-04, 1.15218230e-04, 1.15220348e-04, 1.15252529e-04, 1.15312985e-04, 1.15272742e-04, 1.14968221e-04, 1.14437229e-04, 1.13874004e-04, 1.13415394e-04, 1.13116694e-04, 1.12926449e-04, 1.12732778e-04, 1.12415721e-04, 1.11990630e-04, 1.11539615e-04, 1.10972825e-04, 1.10261812e-04, 1.09470486e-04, 1.08743807e-04, 1.08207932e-04, 1.07908410e-04, 1.07838503e-04, 1.07848253e-04, 1.07656728e-04, 1.06881351e-04, 1.05356034e-04, 1.03296981e-04, 1.00969475e-04, 9.85318038e-05, 9.57253578e-05, 9.24270062e-05, 8.85496192e-05, 8.40599168e-05, 7.97730099e-05, 7.62462514e-05, 7.38520903e-05, 7.20979078e-05, 6.94374539e-05, 6.56484262e-05, 6.17914484e-05, 5.75082086e-05, 5.28662385e-05, 4.87897814e-05, 4.54656802e-05, 4.12300251e-05, 3.63649160e-05, 3.26971822e-05, 3.02371991e-05, 2.86072827e-05, 2.65054841e-05, 2.31614304e-05, 1.98892067e-05, 1.63594723e-05, 1.22447900e-05, 8.00818361e-06, 4.48398532e-06, 1.93172264e-06, 6.36483321e-07, 3.88347729e-07, 1.43340048e-06, 1.79178085e-06, 1.92372295e-06, 2.48713718e-06, 2.96843814e-06, 2.62561571e-06, 2.39024234e-06, 2.78291168e-06, 3.10404994e-06, 2.68359713e-06, 2.29818215e-06, 2.31993977e-06, 2.30293472e-06, 2.21121923e-06, 2.31861850e-06, 2.28464182e-06, 1.90336016e-06, 1.32962816e-06, 1.00990701e-06, 8.67277322e-07, 7.11611222e-07, 5.89370245e-07, 3.35678806e-07, -2.25264998e-07, -6.89159208e-07, -7.86566773e-07, -8.20826415e-07, -7.68946677e-07], dtype=float32)
- qt_diff(zh)float320.0001169 0.0001104 ... 1.072e-05
- units :
- kg kg-1 m s-1
- long_name :
- Diffusive flux of the Total water mixing ratio
array([1.16919524e-04, 1.10382651e-04, 1.01852107e-04, 9.12145697e-05, 7.93899017e-05, 6.67485219e-05, 5.36923762e-05, 4.13004454e-05, 3.06516195e-05, 2.23285624e-05, 1.62354845e-05, 1.19234328e-05, 8.77922230e-06, 6.86213343e-06, 5.54027974e-06, 4.37171138e-06, 3.55010002e-06, 2.86321369e-06, 2.35697439e-06, 1.98640032e-06, 1.72451564e-06, 1.54468319e-06, 1.42305998e-06, 1.32999310e-06, 1.27586543e-06, 1.25291888e-06, 1.24419250e-06, 1.24622693e-06, 1.24102246e-06, 1.20426000e-06, 1.14766135e-06, 1.10328745e-06, 1.11352188e-06, 1.17647153e-06, 1.22647668e-06, 1.26431371e-06, 1.30449791e-06, 1.35208711e-06, 1.39541783e-06, 1.38653513e-06, 1.36430333e-06, 1.37508277e-06, 1.44311127e-06, 1.54529550e-06, 1.66529696e-06, 1.84677026e-06, 2.02386468e-06, 2.11752945e-06, 2.04431944e-06, 1.97025201e-06, 1.97553595e-06, 2.03326590e-06, 2.31632998e-06, 2.64319806e-06, 3.02139688e-06, 3.72016416e-06, 4.55033796e-06, 5.03488673e-06, 5.03598176e-06, 5.00476699e-06, 4.62926209e-06, 4.46577542e-06, 4.07101606e-06, 3.84759733e-06, 4.05422452e-06, 4.29214651e-06, 4.86747740e-06, 5.95568645e-06, 7.51209382e-06, 8.85653299e-06, 1.11893705e-05, 1.40262855e-05, 1.66818227e-05, 1.98941561e-05, 2.34154231e-05, 2.75152215e-05, 3.12918928e-05, 3.43053871e-05, 3.66522108e-05, 3.79863013e-05, 3.86908432e-05, 4.02458973e-05, 4.18682175e-05, 4.28836320e-05, 4.31522531e-05, 4.31957706e-05, 4.26979095e-05, 4.13078269e-05, 3.94162780e-05, 3.81177670e-05, 3.66438617e-05, 3.48653302e-05, 3.28484275e-05, 3.04962250e-05, 2.80645472e-05, 2.58403325e-05, 2.39039418e-05, 2.22100662e-05, 2.07647536e-05, 1.94432723e-05, 1.82910790e-05, 1.72045002e-05, 1.62525012e-05, 1.53473356e-05, 1.43196557e-05, 1.30856351e-05, 1.18525968e-05, 1.07170563e-05], dtype=float32)
- zhPandasIndex
PandasIndex(Float64Index([ 0.0, 15.5, 27.0, 39.5, 53.0, 67.5, 83.5, 101.5, 121.5, 143.5, ... 3640.0, 3680.0, 3720.0, 3760.0, 3800.0, 3840.0, 3880.0, 3920.0, 3960.0, 4000.0], dtype='float64', name='zh', length=108))
prof.sel(sample='default', NC=100, time="2016-06-11T22:00").qt_w.plot(y='zh')
prof.sel(sample='default', NC=100, time="2016-06-11T22:00").qt_diff.plot(y='zh')
prof.sel(sample='default', NC=100, time="2016-06-11T22:00").qt_flux.plot(y='zh')
[<matplotlib.lines.Line2D at 0x7f7604c2b3a0>]
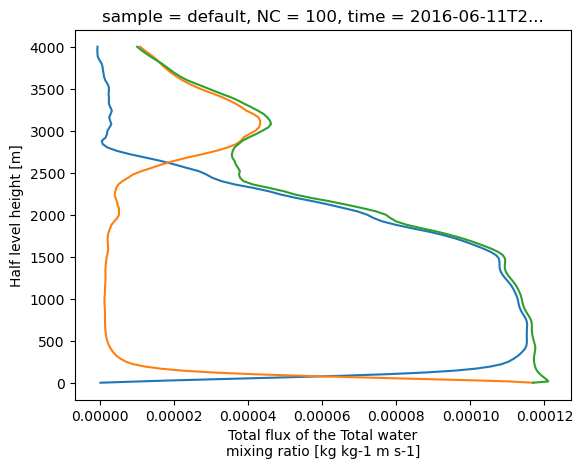
prof.sel(sample='default').ql_cover.plot(x='time', hue='NC')
[<matplotlib.lines.Line2D at 0x7f76046334c0>,
<matplotlib.lines.Line2D at 0x7f7604662ef0>,
<matplotlib.lines.Line2D at 0x7f7604662da0>,
<matplotlib.lines.Line2D at 0x7f7604662ce0>,
<matplotlib.lines.Line2D at 0x7f7604662fb0>,
<matplotlib.lines.Line2D at 0x7f76046630d0>,
<matplotlib.lines.Line2D at 0x7f76046631f0>,
<matplotlib.lines.Line2D at 0x7f7604663310>]
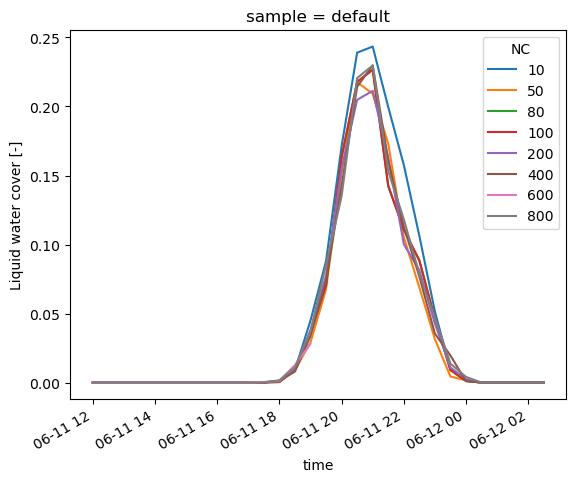
plt.figure()
prof.sel(sample='default', time="2016-06-11T22:00").qt_flux.plot(y='zh', hue='NC')
plt.figure()
prof.sel(sample='default', time="2016-06-11T22:00").thl_flux.plot(y='zh', hue='NC')
[<matplotlib.lines.Line2D at 0x7f7fc04b8e50>,
<matplotlib.lines.Line2D at 0x7f7fc04b8df0>,
<matplotlib.lines.Line2D at 0x7f7fc04b9000>,
<matplotlib.lines.Line2D at 0x7f7fc04b9120>,
<matplotlib.lines.Line2D at 0x7f7fc04b9240>,
<matplotlib.lines.Line2D at 0x7f7fc04b9360>,
<matplotlib.lines.Line2D at 0x7f7fc04b9480>]
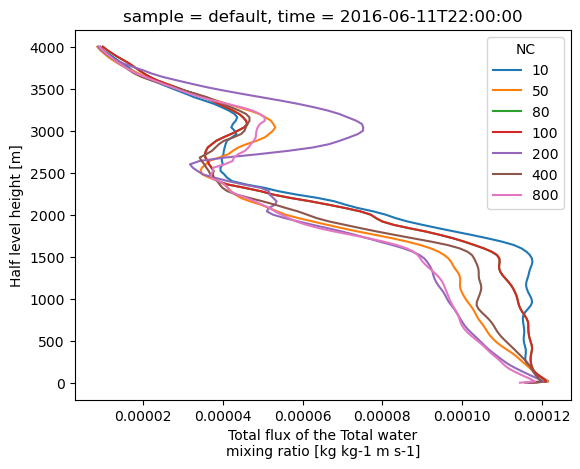
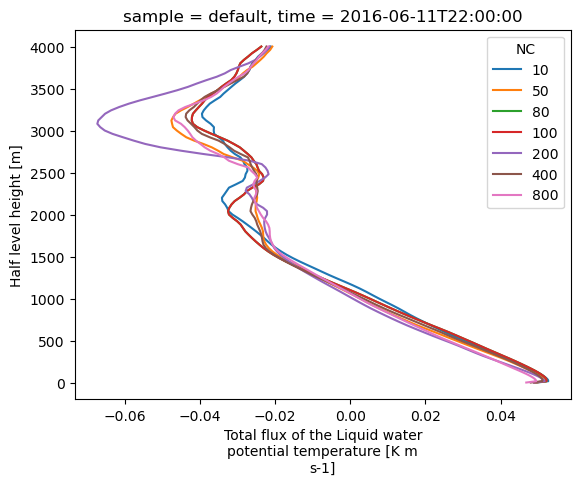